
Component Test's Software to work with "Universal Controller Box" - Software is an interpreter or "compiler" for programs to be done with a .txt file and read off of the SD Card
Dependencies: BridgeDriver FrontPanelButtons MCP23017 SDFileSystem TextLCD mbed
Motor.cpp
00001 #include "Motor.hpp" 00002 00003 //Constructor 00004 Motor::Motor(LineData lineData){ 00005 this->errorFlag = 0; 00006 00007 //Order of Line: Command, Local_Name, MOTOR, MotorID(A,B,C,D) 00008 //Since we can't return from a constructor, instead we'll flip a flag, and check it after we've added the device in interpretCommand 00009 if (lineData.numWords != 4) 00010 this->errorFlag = 1; 00011 00012 string channel = lineData.word[3]; //Parameter is a single character, so dereference the point to the word 00013 00014 if ((channel.compare("A") == 0) || (channel.compare("a") == 0)) 00015 this->motor = BridgeDriver::MOTOR_A; 00016 else if ((channel.compare("B") == 0) || (channel.compare("b") == 0)) 00017 this->motor = BridgeDriver::MOTOR_B; 00018 else if ((channel.compare("C") == 0) || (channel.compare("c") == 0)) 00019 this->motor = BridgeDriver::MOTOR_C; 00020 else if ((channel.compare("D") == 0) || (channel.compare("d") == 0)) 00021 this->motor = BridgeDriver::MOTOR_D; 00022 00023 //MotorID not known 00024 else 00025 this->errorFlag = 1; 00026 00027 bridges.enablePwm(this->motor, 1); 00028 } 00029 00030 00031 enum BridgeDriver::Motors Motor::getMotor(){ 00032 return this->motor; 00033 } 00034 00035 //A line consists of [ __(Local_Name)__ __(function)__ __(parameter1)__ __(parameter2)__ __(parameter3)__ ... and so on] 00036 int Motor::interpret(LineData &lineData){ 00037 00038 //Order of Line: Local_Name, Function_Name, Param1, Param2, Param3,....... 00039 string func = lineData.word[1]; 00040 00041 /******************************************************************************/ 00042 /*** <Func: enableBrake> ***/ 00043 /******************************************************************************/ 00044 if (func.compare("enableBrake") == 0){ 00045 00046 if (lineData.numWords != 3){ 00047 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00048 return -1; 00049 } 00050 00051 //Initialize and Convert Parameters 00052 string enable = lineData.word[2]; 00053 int enableValue = 0; 00054 00055 int numValuesFound = sscanf(enable.c_str(), "%d", &enableValue); 00056 if (numValuesFound < 1){ 00057 ErrorOut("Parameter Unknown, enableBrake value can't be converted", lineData.lineNumber); 00058 return -1; 00059 } 00060 00061 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00062 if (DummyMode) 00063 return 0; //Function operated successfully but doesn't return a value 00064 00065 bridges.enableBraking(getMotor(), enableValue); 00066 } 00067 00068 /******************************************************************************/ 00069 /*** <Func: forceBrake> ***/ 00070 /******************************************************************************/ 00071 else if (func.compare("forceBrake") == 0){ 00072 00073 if (lineData.numWords != 2){ 00074 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00075 return -1; 00076 } 00077 00078 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00079 if (DummyMode) 00080 return 0; //Function operated successfully but doesn't return a value 00081 00082 bridges.forceBrake(getMotor()); 00083 00084 //Record the settings for Pause and Resume 00085 currDir = 0; 00086 currSpeed = 0; 00087 } 00088 00089 00090 /******************************************************************************/ 00091 /*** <Func: drive> ***/ 00092 /******************************************************************************/ 00093 else if (func.compare("drive") == 0){ 00094 00095 if (lineData.numWords != 4){ 00096 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00097 return -1; 00098 } 00099 00100 //Initialize Parameters 00101 string speed = lineData.word[2]; 00102 string dir = lineData.word[3]; 00103 00104 //Initialize Convertion Variables if needed 00105 float speedValue; 00106 int dirValue = 0; 00107 00108 //Convert string to usable values 00109 int numValuesFound = sscanf(speed.c_str(), "%f", &speedValue); 00110 if (numValuesFound < 1){ 00111 ErrorOut("Parameter Unknown, speed value can't be converted", lineData.lineNumber); 00112 return -1; 00113 } 00114 00115 //Speed is given as a percentage of 100, so convert it to the value needed for the bridge.drive function 00116 speedValue = speedValue / 100; 00117 00118 00119 if (speedValue <= 0 && speedValue > 1.0){ 00120 ErrorOut("Speed Value must be between 0 - 100", lineData.lineNumber); 00121 return -1; 00122 } 00123 00124 if (dir.compare("CC") == 0 || dir.compare("cc") == 0) 00125 dirValue = -1; //Turn Clockwise 00126 else if (dir.compare("C") == 0 || dir.compare("c") == 0) 00127 dirValue = 1; //Turn CounterClockwise 00128 00129 else{ 00130 ErrorOut("Direction Value must be C or CC", lineData.lineNumber); 00131 return -1; 00132 } 00133 00134 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00135 if (DummyMode) 00136 return 0; //Function operated successfully but doesn't return a value 00137 00138 bridges.drive(getMotor(), dirValue, speedValue); //Turn on the Motor 00139 00140 //Record the settings for Pause and Resume 00141 currDir = dirValue; 00142 currSpeed = speedValue; 00143 } 00144 00145 00146 /******************************************************************************/ 00147 /**** <Func: off> ****/ 00148 /******************************************************************************/ 00149 else if (func.compare("off") == 0){ 00150 00151 if (lineData.numWords != 2){ 00152 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00153 return -1; 00154 } 00155 00156 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00157 if (DummyMode) 00158 return 0; //Function operated successfully but doesn't return a value 00159 00160 off(); 00161 } 00162 00163 else { 00164 ErrorOut("Unknown Command for Motor Class", lineData.lineNumber); 00165 return -1; 00166 } 00167 00168 return 0; //Return success as 0 since no condition had to be met 00169 } 00170 00171 00172 00173 //For stopping the entire system if an error occurs, can be called from main 00174 int Motor::off(void){ 00175 bridges.drive(getMotor(), 0, 0); //Turn off the Motor 00176 00177 //Record the settings for Pause and Resume, and exit 00178 currDir = 0; 00179 currSpeed = 0; 00180 return 0; 00181 } 00182 00183 00184 //Stop the motor without changing the previous known settings, so that it will be saved on resume 00185 int Motor::pause(void){ 00186 bridges.drive(getMotor(), 0, 0); //Turn off the Motor 00187 return 0; 00188 } 00189 00190 //Resume the motor using its previously known settings 00191 int Motor::resume(void){ 00192 bridges.drive(getMotor(), this->currDir, this->currSpeed); //Resume Motor from it's last known state 00193 return 0; 00194 }
Generated on Sun Jul 24 2022 01:49:11 by
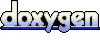