mbed-dev library fork for STM32F100R6 microcontroller (LQFP64, 24MHz, 32kB flash, 4kB ram, 2-channel DAC, HDMI CEC, very cheap) . Use in online compiler (instead mbed library) with selected platform Nucleo F103RB.
Fork of mbed-dev by
SerialBase.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_SERIALBASE_H 00017 #define MBED_SERIALBASE_H 00018 00019 #include "platform.h" 00020 00021 // for 100F6 not implemented (N.S.) 00022 #undef DEVICE_SERIAL_ASYNCH 00023 00024 // for 100F6 not implemented (N.S.) 00025 #undef DEVICE_SERIAL_FC 00026 00027 00028 00029 #if DEVICE_SERIAL 00030 00031 #include "Stream.h" 00032 #include "FunctionPointer.h" 00033 #include "serial_api.h" 00034 00035 #if DEVICE_SERIAL_ASYNCH 00036 #include "CThunk.h" 00037 #include "dma_api.h" 00038 #endif 00039 00040 namespace mbed { 00041 00042 /** A base class for serial port implementations 00043 * Can't be instantiated directly (use Serial or RawSerial) 00044 */ 00045 class SerialBase { 00046 00047 public: 00048 /** Set the baud rate of the serial port 00049 * 00050 * @param baudrate The baudrate of the serial port (default = 9600). 00051 */ 00052 void baud(int baudrate); 00053 00054 enum Parity { 00055 None = 0, 00056 Odd, 00057 Even, 00058 Forced1, 00059 Forced0 00060 }; 00061 00062 enum IrqType { 00063 RxIrq = 0, 00064 TxIrq 00065 }; 00066 00067 enum Flow { 00068 Disabled = 0, 00069 RTS, 00070 CTS, 00071 RTSCTS 00072 }; 00073 00074 /** Set the transmission format used by the serial port 00075 * 00076 * @param bits The number of bits in a word (5-8; default = 8) 00077 * @param parity The parity used (SerialBase::None, SerialBase::Odd, SerialBase::Even, SerialBase::Forced1, SerialBase::Forced0; default = SerialBase::None) 00078 * @param stop The number of stop bits (1 or 2; default = 1) 00079 */ 00080 void format(int bits=8, Parity parity=SerialBase::None, int stop_bits=1); 00081 00082 /** Determine if there is a character available to read 00083 * 00084 * @returns 00085 * 1 if there is a character available to read, 00086 * 0 otherwise 00087 */ 00088 int readable(); 00089 00090 /** Determine if there is space available to write a character 00091 * 00092 * @returns 00093 * 1 if there is space to write a character, 00094 * 0 otherwise 00095 */ 00096 int writeable(); 00097 00098 /** Attach a function to call whenever a serial interrupt is generated 00099 * 00100 * @param fptr A pointer to a void function, or 0 to set as none 00101 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00102 */ 00103 void attach(void (*fptr)(void), IrqType type=RxIrq); 00104 00105 /** Attach a member function to call whenever a serial interrupt is generated 00106 * 00107 * @param tptr pointer to the object to call the member function on 00108 * @param mptr pointer to the member function to be called 00109 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00110 */ 00111 template<typename T> 00112 void attach(T* tptr, void (T::*mptr)(void), IrqType type=RxIrq) { 00113 if((mptr != NULL) && (tptr != NULL)) { 00114 _irq[type].attach(tptr, mptr); 00115 serial_irq_set(&_serial, (SerialIrq)type, 1); 00116 } else { 00117 serial_irq_set(&_serial, (SerialIrq)type, 0); 00118 } 00119 } 00120 00121 /** Generate a break condition on the serial line 00122 */ 00123 void send_break(); 00124 00125 #if DEVICE_SERIAL_FC 00126 /** Set the flow control type on the serial port 00127 * 00128 * @param type the flow control type (Disabled, RTS, CTS, RTSCTS) 00129 * @param flow1 the first flow control pin (RTS for RTS or RTSCTS, CTS for CTS) 00130 * @param flow2 the second flow control pin (CTS for RTSCTS) 00131 */ 00132 void set_flow_control(Flow type, PinName flow1=NC, PinName flow2=NC); 00133 #endif 00134 00135 static void _irq_handler(uint32_t id, SerialIrq irq_type); 00136 00137 #if DEVICE_SERIAL_ASYNCH 00138 00139 /** Begin asynchronous write using 8bit buffer. The completition invokes registered TX event callback 00140 * 00141 * @param buffer The buffer where received data will be stored 00142 * @param length The buffer length in bytes 00143 * @param callback The event callback function 00144 * @param event The logical OR of TX events 00145 */ 00146 int write(const uint8_t *buffer, int length, const event_callback_t & callback, int event = SERIAL_EVENT_TX_COMPLETE); 00147 00148 /** Begin asynchronous write using 16bit buffer. The completition invokes registered TX event callback 00149 * 00150 * @param buffer The buffer where received data will be stored 00151 * @param length The buffer length in bytes 00152 * @param callback The event callback function 00153 * @param event The logical OR of TX events 00154 */ 00155 int write(const uint16_t *buffer, int length, const event_callback_t & callback, int event = SERIAL_EVENT_TX_COMPLETE); 00156 00157 /** Abort the on-going write transfer 00158 */ 00159 void abort_write(); 00160 00161 /** Begin asynchronous reading using 8bit buffer. The completition invokes registred RX event callback. 00162 * 00163 * @param buffer The buffer where received data will be stored 00164 * @param length The buffer length in bytes 00165 * @param callback The event callback function 00166 * @param event The logical OR of RX events 00167 * @param char_match The matching character 00168 */ 00169 int read(uint8_t *buffer, int length, const event_callback_t & callback, int event = SERIAL_EVENT_RX_COMPLETE, unsigned char char_match = SERIAL_RESERVED_CHAR_MATCH); 00170 00171 /** Begin asynchronous reading using 16bit buffer. The completition invokes registred RX event callback. 00172 * 00173 * @param buffer The buffer where received data will be stored 00174 * @param length The buffer length in bytes 00175 * @param callback The event callback function 00176 * @param event The logical OR of RX events 00177 * @param char_match The matching character 00178 */ 00179 int read(uint16_t *buffer, int length, const event_callback_t & callback, int event = SERIAL_EVENT_RX_COMPLETE, unsigned char char_match = SERIAL_RESERVED_CHAR_MATCH); 00180 00181 /** Abort the on-going read transfer 00182 */ 00183 void abort_read(); 00184 00185 /** Configure DMA usage suggestion for non-blocking TX transfers 00186 * 00187 * @param usage The usage DMA hint for peripheral 00188 * @return Zero if the usage was set, -1 if a transaction is on-going 00189 */ 00190 int set_dma_usage_tx(DMAUsage usage); 00191 00192 /** Configure DMA usage suggestion for non-blocking RX transfers 00193 * 00194 * @param usage The usage DMA hint for peripheral 00195 * @return Zero if the usage was set, -1 if a transaction is on-going 00196 */ 00197 int set_dma_usage_rx(DMAUsage usage); 00198 00199 protected: 00200 void start_read(void *buffer, int buffer_size, char buffer_width, const event_callback_t & callback, int event, unsigned char char_match); 00201 void start_write(const void *buffer, int buffer_size, char buffer_width, const event_callback_t & callback, int event); 00202 void interrupt_handler_asynch(void); 00203 #endif 00204 00205 protected: 00206 SerialBase(PinName tx, PinName rx); 00207 virtual ~SerialBase() { 00208 } 00209 00210 int _base_getc(); 00211 int _base_putc(int c); 00212 00213 #if DEVICE_SERIAL_ASYNCH 00214 CThunk<SerialBase> _thunk_irq; 00215 event_callback_t _tx_callback; 00216 event_callback_t _rx_callback; 00217 DMAUsage _tx_usage; 00218 DMAUsage _rx_usage; 00219 #endif 00220 00221 serial_t _serial; 00222 FunctionPointer _irq[2]; 00223 int _baud; 00224 00225 }; 00226 00227 } // namespace mbed 00228 00229 #endif 00230 00231 #endif
Generated on Wed Jul 13 2022 04:55:01 by
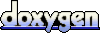