Official mbed Real Time Operating System based on the RTX implementation of the CMSIS-RTOS API open standard.
Dependents: denki-yohou_b TestY201 Network-RTOS NTPClient_HelloWorld ... more
RtosTimer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2012 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef RTOS_TIMER_H 00023 #define RTOS_TIMER_H 00024 00025 #include <stdint.h> 00026 #include "cmsis_os.h" 00027 #include "platform/Callback.h" 00028 #include "platform/toolchain.h" 00029 00030 namespace rtos { 00031 /** \addtogroup rtos */ 00032 /** @{*/ 00033 00034 /** The RtosTimer class allow creating and and controlling of timer functions in the system. 00035 A timer function is called when a time period expires whereby both on-shot and 00036 periodic timers are possible. A timer can be started, restarted, or stopped. 00037 00038 Timers are handled in the thread osTimerThread. 00039 Callback functions run under control of this thread and may use CMSIS-RTOS API calls. 00040 */ 00041 class RtosTimer { 00042 public: 00043 /** Create timer. 00044 @param func function to be executed by this timer. 00045 @param type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic) 00046 @param argument argument to the timer call back function. (default: NULL) 00047 @deprecated Replaced with RtosTimer(Callback<void()>, os_timer_type) 00048 */ 00049 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00050 "Replaced with RtosTimer(Callback<void()>, os_timer_type)") 00051 RtosTimer(void (*func)(void const *argument), os_timer_type type=osTimerPeriodic, void *argument=NULL) { 00052 constructor(mbed::callback((void (*)(void *))func, argument), type); 00053 } 00054 00055 /** Create timer. 00056 @param func function to be executed by this timer. 00057 @param type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic) 00058 */ 00059 RtosTimer(mbed::Callback<void()> func, os_timer_type type=osTimerPeriodic) { 00060 constructor(func, type); 00061 } 00062 00063 /** Create timer. 00064 @param obj pointer to the object to call the member function on. 00065 @param method member function to be executed by this timer. 00066 @param type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic) 00067 @deprecated 00068 The RtosTimer constructor does not support cv-qualifiers. Replaced by 00069 RtosTimer(callback(obj, method), os_timer_type). 00070 */ 00071 template <typename T, typename M> 00072 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00073 "The RtosTimer constructor does not support cv-qualifiers. Replaced by " 00074 "RtosTimer(callback(obj, method), os_timer_type).") 00075 RtosTimer(T *obj, M method, os_timer_type type=osTimerPeriodic) { 00076 constructor(mbed::callback(obj, method), type); 00077 } 00078 00079 /** Stop the timer. 00080 @return status code that indicates the execution status of the function. 00081 */ 00082 osStatus stop(void); 00083 00084 /** Start the timer. 00085 @param millisec time delay value of the timer. 00086 @return status code that indicates the execution status of the function. 00087 */ 00088 osStatus start(uint32_t millisec); 00089 00090 ~RtosTimer(); 00091 00092 private: 00093 // Required to share definitions without 00094 // delegated constructors 00095 void constructor(mbed::Callback<void()> func, os_timer_type type); 00096 00097 mbed::Callback<void()> _function; 00098 osTimerId _timer_id; 00099 osTimerDef_t _timer; 00100 #if defined(CMSIS_OS_RTX) && !defined(__MBED_CMSIS_RTOS_CM) 00101 uint32_t _timer_data[5]; 00102 #else 00103 uint32_t _timer_data[6]; 00104 #endif 00105 }; 00106 00107 } 00108 00109 #endif 00110 00111 /** @}*/
Generated on Tue Jul 12 2022 11:27:30 by
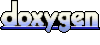