
simple example program to control one mirror from serial data
Embed:
(wiki syntax)
Show/hide line numbers
laserProjectorHardware.h
00001 00002 #ifndef hardwareIO_h 00003 #define hardwareIO_h 00004 00005 #include "mbed.h" 00006 00007 00008 //SPI library (for ADC chip) uses the following pins, but we don't have to set them and inputs or outputs 00009 // (this is done when the library is initialized, which is done by pre-instantiation: 00010 #define SCK_PIN p7 //SPI Clock 00011 #define MISO_PIN p6 //SPI input (data comming from DAC, here not connected) 00012 #define MOSI_PIN p5 //SPI output (data going to DAC) 00013 00014 //**** CHIP SELECT pins for MP4922 DAC (mirrors and red laser) 00015 // VERY IMPORTANT: the chip select for the DACs should be different from the default SPI "SS" pin (Slave Select), which is 53 by default (and will be used by the Ethernet Shield). 00016 #define CS_DAC_MIRRORS p8 //Chip Select of the first DAC (mirrors) 00017 00018 //**** LASERS pins: 00019 #define LASER_RED_PIN p28 // NOT YET USED (TTL control). NOTE: this is NOT the locking sensing laser! 00020 #define LASER_GREEN_PIN p29 // USED (TTL control) 00021 #define LASER_BLUE_PIN p30 // USED (TTL control) 00022 00023 //**** MIRRORS: 00024 //The DAC is 12 bytes capable (Max=4096), but we will limit this a little. 00025 #define MAX_AD_MIRRORS 4095 // note: 4095 is the absolute maximum for the SPI voltage (5V). This is for checking hardware compliance, but max and min angles can be defined for X and Y in each LivingSpot instance. 00026 #define MIN_AD_MIRRORS 0 // note: 0 is 0 volts for the SPI voltage. 00027 // We assume that the center of the mirror is at MAX_AD_MIRRORS/2 = 2000: 00028 #define CENTER_AD_MIRROR_X 2047 // This MUST BE the direction of the photodetector. 00029 #define CENTER_AD_MIRROR_Y 2047 // This MUST BE the direction of the photodetector. 00030 00031 00032 extern DigitalOut Laser_Red, Laser_Green, Laser_Blue; 00033 00034 // ================================================================================================================================================================== 00035 00036 class HardwareIO { 00037 public: 00038 00039 00040 void init(void); 00041 00042 void showLimitsMirrors( int times ); 00043 00044 // SPI control for DAC for mirrors and red laser power (low level): 00045 void writeOutX(unsigned short value); 00046 void writeOutY(unsigned short value); 00047 void writeOutXY(unsigned short valueX, unsigned short valueY) {writeOutX(valueX); writeOutY(valueY);}; 00048 00049 00050 //Displaying lasers: 00051 // Again: for the moment laser are TTL but these could be analog. Now, it is just: powerValue > 0 ==> 'true'; else 'false' 00052 // Red laser: 00053 void setRedPower(int powerRed); 00054 // Green laser: 00055 void setGreenPower(int powerGreen); 00056 // Blue laser: 00057 void setBluePower(int powerBlue); 00058 // Setting all colors at once: 00059 void setRGBPower(unsigned char color); // we will use the 3 LSB bits to set each color 00060 00061 00062 00063 private: 00064 00065 }; 00066 00067 00068 extern HardwareIO IO; // allows the object IO to be used in other .cpp files (IO is pre-instantiated in hardwareIO.cpp) 00069 // NOTE: IO encapsulates many IO functions, but perhaps it is better not to have an IO object - just use each IO function separatedly (as with pc object for instance) 00070 extern Serial pc; // allows pc to be manipulated by other .cpp files, even if pc is defined in the hardwareIO.cpp 00071 00072 00073 #endif
Generated on Thu Jul 21 2022 11:57:14 by
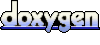