
Component Test's Software to work with "Universal Controller Box" - Software is an interpreter or "compiler" for programs to be done with a .txt file and read off of the SD Card
Dependencies: BridgeDriver FrontPanelButtons MCP23017 SDFileSystem TextLCD mbed
CAN_Device.cpp
00001 #include "CAN_Device.hpp" 00002 00003 //Constructor 00004 CAN_Device::CAN_Device(LineData lineData){ 00005 00006 //Order of Line: Command, Local_Name, CAN_DEVICE, selectedCAN(1,2), freq 00007 //Since we can't return from a constructor, instead we'll flip a flag, and check it after we've added the device in interpretCommand 00008 if (lineData.numWords != 5) 00009 this->errorFlag = 1; 00010 00011 string channelstr = lineData.word[3], freqstr = lineData.word[4]; //Parameters are numbers 00012 int tempChannel = -1, tempFreq = -1; 00013 int numValuesFound = sscanf(channelstr.c_str(), "%d", &tempChannel); 00014 if (numValuesFound < 1) 00015 this->errorFlag = 1; 00016 00017 numValuesFound = sscanf(freqstr.c_str(), "%d", &tempFreq); 00018 if (numValuesFound < 1) 00019 this->errorFlag = 1; 00020 00021 //channel must be either CAN1 or CAN2 00022 //CAN 1 is on the Back of the Box, CAN2 is on the Front of the Box 00023 if (tempChannel == 1 || tempChannel == 2){ 00024 if (tempChannel == 1){ 00025 this->canDevice = new CAN (p30, p29); 00026 // this->pinRD = p30; 00027 // this->pinTD = p29; 00028 } 00029 else if (tempChannel == 2){ 00030 this->canDevice = new CAN (p9, p10); 00031 // this->pinRD = p9; 00032 // this->pinTD = p10; 00033 } 00034 00035 this->selectedCAN = tempChannel; 00036 } 00037 00038 else 00039 this->errorFlag = 1; 00040 00041 //should have a frequency greater than 0 00042 if (tempFreq > 0) 00043 this->canDevice->frequency(tempFreq); 00044 else 00045 this->errorFlag = 1; 00046 } 00047 00048 00049 //int CAN_Device::getSelectedCAN(){ 00050 // return this->selectedCAN; 00051 //} 00052 // 00053 //int CAN_Device::getFreq(){ 00054 // return this->freq; 00055 //} 00056 00057 //A line consists of [ __(Local_Name)__ __(function)__ __(parameter1)__ __(parameter2)__ __(parameter3)__ ... and so on] 00058 int CAN_Device::CAN_Device(LineData &lineData){ 00059 // 00060 // //Initialize the CAN device 00061 // CAN canDevice(pinRD, pinTD); 00062 // canDevice.frequency(125000); 00063 00064 //Order of Line: Local_Name, Function_Name, Param1, Param2, Param3,....... 00065 string func = lineData.word[1]; 00066 00067 /******************************************************************************/ 00068 /*** <Func: write> ***/ 00069 /******************************************************************************/ 00070 if (func.compare("write") == 0){ 00071 //line looks like: Local_Name, write, ID_val, length_val, data 00072 if (lineData.numWords < 5){ 00073 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00074 return -1; 00075 } 00076 00077 //Initialize and Convert Parameters 00078 string _id = lineData.word[2]; 00079 string _length = lineData.word[3]; 00080 string _data = lineData.word[4]; 00081 int _idValue = 0; 00082 char _lengthValue = 0; 00083 00084 int numValuesFound = sscanf(_id.c_str(), "%d", &_idValue); 00085 if (numValuesFound < 1){ 00086 ErrorOut("Parameter Unknown, id value can't be converted", lineData.lineNumber); 00087 return -1; 00088 } 00089 00090 int numValuesFound = sscanf(_length.c_str(), "%c", &_lengthValue); 00091 if (numValuesFound < 1){ 00092 ErrorOut("Parameter Unknown, length value can't be converted", lineData.lineNumber); 00093 return -1; 00094 } 00095 00096 int numDataVals = numWords - 4; //number of data bytes present in the line 00097 const char* dataBytes[numDataVals]; 00098 int i = 0; 00099 for (i = 0; i < numDataVals; i++){ 00100 string thisDataByte = lineData.word[4 + i]; 00101 int numValuesFound = sscanf(thisDataByte.c_str(), "%c", &dataBytes[i]); 00102 if (numValuesFound < 1){ 00103 ErrorOut("Parameter Unknown, a data byte %d can't be converted", (i + 1), lineData.lineNumber); 00104 return -1; 00105 } 00106 } 00107 00108 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00109 if (DummyMode) 00110 return 0; //Function operated successfully but doesn't return a value 00111 00112 00113 canDevice->write(CANMessage(_idValue, &dataBytes, _lengthValue)); 00114 } 00115 00116 /******************************************************************************/ 00117 /*** <Func: read> ***/ 00118 /******************************************************************************/ 00119 else if (func.compare("forceBrake") == 0){ 00120 00121 if (lineData.numWords != 2){ 00122 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00123 return -1; 00124 } 00125 00126 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00127 if (DummyMode) 00128 return 0; //Function operated successfully but doesn't return a value 00129 00130 bridges.forceBrake(getMotor()); 00131 } 00132 00133 00134 /******************************************************************************/ 00135 /*** <Func: drive> ***/ 00136 /******************************************************************************/ 00137 else if (func.compare("drive") == 0){ 00138 00139 if (lineData.numWords != 4){ 00140 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00141 return -1; 00142 } 00143 00144 //Initialize Parameters 00145 string speed = lineData.word[2]; 00146 string dir = lineData.word[3]; 00147 00148 //Initialize Convertion Variables if needed 00149 float speedValue; 00150 int dirValue = 0; 00151 00152 //Convert string to usable values 00153 int numValuesFound = sscanf(speed.c_str(), "%f", &speedValue); 00154 if (numValuesFound < 1){ 00155 ErrorOut("Parameter Unknown, speed value can't be converted", lineData.lineNumber); 00156 return -1; 00157 } 00158 00159 //Speed is given as a percentage of 100, so convert it to the value needed for the bridge.drive function 00160 speedValue = speedValue / 100; 00161 00162 00163 if (speedValue <= 0 && speedValue > 1.0){ 00164 ErrorOut("Speed Value must be between 0 - 100", lineData.lineNumber); 00165 return -1; 00166 } 00167 00168 if (dir.compare("CC") == 0 || dir.compare("cc") == 0) 00169 dirValue = -1; //Turn Clockwise 00170 else if (dir.compare("C") == 0 || dir.compare("c") == 0) 00171 dirValue = 1; //Turn CounterClockwise 00172 00173 else{ 00174 ErrorOut("Direction Value must be C or CC", lineData.lineNumber); 00175 return -1; 00176 } 00177 00178 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00179 if (DummyMode) 00180 return 0; //Function operated successfully but doesn't return a value 00181 00182 bridges.drive(getMotor(), dirValue, speedValue); //Turn on the Motor 00183 } 00184 00185 00186 /******************************************************************************/ 00187 /**** <Func: off> ****/ 00188 /******************************************************************************/ 00189 else if (func.compare("off") == 0){ 00190 00191 if (lineData.numWords != 2){ 00192 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00193 return -1; 00194 } 00195 00196 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00197 if (DummyMode) 00198 return 0; //Function operated successfully but doesn't return a value 00199 00200 off(); 00201 } 00202 00203 else { 00204 ErrorOut("Unknown Command for Motor Class", lineData.lineNumber); 00205 return -1; 00206 } 00207 00208 return 0; //Return success as 0 since no condition had to be met 00209 } 00210 00211 00212 00213 //For stopping the entire system if an error occurs, can be called from main 00214 int CAN_Device::off(void){ 00215 bridges.drive(getMotor(), 0, 0); //Turn off the Motor 00216 return 0; 00217 }
Generated on Sun Jul 24 2022 01:49:11 by
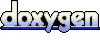