mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
pwmout_api.h
00001 00002 /** \addtogroup hal */ 00003 /** @{*/ 00004 /* mbed Microcontroller Library 00005 * Copyright (c) 2006-2013 ARM Limited 00006 * SPDX-License-Identifier: Apache-2.0 00007 * 00008 * Licensed under the Apache License, Version 2.0 (the "License"); 00009 * you may not use this file except in compliance with the License. 00010 * You may obtain a copy of the License at 00011 * 00012 * http://www.apache.org/licenses/LICENSE-2.0 00013 * 00014 * Unless required by applicable law or agreed to in writing, software 00015 * distributed under the License is distributed on an "AS IS" BASIS, 00016 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 */ 00020 #ifndef MBED_PWMOUT_API_H 00021 #define MBED_PWMOUT_API_H 00022 00023 #include "device.h" 00024 00025 #if DEVICE_PWMOUT 00026 00027 #ifdef __cplusplus 00028 extern "C" { 00029 #endif 00030 00031 /** Pwmout hal structure. pwmout_s is declared in the target's hal 00032 */ 00033 typedef struct pwmout_s pwmout_t; 00034 00035 /** 00036 * \defgroup hal_pwmout Pwmout hal functions 00037 * @{ 00038 */ 00039 00040 /** Initialize the pwm out peripheral and configure the pin 00041 * 00042 * @param obj The pwmout object to initialize 00043 * @param pin The pwmout pin to initialize 00044 */ 00045 void pwmout_init(pwmout_t *obj, PinName pin); 00046 00047 /** Deinitialize the pwmout object 00048 * 00049 * @param obj The pwmout object 00050 */ 00051 void pwmout_free(pwmout_t *obj); 00052 00053 /** Set the output duty-cycle in range <0.0f, 1.0f> 00054 * 00055 * Value 0.0f represents 0 percentage, 1.0f represents 100 percent. 00056 * @param obj The pwmout object 00057 * @param percent The floating-point percentage number 00058 */ 00059 void pwmout_write(pwmout_t *obj, float percent); 00060 00061 /** Read the current float-point output duty-cycle 00062 * 00063 * @param obj The pwmout object 00064 * @return A floating-point output duty-cycle 00065 */ 00066 float pwmout_read(pwmout_t *obj); 00067 00068 /** Set the PWM period specified in seconds, keeping the duty cycle the same 00069 * 00070 * Periods smaller than microseconds (the lowest resolution) are set to zero. 00071 * @param obj The pwmout object 00072 * @param seconds The floating-point seconds period 00073 */ 00074 void pwmout_period(pwmout_t *obj, float seconds); 00075 00076 /** Set the PWM period specified in miliseconds, keeping the duty cycle the same 00077 * 00078 * @param obj The pwmout object 00079 * @param ms The milisecond period 00080 */ 00081 void pwmout_period_ms(pwmout_t *obj, int ms); 00082 00083 /** Set the PWM period specified in microseconds, keeping the duty cycle the same 00084 * 00085 * @param obj The pwmout object 00086 * @param us The microsecond period 00087 */ 00088 void pwmout_period_us(pwmout_t *obj, int us); 00089 00090 /** Set the PWM pulsewidth specified in seconds, keeping the period the same. 00091 * 00092 * @param obj The pwmout object 00093 * @param seconds The floating-point pulsewidth in seconds 00094 */ 00095 void pwmout_pulsewidth(pwmout_t *obj, float seconds); 00096 00097 /** Set the PWM pulsewidth specified in miliseconds, keeping the period the same. 00098 * 00099 * @param obj The pwmout object 00100 * @param ms The floating-point pulsewidth in miliseconds 00101 */ 00102 void pwmout_pulsewidth_ms(pwmout_t *obj, int ms); 00103 00104 /** Set the PWM pulsewidth specified in microseconds, keeping the period the same. 00105 * 00106 * @param obj The pwmout object 00107 * @param us The floating-point pulsewidth in microseconds 00108 */ 00109 void pwmout_pulsewidth_us(pwmout_t *obj, int us); 00110 00111 /**@}*/ 00112 00113 #ifdef __cplusplus 00114 } 00115 #endif 00116 00117 #endif 00118 00119 #endif 00120 00121 /** @}*/
Generated on Tue Jul 12 2022 20:41:15 by
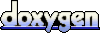