MAX31855 Cold-Junction Compensated Thermocouple-to-Digital Converter
Embed:
(wiki syntax)
Show/hide line numbers
MAX31855.h
00001 #ifndef MAX31855_H 00002 #define MAX31855_H 00003 00004 #include "mbed.h" 00005 /** MAX31855 class. 00006 * Used for read MAX31855 Cold-Junction Compensated Thermocouple-to-Digital Converter 00007 * 00008 * Example: 00009 * @code 00010 * DigitalOut led(LED2); 00011 * Serial pc(USBTX,USBRX); 00012 * MAX31855 therm(p5,p6,p7,p8); 00013 * 00014 * int main() 00015 * { 00016 * while(1) 00017 * { 00018 * pc.printf("T=%f;Chip=%f\r\n",therm.thermocouple(),therm.chip()); 00019 * led = therm.fault(); 00020 * wait(0.5); 00021 * } 00022 * } 00023 * @endcode 00024 */ 00025 class MAX31855 00026 { 00027 public: 00028 /** Create MAX31855 instance connected to spi & ncs 00029 * @param mosi SPI master out slave in pin (MAX31855 is only read device) 00030 * @param miso SPI master in slave out pin 00031 * @param sck SPI clock pin 00032 * @param ncs pin to connect at CS input 00033 */ 00034 MAX31855(PinName mosi, PinName miso, PinName sck, PinName ncs); 00035 00036 /** Create MAX31855 instance connected to spi & ncs 00037 * @param SPI bus instance (MAX31855 is only read device) 00038 * @param ncs pin to connect at CS input 00039 */ 00040 MAX31855(SPI& spi, PinName ncs); 00041 00042 /**Get Thermocouple temperature 00043 * @returns temperature [°C] 00044 */ 00045 float thermocouple(void); 00046 00047 /**Get Chip temperature 00048 * @returns temperature [°C] 00049 */ 00050 float chip(void); 00051 00052 /**Check if thermocouple disconnected 00053 */ 00054 bool opened(void); 00055 00056 /**Check if an error 00057 */ 00058 bool fault(void); 00059 00060 /**Check if thermocouple short-circuited to Vcc 00061 */ 00062 bool scToVcc(void); 00063 00064 /**Check if thermocouple shorted-circuited to GND 00065 */ 00066 bool scToGnd(void); 00067 00068 #ifdef MBED_OPERATORS 00069 /** An operator shorthand for thermocouple() 00070 * 00071 * The float() operator can be used as a shorthand for thermocouple() to simplify common code sequences 00072 * 00073 * Example: 00074 * @code 00075 * float x = temp.thermocouple(); 00076 * float x = temp; 00077 * 00078 * if(temp.thermocouple() > 20.25) { ... } 00079 * if(temp > 20.25) { ... } 00080 * @endcode 00081 */ 00082 operator float(){return thermocouple();} 00083 #endif 00084 00085 protected: 00086 void read(void); 00087 00088 private: 00089 SPI _spi; 00090 DigitalOut _ncs; 00091 float _t; 00092 float _chip_t; 00093 bool _fault; 00094 bool _scv; 00095 bool _scg; 00096 bool _oc; 00097 }; 00098 00099 #endif
Generated on Tue Jul 19 2022 08:19:19 by
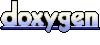