NUCLEO OK. Need to add pull ups for SDA and SCL. (3)
Embed:
(wiki syntax)
Show/hide line numbers
main_vcnl40x0.cpp
00001 /* 00002 Copyright (c) 2012 Vishay GmbH, www.vishay.com 00003 author: DS, version 1.2 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #define VERSION "\n Version: 1.2 01/2012\n" 00025 #define MAIN1 // select MAIN1, MAIN2, MAIN3 or MIAN4 00026 #define BAUD 9600 // increase up to 921600 for high speed communication (depends on terminal programm and USB mode) 00027 00028 #include "mbed.h" 00029 #include "VCNL40x0.h" 00030 00031 //VCNL40x0 VCNL40x0_Device (p28, p27, VCNL40x0_ADDRESS); // Define SDA, SCL pin and I2C address 00032 VCNL40x0 VCNL40x0_Device (I2C_SDA, I2C_SCL, VCNL40x0_ADDRESS); // Define SDA, SCL pin and I2C address 00033 DigitalOut mled0(LED1); // LED #1 00034 DigitalOut mled1(LED2); // LED #2 00035 DigitalOut mled2(LED3); // LED #3 00036 Serial pc(USBTX, USBRX); // Tx, Rx USB transmission 00037 00038 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00039 // main #1 00040 // Read Proximity on demand and Ambillight on demand in endless loop 00041 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00042 # ifdef MAIN1 00043 00044 int main() { 00045 unsigned char ID=0; 00046 unsigned char Current=0; 00047 unsigned int ProxiValue=0; 00048 unsigned int AmbiValue=0; 00049 00050 pc.baud(BAUD); // set USB speed (virtual COM port) 00051 00052 // print information on screen 00053 pc.printf("\n\n\r VCNL4010/4020 Proximity/Ambient Light Sensor"); 00054 pc.printf("\n\r library tested with mbed LPC1768 (ARM Cortex-M3 core) on www.mbed.org"); 00055 pc.printf(VERSION); 00056 pc.printf("\n\r Demonstration #1:"); 00057 pc.printf("\n\r Read Proximity on demand and Ambillight on demand in endless loop"); 00058 00059 VCNL40x0_Device.ReadID (&ID); // Read VCNL40x0 product ID revision register 00060 pc.printf("\n\n\r Product ID Revision Register: %d", ID); 00061 00062 VCNL40x0_Device.SetCurrent (20); // Set current to 200mA 00063 VCNL40x0_Device.ReadCurrent (&Current); // Read back IR LED current 00064 pc.printf("\n IR LED Current: %d\n\n", Current); 00065 00066 wait_ms(3000); // wait 3s (only for display) 00067 00068 // endless loop ///////////////////////////////////////////////////////////////////////////////////// 00069 00070 while (1) { 00071 //mled0 = 1; // LED on 00072 VCNL40x0_Device.ReadProxiOnDemand (&ProxiValue); // read prox value on demand 00073 VCNL40x0_Device.ReadAmbiOnDemand (&AmbiValue); // read ambi value on demand 00074 //mled0 = 0; // LED off 00075 00076 // pront values on screen 00077 pc.printf("\n\rProxi: %5.0i cts \tAmbi: %5.0i cts \tIlluminance: %7.2f lx", ProxiValue, AmbiValue, AmbiValue/4.0); 00078 wait_ms(500); // wait 3s (only for display) 00079 } 00080 } 00081 # endif 00082 00083 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00084 // main #2 00085 // Proximity Measurement in selfetimed mode with 4 measurements/s 00086 // Read prox value if ready with conversion, endless loop 00087 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00088 # ifdef MAIN2 00089 00090 int main() { 00091 unsigned char ID=0; 00092 unsigned char Command=0; 00093 unsigned char Current=0; 00094 unsigned int ProxiValue=0; 00095 unsigned int AmbiValue=0; 00096 00097 pc.baud(BAUD); 00098 00099 // print information on screen 00100 pc.printf("\n\n VCNL4010/4020 Proximity/Ambient Light Sensor"); 00101 pc.printf("\n library tested with mbed LPC1768 (ARM Cortex-M3 core) on www.mbed.org"); 00102 pc.printf(VERSION); 00103 pc.printf("\n Demonstration #2:"); 00104 pc.printf("\n Proximity Measurement in selftimed mode with 4 measurements/s"); 00105 pc.printf("\n Read prox value if ready with conversion, endless loop"); 00106 00107 VCNL40x0_Device.ReadID (&ID); // Read VCNL40x0 product ID revision register 00108 pc.printf("\n\n Product ID Revision Register: %d", ID); 00109 00110 VCNL40x0_Device.SetCurrent (20); // Set current to 200mA 00111 VCNL40x0_Device.ReadCurrent (&Current); // Read back IR LED current 00112 pc.printf("\n IR LED Current: %d\n\n", Current); 00113 00114 VCNL40x0_Device.SetProximityRate (PROX_MEASUREMENT_RATE_4); // set proximity rate to 4/s 00115 00116 // enable prox in selftimed mode 00117 VCNL40x0_Device.SetCommandRegister (COMMAND_PROX_ENABLE | COMMAND_SELFTIMED_MODE_ENABLE); 00118 00119 wait_ms(3000); // wait 3s (only for display) 00120 00121 // endless loop ///////////////////////////////////////////////////////////////////////////////////// 00122 00123 while (1) { 00124 00125 // wait on prox data ready bit 00126 do { 00127 VCNL40x0_Device.ReadCommandRegister (&Command); // read command register 00128 } while (!(Command & COMMAND_MASK_PROX_DATA_READY));// prox data ready ? 00129 00130 mled0 = 1; // LED on 00131 VCNL40x0_Device.ReadProxiValue (&ProxiValue); // read prox value 00132 mled0 = 0; // LED off 00133 00134 // print values on screen 00135 pc.printf("\nProxi: %5.0i cts", ProxiValue); 00136 } 00137 } 00138 # endif 00139 00140 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00141 // main #3 00142 // Proximity Measurement in selfetimed mode with 31 measurements/s 00143 // Interrupt waiting on proximity value > upper threshold limit 00144 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00145 # ifdef MAIN3 00146 00147 int main() { 00148 unsigned char ID=0; 00149 unsigned char Command=0; 00150 unsigned char Current=0; 00151 unsigned int ProxiValue=0; 00152 unsigned int AmbiValue=0; 00153 unsigned char InterruptStatus=0; 00154 unsigned char InterruptControl=0; 00155 00156 pc.baud(BAUD); 00157 00158 // print information on screen 00159 pc.printf("\n\n VCNL4010/4020 Proximity/Ambient Light Sensor"); 00160 pc.printf("\n library tested with mbed LPC1768 (ARM Cortex-M3 core) on www.mbed.org"); 00161 pc.printf(VERSION); 00162 pc.printf("\n Demonstration #3:"); 00163 pc.printf("\n Proximity Measurement in selfetimed mode with 31 measurements/s"); 00164 pc.printf("\n Interrupt waiting on proximity value > upper threshold limit"); 00165 00166 VCNL40x0_Device.ReadID (&ID); // Read VCNL40x0 product ID revision register 00167 pc.printf("\n\n Product ID Revision Register: %d", ID); 00168 00169 VCNL40x0_Device.SetCurrent (20); // Set current to 200mA 00170 VCNL40x0_Device.ReadCurrent (&Current); // Read back IR LED current 00171 pc.printf("\n IR LED Current: %d\n\n", Current); 00172 00173 // stop all activities (necessary for changing proximity rate, see datasheet) 00174 VCNL40x0_Device.SetCommandRegister (COMMAND_ALL_DISABLE); 00175 00176 // set proximity rate to 31/s 00177 VCNL40x0_Device.SetProximityRate (PROX_MEASUREMENT_RATE_31); 00178 00179 // enable prox in selftimed mode 00180 VCNL40x0_Device.SetCommandRegister (COMMAND_PROX_ENABLE | 00181 COMMAND_SELFTIMED_MODE_ENABLE); 00182 00183 // set interrupt control register 00184 VCNL40x0_Device.SetInterruptControl (INTERRUPT_THRES_SEL_PROX | 00185 INTERRUPT_THRES_ENABLE | 00186 INTERRUPT_COUNT_EXCEED_1); 00187 00188 VCNL40x0_Device.ReadInterruptControl (&InterruptControl); // Read back Interrupt Control register 00189 pc.printf("\n Interrupt Control Register: %i\n\n", InterruptControl); 00190 00191 00192 // wait on prox data ready bit 00193 do { 00194 VCNL40x0_Device.ReadCommandRegister (&Command); // read command register 00195 } while (!(Command & COMMAND_MASK_PROX_DATA_READY)); // prox data ready ? 00196 00197 VCNL40x0_Device.ReadProxiValue (&ProxiValue); // read prox value 00198 VCNL40x0_Device.SetHighThreshold (ProxiValue+100); // set high threshold for interrupt 00199 00200 wait_ms(3000); // wait 3s (only for display) 00201 00202 // endless loop ///////////////////////////////////////////////////////////////////////////////////// 00203 00204 while (1) { 00205 00206 // wait on prox data ready bit 00207 do { 00208 VCNL40x0_Device.ReadCommandRegister (&Command); // read command register 00209 } while (!(Command & COMMAND_MASK_PROX_DATA_READY));// prox data ready ? 00210 00211 mled0 = 1; // LED on 00212 VCNL40x0_Device.ReadProxiValue (&ProxiValue); // read prox value 00213 mled0 = 0; // LED off 00214 00215 // read interrupt status register 00216 VCNL40x0_Device.ReadInterruptStatus (&InterruptStatus); 00217 00218 // print prox value and interrupt status on screen 00219 pc.printf("\nProxi: %5.0i cts \tInterruptStatus: %i", ProxiValue, InterruptStatus); 00220 00221 // check interrupt status for High Threshold 00222 if (InterruptStatus & INTERRUPT_MASK_STATUS_THRES_HI) { 00223 mled1 = 1; // LED on 00224 VCNL40x0_Device.SetInterruptStatus (InterruptStatus); // clear Interrupt Status 00225 mled1 = 0; // LED on 00226 } 00227 } 00228 } 00229 # endif 00230 00231 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00232 // main #4 00233 // Proximity Measurement and Ambientlight Measurement in selftimed mode 00234 // Proximity with 31 measurements/s, Ambientlight with 2 measurement/s 00235 // Interrupt waiting on proximity value > upper threshold limit 00236 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00237 # ifdef MAIN4 00238 00239 int main() { 00240 unsigned int i=0; 00241 unsigned char ID=0; 00242 unsigned char Command=0; 00243 unsigned char Current=0; 00244 unsigned int ProxiValue=0; 00245 unsigned int SummeProxiValue=0; 00246 unsigned int AverageProxiValue=0; 00247 unsigned int AmbiValue=0; 00248 unsigned char InterruptStatus=0; 00249 unsigned char InterruptControl=0; 00250 00251 pc.baud(BAUD); 00252 00253 // print information on screen 00254 pc.printf("\n\n VCNL4010/4020 Proximity/Ambient Light Sensor"); 00255 pc.printf("\n library tested with mbed LPC1768 (ARM Cortex-M3 core) on www.mbed.org"); 00256 pc.printf(VERSION); 00257 pc.printf("\n Demonstration #4:"); 00258 pc.printf("\n Proximity Measurement and Ambient light Measurement in selftimed mode"); 00259 pc.printf("\n Proximity with 31 measurements/s, Ambient light with 2 measurement/s"); 00260 pc.printf("\n Interrupt waiting on proximity value > upper threshold limit"); 00261 00262 VCNL40x0_Device.ReadID (&ID); // Read VCNL40x0 product ID revision register 00263 pc.printf("\n\n Product ID Revision Register: %d", ID); 00264 00265 VCNL40x0_Device.SetCurrent (20); // Set current to 200mA 00266 VCNL40x0_Device.ReadCurrent (&Current); // Read back IR LED current 00267 pc.printf("\n IR LED Current: %d", Current); 00268 00269 // stop all activities (necessary for changing proximity rate, see datasheet) 00270 VCNL40x0_Device.SetCommandRegister (COMMAND_ALL_DISABLE); 00271 00272 // set proximity rate to 31/s 00273 VCNL40x0_Device.SetProximityRate (PROX_MEASUREMENT_RATE_31); 00274 00275 // enable prox and ambi in selftimed mode 00276 VCNL40x0_Device.SetCommandRegister (COMMAND_PROX_ENABLE | 00277 COMMAND_AMBI_ENABLE | 00278 COMMAND_SELFTIMED_MODE_ENABLE); 00279 00280 // set interrupt control for threshold 00281 VCNL40x0_Device.SetInterruptControl (INTERRUPT_THRES_SEL_PROX | 00282 INTERRUPT_THRES_ENABLE | 00283 INTERRUPT_COUNT_EXCEED_1); 00284 00285 // set ambient light measurement parameter 00286 VCNL40x0_Device.SetAmbiConfiguration (AMBI_PARA_AVERAGE_32 | 00287 AMBI_PARA_AUTO_OFFSET_ENABLE | 00288 AMBI_PARA_MEAS_RATE_2); 00289 00290 // measure average of prox value 00291 SummeProxiValue = 0; 00292 00293 for (i=0; i<30; i++) { 00294 do { // wait on prox data ready bit 00295 VCNL40x0_Device.ReadCommandRegister (&Command); // read command register 00296 } while (!(Command & COMMAND_MASK_PROX_DATA_READY)); // prox data ready ? 00297 00298 VCNL40x0_Device.ReadProxiValue (&ProxiValue); // read prox value 00299 00300 SummeProxiValue += ProxiValue; // Summary of all measured prox values 00301 } 00302 00303 AverageProxiValue = SummeProxiValue/30; // calculate average 00304 00305 VCNL40x0_Device.SetHighThreshold (AverageProxiValue+100); // set upper threshold for interrupt 00306 pc.printf("\n Upper Threshold Value: %i cts\n\n", AverageProxiValue+100); 00307 00308 wait_ms(2000); // wait 2s (only for display) 00309 00310 // endless loop ///////////////////////////////////////////////////////////////////////////////////// 00311 00312 while (1) { 00313 00314 // wait on data ready bit 00315 do { 00316 VCNL40x0_Device.ReadCommandRegister (&Command); // read command register 00317 } while (!(Command & (COMMAND_MASK_PROX_DATA_READY | COMMAND_MASK_AMBI_DATA_READY))); // data ready ? 00318 00319 // read interrupt status register 00320 VCNL40x0_Device.ReadInterruptStatus (&InterruptStatus); 00321 00322 // check interrupt status for High Threshold 00323 if (InterruptStatus & INTERRUPT_MASK_STATUS_THRES_HI) { 00324 mled2 = 1; // LED on, Interrupt 00325 VCNL40x0_Device.SetInterruptStatus (InterruptStatus); // clear Interrupt Status 00326 mled2 = 0; // LED off, Interrupt 00327 } 00328 00329 // prox value ready for using 00330 if (Command & COMMAND_MASK_PROX_DATA_READY) { 00331 mled0 = 1; // LED on, Prox Data Ready 00332 VCNL40x0_Device.ReadProxiValue (&ProxiValue); // read prox value 00333 00334 // print prox value and interrupt status on screen 00335 pc.printf("\nProxi: %5.0i cts \tInterruptStatus: %i", ProxiValue, InterruptStatus); 00336 00337 mled0 = 0; // LED off, Prox data Ready 00338 } 00339 00340 // ambi value ready for using 00341 if (Command & COMMAND_MASK_AMBI_DATA_READY) { 00342 mled1 = 1; // LED on, Ambi Data Ready 00343 VCNL40x0_Device.ReadAmbiValue (&AmbiValue); // read ambi value 00344 00345 // print ambi value and interrupt status on screen 00346 pc.printf("\n Ambi: %i", AmbiValue); 00347 00348 mled1 = 0; // LED off, Ambi Data Ready 00349 } 00350 } 00351 } 00352 # endif 00353
Generated on Sat Jul 16 2022 03:21:41 by
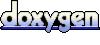