
simple example program to control one mirror from serial data
Embed:
(wiki syntax)
Show/hide line numbers
laserProjectorHardware.cpp
00001 #include "laserProjectorHardware.h" 00002 00003 HardwareIO IO; // preintantiation of cross-file global object IO 00004 00005 // -------------------------------------- (0) SETUP ALL IO (call this in the setup() function in main program) 00006 00007 Serial pc(USBTX, USBRX); // tx, rx 00008 00009 SPI spiDAC(MOSI_PIN, MISO_PIN, SCK_PIN); // mosi, miso, sclk 00010 DigitalOut csDAC(CS_DAC_MIRRORS); 00011 00012 DigitalOut Laser_Red(LASER_RED_PIN); // NOTE: this is NOT the lock in sensing laser (actually, not used yet) 00013 DigitalOut Laser_Green(LASER_GREEN_PIN); 00014 DigitalOut Laser_Blue(LASER_BLUE_PIN); 00015 00016 void HardwareIO::init(void) { 00017 Laser_Red = 0; // note: this is not the lockin-laser! 00018 Laser_Green = 0; 00019 Laser_Blue = 0; 00020 00021 //Serial Communication setup: 00022 pc.baud(115200);// 00023 // pc.baud(921600);//115200);// 00024 00025 // Setup the spi for 8 bit data, high steady state clock, 00026 // second edge capture, with a 10MHz clock rate 00027 csDAC = 1; 00028 spiDAC.format(16,0); 00029 spiDAC.frequency(16000000); 00030 00031 // default initial mirror position: 00032 writeOutX(CENTER_AD_MIRROR_X); 00033 writeOutY(CENTER_AD_MIRROR_Y); 00034 00035 } 00036 00037 //write on the first DAC, output A (mirror X) 00038 void HardwareIO::writeOutX(unsigned short value){ 00039 if(value > MAX_AD_MIRRORS) value = MAX_AD_MIRRORS; 00040 if(value < MIN_AD_MIRRORS) value = MIN_AD_MIRRORS; 00041 00042 value |= 0x7000; 00043 value &= 0x7FFF; 00044 00045 csDAC = 0; 00046 spiDAC.write(value); 00047 csDAC = 1; 00048 } 00049 00050 //write on the first DAC, output B (mirror Y) 00051 void HardwareIO::writeOutY(unsigned short value){ 00052 if(value > MAX_AD_MIRRORS) value = MAX_AD_MIRRORS; 00053 if(value < MIN_AD_MIRRORS) value = MIN_AD_MIRRORS; 00054 00055 value |= 0xF000; 00056 value &= 0xFFFF; 00057 00058 csDAC = 0; 00059 spiDAC.write(value); 00060 csDAC = 1; 00061 } 00062 00063 void HardwareIO::setRedPower(int powerValue){ 00064 if(powerValue > 0){ 00065 Laser_Red = 1; 00066 } 00067 else{ 00068 Laser_Red = 0; 00069 } 00070 } 00071 void HardwareIO::setGreenPower(int powerValue){ 00072 if(powerValue > 0){ 00073 Laser_Green = 1; 00074 } 00075 else{ 00076 Laser_Green = 0; 00077 } 00078 } 00079 void HardwareIO::setBluePower(int powerValue){ 00080 if(powerValue > 0){ 00081 Laser_Blue = 1; 00082 } 00083 else{ 00084 Laser_Blue = 0; 00085 } 00086 } 00087 void HardwareIO::setRGBPower(unsigned char color) { 00088 //lockin.setLaserPower(color&0x04>0? false : true); 00089 Laser_Red=color&0x04>>2; 00090 Laser_Green=(color&0x02)>>1; 00091 Laser_Blue =color&0x01; 00092 } 00093 00094 void HardwareIO::showLimitsMirrors(int times) { 00095 unsigned short pointsPerLine=150; 00096 int shiftX = (MAX_AD_MIRRORS - MIN_AD_MIRRORS) / pointsPerLine; 00097 int shiftY = (MAX_AD_MIRRORS - MIN_AD_MIRRORS) / pointsPerLine; 00098 00099 Laser_Green=1; 00100 00101 //for (int repeat=0; repeat<times; repeat++) { 00102 00103 Timer t; 00104 t.start(); 00105 while(t.read_ms()<times*1000) { 00106 00107 writeOutX(MIN_AD_MIRRORS);writeOutY(MIN_AD_MIRRORS); 00108 00109 for(int j=0; j<pointsPerLine; j++){ 00110 wait_us(200);//delay between each points 00111 writeOutY(j*shiftY + MIN_AD_MIRRORS); 00112 } 00113 00114 writeOutX(MIN_AD_MIRRORS);writeOutY(MAX_AD_MIRRORS); 00115 for(int j=0; j<pointsPerLine; j++) { 00116 wait_us(200);//delay between each points 00117 writeOutX(j*shiftX + MIN_AD_MIRRORS); 00118 } 00119 00120 writeOutX(MAX_AD_MIRRORS);writeOutY(MAX_AD_MIRRORS); 00121 for(int j=0; j<pointsPerLine; j++) { 00122 wait_us(200);//delay between each points 00123 writeOutY(-j*shiftX + MAX_AD_MIRRORS); 00124 } 00125 00126 writeOutX(MAX_AD_MIRRORS);writeOutY(MIN_AD_MIRRORS); 00127 for(int j=0; j<pointsPerLine; j++) { 00128 wait_us(200);//delay between each points 00129 writeOutX(-j*shiftX + MAX_AD_MIRRORS); 00130 } 00131 00132 } 00133 t.stop(); 00134 Laser_Green=0; 00135 }
Generated on Thu Jul 21 2022 11:57:14 by
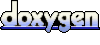