
simple test EEPROM emulation (STM algorithm described in the application notes: AN4061, AN3969, AN2594, AN3390, AN4056) for STM32F091
EEPROM_Emulation
Functions | |
static HAL_StatusTypeDef | EE_Format (void) |
Erases PAGE and PAGE1 and writes VALID_PAGE header to PAGE. | |
static uint16_t | EE_FindValidPage (uint8_t Operation) |
Find valid Page for write or read operation. | |
static uint16_t | EE_VerifyPageFullWriteVariable (uint16_t VirtAddress, uint16_t Data) |
Verify if active page is full and Writes variable in EEPROM. | |
static uint16_t | EE_PageTransfer (uint16_t VirtAddress, uint16_t Data) |
Transfers last updated variables data from the full Page to an empty one. | |
static uint16_t | EE_VerifyPageFullyErased (uint32_t Address) |
Verify if specified page is fully erased. | |
uint16_t | EE_Init (void) |
Restore the pages to a known good state in case of page's status corruption after a power loss. | |
uint16_t | EE_ReadVariable (uint16_t VirtAddress, uint16_t *Data) |
Returns the last stored variable data, if found, which correspond to the passed virtual address. | |
uint16_t | EE_WriteVariable (uint16_t VirtAddress, uint16_t Data) |
Writes/upadtes variable data in EEPROM. |
Function Documentation
static uint16_t EE_FindValidPage | ( | uint8_t | Operation ) | [static] |
Find valid Page for write or read operation.
- Parameters:
-
Operation,: operation to achieve on the valid page. This parameter can be one of the following values: - READ_FROM_VALID_PAGE: read operation from valid page
- WRITE_IN_VALID_PAGE: write operation from valid page
- Return values:
-
Valid page number (PAGE or PAGE1) or NO_VALID_PAGE in case of no valid page was found
Definition at line 512 of file eeprom.cpp.
static HAL_StatusTypeDef EE_Format | ( | void | ) | [static] |
Erases PAGE and PAGE1 and writes VALID_PAGE header to PAGE.
- Parameters:
-
None
- Return values:
-
Status of the last operation (Flash write or erase) done during EEPROM formating
Definition at line 461 of file eeprom.cpp.
uint16_t EE_Init | ( | void | ) |
Restore the pages to a known good state in case of page's status corruption after a power loss.
- Parameters:
-
None.
- Return values:
-
- Flash error code: on write Flash error - FLASH_COMPLETE: on success
Definition at line 76 of file eeprom.cpp.
static uint16_t EE_PageTransfer | ( | uint16_t | VirtAddress, |
uint16_t | Data | ||
) | [static] |
Transfers last updated variables data from the full Page to an empty one.
- Parameters:
-
VirtAddress,: 16 bit virtual address of the variable Data,: 16 bit data to be written as variable value
- Return values:
-
Success or error status: - FLASH_COMPLETE: on success
- PAGE_FULL: if valid page is full
- NO_VALID_PAGE: if no valid page was found
- Flash error code: on write Flash error
Definition at line 645 of file eeprom.cpp.
uint16_t EE_ReadVariable | ( | uint16_t | VirtAddress, |
uint16_t * | Data | ||
) |
Returns the last stored variable data, if found, which correspond to the passed virtual address.
- Parameters:
-
VirtAddress,: Variable virtual address Data,: Global variable contains the read variable value
- Return values:
-
Success or error status: - 0: if variable was found
- 1: if the variable was not found
- NO_VALID_PAGE: if no valid page was found.
Definition at line 378 of file eeprom.cpp.
static uint16_t EE_VerifyPageFullWriteVariable | ( | uint16_t | VirtAddress, |
uint16_t | Data | ||
) | [static] |
Verify if active page is full and Writes variable in EEPROM.
- Parameters:
-
VirtAddress,: 16 bit virtual address of the variable Data,: 16 bit data to be written as variable value
- Return values:
-
Success or error status: - FLASH_COMPLETE: on success
- PAGE_FULL: if valid page is full
- NO_VALID_PAGE: if no valid page was found
- Flash error code: on write Flash error
Definition at line 584 of file eeprom.cpp.
uint16_t EE_VerifyPageFullyErased | ( | uint32_t | Address ) | [static] |
Verify if specified page is fully erased.
- Parameters:
-
Address,: page address This parameter can be one of the following values: - PAGE0_BASE_ADDRESS: Page0 base address
- PAGE1_BASE_ADDRESS: Page1 base address
- Return values:
-
page fully erased status: - 0: if Page not erased
- 1: if Page erased
Definition at line 329 of file eeprom.cpp.
uint16_t EE_WriteVariable | ( | uint16_t | VirtAddress, |
uint16_t | Data | ||
) |
Writes/upadtes variable data in EEPROM.
- Parameters:
-
VirtAddress,: Variable virtual address Data,: 16 bit data to be written
- Return values:
-
Success or error status: - FLASH_COMPLETE: on success
- PAGE_FULL: if valid page is full
- NO_VALID_PAGE: if no valid page was found
- Flash error code: on write Flash error
Definition at line 437 of file eeprom.cpp.
Generated on Fri Jul 22 2022 14:32:51 by
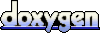