Lib to read the MCP9808 over Initialized I2C bus
Embed:
(wiki syntax)
Show/hide line numbers
MCP9808.cpp
00001 #include "MCP9808.h" 00002 00003 //***********************************/************************************ 00004 // Constant // 00005 //***********************************/************************************ 00006 #define MCP9808_I2C_ADDR 0x30 //( 18<<1) 00007 00008 #define MCP9808_REG_CONFIG 0x01 00009 #define MCP9808_REG_TUPPER 0x02 00010 #define MCP9808_REG_TLOWER 0x03 00011 #define MCP9808_REG_TCRIT 0x04 00012 #define MCP9808_REG_TAMB 0x05 00013 #define MCP9808_REG_MANID 0x06 00014 #define MCP9808_REG_DEVID 0x07 00015 #define MCP9808_REG_RESOL 0x08 00016 00017 #define MCP9808_TAMB_MASK 0x0F 00018 #define MCP9808_SIGN_POS 0x03 00019 00020 //***********************************/************************************ 00021 // Constructors // 00022 //***********************************/************************************ 00023 MCP9808::MCP9808(I2C *i2c, bool a0, bool a1, bool a2):_i2c(i2c) 00024 { 00025 _addr = (MCP9808_I2C_ADDR | (a2<<3) | (a1<<2) | (a0<<1)); 00026 } 00027 00028 float MCP9808::getTemp(void) 00029 { 00030 char reg = MCP9808_REG_TAMB; 00031 float ta; 00032 char buf[2]; 00033 00034 _i2c->write(_addr, ®, 1); 00035 _i2c->read(_addr, buf, 2); 00036 00037 /* 00038 if(buf[0] & (1<<MCP9808_SIGN_POS)) 00039 { 00040 buf[0] &= MCP9808_TAMB_MASK; 00041 00042 ta = 256 - (buf[0]*16 + buf[1]*0.0625); 00043 } 00044 else 00045 { 00046 buf[0] &= MCP9808_TAMB_MASK; 00047 ta = (buf[0]*16 + buf[1]*0.0625); 00048 }*/ 00049 00050 ta = ((buf[0]&MCP9808_TAMB_MASK) << 8) | buf[1]; 00051 ta /= 16.0; 00052 00053 if(buf[0] & (1<<MCP9808_SIGN_POS)) 00054 { 00055 ta -= 256; 00056 } 00057 00058 return ta; 00059 } 00060
Generated on Thu Jul 14 2022 11:37:13 by
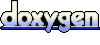