
Component Test's Software to work with "Universal Controller Box" - Software is an interpreter or "compiler" for programs to be done with a .txt file and read off of the SD Card
Dependencies: BridgeDriver FrontPanelButtons MCP23017 SDFileSystem TextLCD mbed
TimerDevice.cpp
00001 #include "TimerDevice.hpp" 00002 00003 //Constructor 00004 TimerDevice::TimerDevice(LineData lineData){ 00005 //No Constructor needed... functionality already exists in mBed library 00006 } 00007 00008 00009 //A line consists of [ __(Local_Name)__ __(function)__ __(parameter1)__ __(parameter2)__ __(parameter3)__ ... and so on] 00010 int TimerDevice::interpret(LineData &lineData){ 00011 00012 00013 00014 //Order of line: local_name, function_name, param1, param2, param3,....... 00015 string func = lineData.word[1]; 00016 00017 /******************************************************************************/ 00018 /*** <func: start> ***/ 00019 /******************************************************************************/ 00020 if (func.compare("start") == 0){ 00021 00022 if (lineData.numWords != 2){ 00023 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00024 return -1; 00025 } 00026 00027 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00028 if (DummyMode) 00029 return 0; //Function operated successfully but doesn't return a value 00030 00031 this->Timer.start(); 00032 } 00033 00034 00035 /******************************************************************************/ 00036 /*** <func: stop> ***/ 00037 /******************************************************************************/ 00038 else if (func.compare("stop") == 0){ 00039 00040 if (lineData.numWords != 2){ 00041 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00042 return -1; 00043 } 00044 00045 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00046 if (DummyMode) 00047 return 0; //Function operated successfully but doesn't return a value 00048 00049 this->Timer.stop(); 00050 } 00051 00052 /******************************************************************************/ 00053 /*** <func: reset> ***/ 00054 /******************************************************************************/ 00055 else if (func.compare("reset") == 0){ 00056 00057 if (lineData.numWords != 2){ 00058 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00059 return -1; 00060 } 00061 00062 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00063 if (DummyMode) 00064 return 0; //Function operated successfully but doesn't return a value 00065 00066 this->Timer.stop(); 00067 this->Timer.reset(); 00068 this->Timer.start(); 00069 } 00070 00071 /******************************************************************************/ 00072 /**** <func: val> ****/ 00073 /******************************************************************************/ 00074 else if (func.compare("val") == 0){ 00075 00076 //line looks like: local_name, val, comparison_characteristics (<, >, =) 00077 if (lineData.numWords != 4){ 00078 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00079 return -1; 00080 } 00081 00082 string cmpChar = lineData.word[2]; //Get the comparison characteristic value 00083 00084 string timestr = lineData.word[3]; //Parameter is a number 00085 int timeValue = 0; //Time value to compare in ms 00086 int numValuesFound = sscanf(timestr.c_str(), "%d", &timeValue); 00087 if (numValuesFound < 1){ 00088 ErrorOut("Parameter Unknown, time value can't be converted", lineData.lineNumber); 00089 return -1; 00090 } 00091 00092 //Error check comparision characteristic 00093 if (cmpChar.compare("<") != 0 && cmpChar.compare(">") != 0 && cmpChar.compare("=") != 0){ 00094 ErrorOut("Parameter Unknown, comparison character can't be converted", lineData.lineNumber); 00095 return -1; 00096 } 00097 00098 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00099 if (DummyMode) 00100 return 0; //Function operated successfully but doesn't return a value 00101 00102 if (cmpChar.compare("<") == 0){ 00103 if (this->Timer.read_ms() < timeValue) 00104 return 1; //met condition 00105 } 00106 else if (cmpChar.compare(">") == 0){ 00107 if (this->Timer.read_ms() > timeValue) 00108 return 1; //met condition 00109 } 00110 else if (cmpChar.compare("=") == 0){ 00111 if (this->Timer.read_ms() == timeValue) 00112 return 1; //met condition 00113 } 00114 00115 } 00116 00117 00118 else { 00119 ErrorOut("Unknown Command for Voltage Driver Class", lineData.lineNumber); 00120 return -1; 00121 } 00122 00123 return 0; //Return success as 0 since no condition had to be met 00124 } 00125 00126 00127 //For stopping the entire system if an error occurs, can be called from main 00128 int TimerDevice::off(void){ 00129 this->Timer.stop(); 00130 return 0; 00131 } 00132 00133 00134 //Pause the timer 00135 int TimerDevice::pause(void){ 00136 this->Timer.stop(); 00137 return 0; 00138 } 00139 00140 //Resume the timer 00141 int TimerDevice::resume(void){ 00142 this->Timer.start(); 00143 return 0; 00144 } 00145 00146
Generated on Sun Jul 24 2022 01:49:11 by
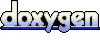