
EMencha_Algorithms_HW4.2
Fork of blink_kl46z_button_LCD by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "SLCD.h" 00003 00004 #define LEDON false 00005 #define LEDOFF true 00006 #define NUMBUTS 2 00007 #define LBUT PTC12 // port addresses for buttons 00008 #define RBUT PTC3 00009 #define BLINKTIME 0.5 // in seconds 00010 #define BUTTONTIME 0.2 // 00011 #define LCDCHARLEN 20 00012 #define NUMMESS 2 00013 #define LRED "REDBUTTONPRESSED" 00014 #define LGREEN "GREENBUTTONPRESSED" 00015 #define PRED "RED\r\n" 00016 #define PGREEN "GREEN\r\n" 00017 #define PROGNAME "blink_kl46z_buttton LCD v2\r\n" 00018 00019 // slightly more interesting blinky 140814 sc 00020 SLCD slcd; //define LCD display 00021 00022 // Timer to elliminate wait() function 00023 Timer LEDTimer; // for blinking LEDs 00024 Timer ButtonTimer; // for reading button states 00025 00026 bool ledState = LEDON; 00027 00028 DigitalIn buttons[NUMBUTS] = {RBUT, LBUT}; 00029 DigitalOut LEDs[NUMBUTS] = {LED_GREEN, LED_RED}; 00030 Serial pc(USBTX, USBRX);// set up USB as communicationis to Host PC via USB connectons 00031 00032 void allLEDsOff(){ 00033 int i; 00034 for (i=0; i<NUMBUTS; i++){ 00035 LEDs[i] = LEDOFF; 00036 } 00037 } 00038 00039 void LCDMess(char *lMess){ 00040 slcd.Home(); 00041 slcd.clear(); 00042 slcd.printf(lMess); 00043 } 00044 00045 void initialize_global_vars(){ 00046 pc.printf(PROGNAME); 00047 // set up DAQ timers 00048 ButtonTimer.start(); 00049 ButtonTimer.reset(); 00050 LEDTimer.start(); 00051 LEDTimer.reset(); 00052 allLEDsOff(); 00053 } 00054 // -------------------------------- 00055 int main() { 00056 int i; 00057 int currentLED = 0; 00058 char rMess[NUMMESS][LCDCHARLEN]={LGREEN, LRED}; // for LCD 00059 char pMess[NUMMESS][LCDCHARLEN]={PRED, PGREEN}; // for pc serial port 00060 00061 initialize_global_vars(); //keep things organized 00062 LEDs[currentLED] = LEDON; 00063 LCDMess(rMess[currentLED]); 00064 pc.printf(pMess[currentLED]); 00065 // End of setup 00066 while(true) { 00067 if (ButtonTimer > BUTTONTIME){ 00068 for (i=0; i<NUMBUTS; i++){ // index will be 0 or 1 00069 if(!buttons[i]) { 00070 allLEDsOff(); 00071 LCDMess(rMess[i]); 00072 pc.printf(pMess[i]); 00073 currentLED = i; 00074 } // if ! buttons 00075 }// for loop to look at buttons 00076 ButtonTimer.reset(); 00077 } 00078 if(LEDTimer.read() > BLINKTIME){ 00079 LEDTimer.reset(); 00080 ledState = !ledState; // Flip the general state 00081 LEDs[currentLED] = ledState; 00082 } 00083 // Do other things here between times of reading and flashing 00084 } 00085 }
Generated on Tue Jul 19 2022 05:12:45 by
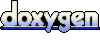