
Version one of final code
Dependencies: ARCH_GPRS_V2_HW Blinker GPRSInterface HTTPClient_GPRS SDFileSystem USBDevice mbed
finalCode.cpp
00001 //SEED Team 20 Final Code draft 1 4/16/15 00002 00003 #include "mbed.h" 00004 #include "SDFileSystem.h" 00005 #include "ARCH_GPRS_V2_HW.h" 00006 #include "HTTPClient.h" 00007 #include "GPRSInterface.h" 00008 #include "Blinker.h" 00009 #include "DHT.h" 00010 #include "i2c_uart.h" 00011 #include "ARCH_GPRS_Sleep.h" 00012 00013 #define PIN_TX P1_27 00014 #define PIN_RX P1_26 00015 00016 #define BROADCAST_TIME 20 00017 //LED Blink 00018 Blinker yellowLED(LED1), redLED(LED2), greenLED(LED3), blueLED(LED4); 00019 00020 //USBSerial pc; 00021 SDFileSystem sd(P1_22, P1_21, P1_20, P1_23, "sd"); // the pinout on the /Arch GPRS v2 mbed board. 00022 00023 //variables for reading data from SD card 00024 char APIKey[17]; 00025 char sensors[3][5]; 00026 char sensorBuff[5]; 00027 int tempField, humField, lightField; 00028 int field1, field2, field3; 00029 int numSensors; 00030 bool tempSensorflag=false, lightSensorflag=false, SomeotherSensorflag=false; 00031 00032 //GPRS setup 00033 GPRSInterface gprs(PIN_TX,PIN_RX,115200,"internetd.gdsp",NULL,NULL); 00034 HTTPClient http; 00035 char* thingSpeakUrl = "http://api.thingspeak.com/update"; 00036 char urlBuffer[256]; 00037 char str[1024]; 00038 00039 //Sensors 00040 DHT sensor(P1_14,SEN51035P); 00041 AnalogIn lightSensor(P0_12); 00042 00043 00044 int tempData,humData, lightData; 00045 00046 00047 void connectGPRS(void) { 00048 iot_hw.init(); // power on SIM900 00049 00050 int count = 0; 00051 while(false == gprs.connect() && count < 5) { 00052 wait(2); 00053 count += 1; 00054 } 00055 } 00056 00057 void getTempHumid(int* tempData,int* humData){ 00058 int err = 1; 00059 int count = 0; 00060 iot_hw.grovePwrOn(); 00061 wait(1); // wait 1 second for device stable status 00062 while (err != 0 && count < 4) { 00063 err = sensor.readData(); 00064 count += 1; 00065 *tempData = sensor.ReadTemperature(FARENHEIT); 00066 *humData = sensor.ReadHumidity(); 00067 00068 wait(1); 00069 } 00070 iot_hw.grovePwrOff(); 00071 } 00072 00073 void getLightReading(int* lightData) 00074 { 00075 *lightData = lightSensor*1000; 00076 } 00077 00078 00079 int ReadFile (void) { 00080 mkdir("/sd", 0777); // All other times open file in append mode 00081 FILE *fp = fopen("/sd/config.txt","r"); 00082 if (fp==NULL) { 00083 fclose(fp); 00084 return 0; 00085 00086 } else if (fp) { 00087 fscanf(fp,"%16c %d %s %s", APIKey, &numSensors, sensors[0],sensors[1]); 00088 // fscanf(fp,"%16c",APIKey); 00089 // pc.printf("APIKEY= %s\r\n",APIKey); 00090 // 00091 // fscanf(fp,"%d",&numSensors); 00092 // pc.printf("number of sensors = %d \r\n",numSensors); 00093 // 00094 // for (int i = 0; i<numSensors;i=i+1) { 00095 // //fscanf(fp,"%s",sensors[i]); 00096 // fscanf(fp,"%s",sensorBuff); 00097 // strcpy(sensors[i],sensorBuff); 00098 // pc.printf("sensor %d = %s\r\n",i,sensors[i]); 00099 // } 00100 00101 00102 fclose(fp); 00103 return 1; 00104 00105 } else { 00106 fclose(fp); 00107 return 0; 00108 } 00109 00110 } 00111 00112 bool stringComparison (const char *string1, const char *string2) { 00113 int count = 0; 00114 while (string1[count] != 0) { 00115 if (string1[count] != string2[count]) 00116 return false; 00117 count++; 00118 } 00119 return true; 00120 } 00121 00122 00123 void sendData1(int sensor1Data, int field1) { 00124 connectGPRS(); 00125 urlBuffer[0] = 0; 00126 sprintf(urlBuffer, "%s?key=%s&field%d=%d", thingSpeakUrl, APIKey, field1, sensor1Data); 00127 HTTPResult res = http.get(urlBuffer, str,128); 00128 if (res != HTTP_OK) { 00129 redLED.blink(5); 00130 } else { 00131 greenLED.blink(5); 00132 } 00133 iot_hw.init_io(); //power down SIM900 00134 } 00135 00136 void sendData2(int sensor1Data, int field1, int sensor2Data, int field2) { 00137 connectGPRS(); 00138 urlBuffer[0] = 0; 00139 sprintf(urlBuffer, "%s?key=%s&field%d=%d&field%d=%d", thingSpeakUrl, APIKey, field1, sensor1Data, field2, sensor2Data); 00140 HTTPResult res = http.get(urlBuffer, str,128); 00141 if (res != HTTP_OK) { 00142 redLED.blink(5); 00143 } else { 00144 greenLED.blink(5); 00145 } 00146 iot_hw.init_io(); //power down SIM900 00147 } 00148 00149 void sendData3(int sensor1Data, int field1, int sensor2Data, int field2, int sensor3Data, int field3) { 00150 connectGPRS(); 00151 urlBuffer[0] = 0; 00152 sprintf(urlBuffer, "%s?key=%s&field%d=%d&field%d=%d&field%d=%d", thingSpeakUrl, APIKey, field1, sensor1Data, field2, sensor2Data, field3, sensor3Data); 00153 HTTPResult res = http.get(urlBuffer, str,128); 00154 if (res != HTTP_OK) { 00155 redLED.blink(5); 00156 } else { 00157 greenLED.blink(5); 00158 } 00159 iot_hw.init_io(); //power down SIM900 00160 } 00161 00162 void sdWrite1(int sensor1Data) 00163 { 00164 00165 mkdir("/sd", 0777); 00166 FILE *fp = fopen("/sd/node1.csv","a"); 00167 if (fp == NULL) { 00168 //no sd card 00169 redLED.blink(10); 00170 } else { 00171 00172 fprintf(fp,"timestamp, %d \r\n",sensor1Data); 00173 fclose(fp); 00174 blueLED.blink(5); 00175 } 00176 } 00177 00178 void sdWrite2(int sensor1Data, int sensor2Data) 00179 { 00180 mkdir("/sd", 0777); 00181 FILE *fp = fopen("/sd/node1.csv","a"); 00182 if (fp == NULL) { 00183 //no sd card 00184 redLED.blink(10); 00185 } else { 00186 00187 fprintf(fp,"timestamp, %d, %d\r\n",sensor1Data, sensor2Data); 00188 fclose(fp); 00189 blueLED.blink(5); 00190 } 00191 } 00192 00193 void sdWrite3(int sensor1Data, int sensor2Data,int sensor3Data) 00194 { 00195 mkdir("/sd", 0777); 00196 FILE *fp = fopen("/sd/node1.csv","a"); 00197 if (fp == NULL) { 00198 //no sd card 00199 redLED.blink(10); 00200 } else { 00201 00202 fprintf(fp,"timestamp, %d, %d, %d\r\n",sensor1Data, sensor2Data, sensor3Data); 00203 fclose(fp); 00204 blueLED.blink(5); 00205 } 00206 } 00207 00208 00209 00210 00211 int main() { 00212 wdt_sleep.wdtClkSetup(WDTCLK_SRC_IRC_OSC); //set up sleep 00213 00214 //read SD card 00215 while (ReadFile()==0) { 00216 wait(5); 00217 redLED.blink(5); 00218 } 00219 greenLED.blink(10); 00220 00221 //identify sensors and find order of fields 00222 int field=1; 00223 for (int i=0;i<numSensors;i=i+1) { 00224 if (stringComparison(sensors[i],"temp")==true) { 00225 tempSensorflag=true; 00226 tempField=field; 00227 humField=field+1; 00228 field=field+1; 00229 } else if (stringComparison(sensors[i],"light")==true) { 00230 lightSensorflag=true; 00231 lightField=field; 00232 } else if (stringComparison(sensors[i],"some other sensor")==true) { 00233 SomeotherSensorflag=true; 00234 } 00235 field=field+1; 00236 } 00237 00238 while(1) 00239 { 00240 00241 if (numSensors == 3) { 00242 00243 } else if (numSensors ==2) { 00244 if (tempSensorflag==true) { 00245 if (lightSensorflag==true) { 00246 getLightReading(&lightData); 00247 getTempHumid(&tempData,&humData); 00248 sendData3(tempData,tempField,humData,humField,lightData,lightField); 00249 sdWrite3(tempData,humData,lightData); 00250 } else if (SomeotherSensorflag==true) { 00251 00252 } 00253 } else if (lightSensorflag==true) { 00254 if (SomeotherSensorflag==true) { 00255 getLightReading(&lightData); 00256 00257 00258 } 00259 } 00260 } else { 00261 if (tempSensorflag==true) { 00262 getTempHumid(&tempData,&humData); 00263 sendData2(tempData,tempField,humData,humField); 00264 sdWrite2(tempData,humData); 00265 } else if (lightSensorflag==true) { 00266 getLightReading(&lightData); 00267 sendData1(lightData,lightField); 00268 sdWrite1(lightData); 00269 } else if (SomeotherSensorflag==true) { 00270 00271 } 00272 } 00273 wdt_sleep.sleep(BROADCAST_TIME); 00274 00275 } 00276 }
Generated on Sun Jul 17 2022 22:01:51 by
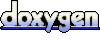