
Component Test's Software to work with "Universal Controller Box" - Software is an interpreter or "compiler" for programs to be done with a .txt file and read off of the SD Card
Dependencies: BridgeDriver FrontPanelButtons MCP23017 SDFileSystem TextLCD mbed
VoltageDriver.cpp
00001 #include "VoltageDriver.hpp" 00002 00003 //Constructor 00004 VoltageDriver::VoltageDriver(LineData lineData){ 00005 this->errorFlag = 0; 00006 00007 //Order of Line: Command, Local_Name, VOLTAGE_DRIVER, Channel(1,2,3,4,5,6,7,8) 00008 if (lineData.numWords != 4) 00009 this->errorFlag = 1; 00010 00011 string channelstr = lineData.word[3]; //Parameter is a number 00012 int numValuesFound = sscanf(channelstr.c_str(), "%d", &channel); 00013 if (numValuesFound < 1) 00014 this->errorFlag = 1; 00015 00016 //Channel Value between 1 to 8 00017 if (channel >= 1 && channel <= 8){ 00018 00019 switch (channel){ 00020 case 1: 00021 case 2: 00022 bridges.enablePwm(BridgeDriver::MOTOR_A, 0); 00023 break; 00024 case 3: 00025 case 4: 00026 bridges.enablePwm(BridgeDriver::MOTOR_B, 0); 00027 break; 00028 case 5: 00029 case 6: 00030 bridges.enablePwm(BridgeDriver::MOTOR_C, 0); 00031 break; 00032 case 7: 00033 case 8: 00034 bridges.enablePwm(BridgeDriver::MOTOR_D, 0); 00035 break; 00036 } 00037 } 00038 00039 //Channel does not fall into valid selection 00040 else 00041 this->errorFlag = 1; 00042 } 00043 00044 00045 int VoltageDriver::getChannel(){ 00046 return this->channel; 00047 } 00048 00049 00050 //A line consists of [ __(Local_Name)__ __(function)__ __(parameter1)__ __(parameter2)__ __(parameter3)__ ... and so on] 00051 int VoltageDriver::interpret(LineData &lineData){ 00052 00053 //Order of line: local_name, function_name, param1, param2, param3,....... 00054 string func = lineData.word[1]; 00055 00056 /******************************************************************************/ 00057 /*** <func: forcebrake> ***/ 00058 /******************************************************************************/ 00059 if (func.compare("forcebrake") == 0){ 00060 00061 if (lineData.numWords != 2){ 00062 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00063 return -1; 00064 } 00065 00066 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00067 if (DummyMode) 00068 return 0; //Function operated successfully but doesn't return a value 00069 00070 bridges.forceBrake(channel); 00071 00072 //Record the settings for Pause and Resume 00073 currState = 0; 00074 } 00075 00076 00077 /******************************************************************************/ 00078 /*** <func: drive> ***/ 00079 /******************************************************************************/ 00080 else if (func.compare("drive") == 0){ 00081 //order of line: local_name, drive 00082 00083 if (lineData.numWords != 2){ 00084 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00085 return -1; 00086 } 00087 00088 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00089 if (DummyMode) 00090 return 0; //Function operated successfully but doesn't return a value 00091 00092 bridges.drive(channel, 1); //turn channel on 00093 00094 //Record the settings for Pause and Resume 00095 currState = 1; 00096 } 00097 00098 00099 /******************************************************************************/ 00100 /**** <func: off> ****/ 00101 /******************************************************************************/ 00102 else if (func.compare("off") == 0){ 00103 00104 if (lineData.numWords != 2){ 00105 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00106 return -1; 00107 } 00108 00109 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00110 if (DummyMode) 00111 return 0; //Function operated successfully but doesn't return a value 00112 00113 off(); 00114 } 00115 00116 00117 else { 00118 ErrorOut("Unknown Command for Voltage Driver Class", lineData.lineNumber); 00119 return -1; 00120 } 00121 00122 return 0; //Return success as 0 since no condition had to be met 00123 } 00124 00125 00126 //For stopping the entire system if an error occurs, can be called from main 00127 int VoltageDriver::off(void){ 00128 bridges.drive(getChannel(), 0); //turn channel off 00129 00130 //Record the settings for Pause and Resume, and exit 00131 currState = 0; 00132 return 0; 00133 } 00134 00135 00136 //Stop the driver without changing the previous known settings, so that it will be saved on resume 00137 int VoltageDriver::pause(void){ 00138 bridges.drive(getChannel(), 0); //turn channel off 00139 return 0; 00140 } 00141 00142 //Resume the driver using its previously known settings 00143 int VoltageDriver::resume(void){ 00144 bridges.drive(getChannel(), this->currState); //turn channel off 00145 return 0; 00146 } 00147 00148
Generated on Sun Jul 24 2022 01:49:11 by
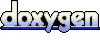