Lib to read the MCP9808 over Initialized I2C bus
Embed:
(wiki syntax)
Show/hide line numbers
MCP9808.h
00001 #ifndef MPC9808_H 00002 #define MPC9808_H 00003 00004 #include "mbed.h" 00005 00006 /** MCP9808 class. 00007 * Used for read MCP9808 ±0.5°C Maximum Accuracy Digital Temperature Sensor 00008 * 00009 * Example: 00010 * @code 00011 *#include "mbed.h" 00012 *#include "MCP9808.h" 00013 * 00014 *DigitalOut myled(LED1); 00015 *I2C i2cBus(D14,D15); 00016 *MCP9808 therm(&i2cBus, true, true, true); 00017 * 00018 *int main() 00019 *{ 00020 * while(1) 00021 * { 00022 * myled = !myled; // Toggle LED 00023 * wait(0.2); // 200 ms 00024 * printf("Temperature=%03.6f*C\r\n",therm.getTemp()); 00025 * } 00026 *} 00027 * @endcode 00028 */ 00029 class MCP9808 00030 { 00031 public: 00032 /** Create MCP9808 instance connected to I2C bus 00033 * @param *i2c I2C bus already initialized 00034 * @param a0 bool indicate a0 pin state to compute I2C address 00035 * @param a1 bool indicate a1 pin state to compute I2C address 00036 * @param a2 bool indicate a2 pin state to compute I2C address 00037 */ 00038 MCP9808(I2C *i2c, bool a0=false, bool a1=false, bool a2=false); 00039 00040 /**Get MCP9808 abient temperature 00041 * @returns temperature [°C] 00042 */ 00043 float getTemp(void); 00044 00045 #ifdef MBED_OPERATORS 00046 /** An operator shorthand for getTemp() 00047 * 00048 * The float() operator can be used as a shorthand for getTemp() to simplify common code sequences 00049 * 00050 * Example: 00051 * @code 00052 * float x = therm.getTemp(); 00053 * float x = therm; 00054 * 00055 * if(therm.getTemp() > 20.25) { ... } 00056 * if(therm > 20.25) { ... } 00057 * @endcode 00058 */ 00059 operator float(){return getTemp();} 00060 #endif 00061 00062 private: 00063 I2C *_i2c; 00064 int _addr; 00065 }; 00066 00067 #endif
Generated on Thu Jul 14 2022 11:37:13 by
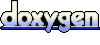