
simple example program to control one mirror from serial data
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "laserProjectorHardware.h" 00003 00004 DigitalOut myled(LED1); 00005 00006 void processSerial(); 00007 00008 unsigned int X, Y; // position of the mirror (note: in fact it is an ANGLE) 00009 bool newPositionReady=false; 00010 00011 unsigned int counter=0; 00012 00013 int main() { 00014 00015 // SETUP: -------------------------------------------------------------------------------------------- 00016 IO.init(); // note: serial speed can be changed by checking in the hardwareIO.cpp initialization 00017 00018 // Set displaying laser powers: 00019 IO.setRedPower(0); 00020 IO.setGreenPower(0);//turn on the green (displaying) laser 00021 00022 wait_ms(100); 00023 00024 Y = CENTER_AD_MIRROR_Y; 00025 IO.writeOutY(Y); 00026 00027 // MAIN LOOP: -------------------------------------------------------------------------------------------- 00028 while(1) { 00029 processSerial(); 00030 if (newPositionReady) { 00031 IO.writeOutY(Y); 00032 newPositionReady=false; 00033 } 00034 00035 Y=int(0.5*4095.0*(1.0+cos(1.0*counter/100000))); 00036 IO.writeOutY(Y); 00037 counter++; 00038 00039 } 00040 } 00041 00042 // -------------------------------------------------------------------------------------------- 00043 // String to store ALPHANUMERIC DATA (i.e., integers, floating point numbers, unsigned ints, etc represented as DEC) sent wirelessly: 00044 char stringData[24]; // note: an integer is two bytes long, represented with a maximum of 5 digits, but we may send floats or unsigned int... 00045 int indexStringData=0;//position of the byte in the string 00046 00047 void processSerial() { 00048 00049 while(pc.readable()>0){ 00050 00051 char val =pc.getc(); 00052 00053 // Save ASCII numeric characters (ASCII 0 - 9) on stringData: 00054 if ((val >= '0') && (val <= '9')){ // this is 45 to 57 (included) 00055 stringData[indexStringData] = val; 00056 indexStringData++; 00057 } 00058 00059 // X value? 00060 else if (val=='X') { 00061 stringData[indexStringData] = 0 ; 00062 X=atoi(stringData); 00063 indexStringData=0; 00064 //newPositionReady=true; 00065 } 00066 // Y value? 00067 else if (val=='Y') { 00068 stringData[indexStringData] = 0 ; 00069 Y=atoi(stringData); 00070 indexStringData=0; 00071 newPositionReady=true; 00072 } 00073 00074 } 00075 }
Generated on Thu Jul 21 2022 11:57:14 by
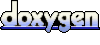