mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
i2c_api.h
00001 00002 /** \addtogroup hal */ 00003 /** @{*/ 00004 /* mbed Microcontroller Library 00005 * Copyright (c) 2006-2015 ARM Limited 00006 * SPDX-License-Identifier: Apache-2.0 00007 * 00008 * Licensed under the Apache License, Version 2.0 (the "License"); 00009 * you may not use this file except in compliance with the License. 00010 * You may obtain a copy of the License at 00011 * 00012 * http://www.apache.org/licenses/LICENSE-2.0 00013 * 00014 * Unless required by applicable law or agreed to in writing, software 00015 * distributed under the License is distributed on an "AS IS" BASIS, 00016 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 */ 00020 #ifndef MBED_I2C_API_H 00021 #define MBED_I2C_API_H 00022 00023 #include "device.h" 00024 #include "hal/buffer.h" 00025 00026 #if DEVICE_I2C_ASYNCH 00027 #include "hal/dma_api.h" 00028 #endif 00029 00030 #if DEVICE_I2C 00031 00032 /** 00033 * @defgroup hal_I2CEvents I2C Events Macros 00034 * 00035 * @{ 00036 */ 00037 #define I2C_EVENT_ERROR (1 << 1) 00038 #define I2C_EVENT_ERROR_NO_SLAVE (1 << 2) 00039 #define I2C_EVENT_TRANSFER_COMPLETE (1 << 3) 00040 #define I2C_EVENT_TRANSFER_EARLY_NACK (1 << 4) 00041 #define I2C_EVENT_ALL (I2C_EVENT_ERROR | I2C_EVENT_TRANSFER_COMPLETE | I2C_EVENT_ERROR_NO_SLAVE | I2C_EVENT_TRANSFER_EARLY_NACK) 00042 00043 /**@}*/ 00044 00045 #if DEVICE_I2C_ASYNCH 00046 /** Asynch I2C HAL structure 00047 */ 00048 typedef struct { 00049 struct i2c_s i2c; /**< Target specific I2C structure */ 00050 struct buffer_s tx_buff; /**< Tx buffer */ 00051 struct buffer_s rx_buff; /**< Rx buffer */ 00052 } i2c_t; 00053 00054 #else 00055 /** Non-asynch I2C HAL structure 00056 */ 00057 typedef struct i2c_s i2c_t; 00058 00059 #endif 00060 00061 enum { 00062 I2C_ERROR_NO_SLAVE = -1, 00063 I2C_ERROR_BUS_BUSY = -2 00064 }; 00065 00066 #ifdef __cplusplus 00067 extern "C" { 00068 #endif 00069 00070 /** 00071 * \defgroup hal_GeneralI2C I2C Configuration Functions 00072 * @{ 00073 */ 00074 00075 /** Initialize the I2C peripheral. It sets the default parameters for I2C 00076 * peripheral, and configures its specifieds pins. 00077 * 00078 * @param obj The I2C object 00079 * @param sda The sda pin 00080 * @param scl The scl pin 00081 */ 00082 void i2c_init(i2c_t *obj, PinName sda, PinName scl); 00083 00084 /** Configure the I2C frequency 00085 * 00086 * @param obj The I2C object 00087 * @param hz Frequency in Hz 00088 */ 00089 void i2c_frequency(i2c_t *obj, int hz); 00090 00091 /** Send START command 00092 * 00093 * @param obj The I2C object 00094 */ 00095 int i2c_start(i2c_t *obj); 00096 00097 /** Send STOP command 00098 * 00099 * @param obj The I2C object 00100 */ 00101 int i2c_stop(i2c_t *obj); 00102 00103 /** Blocking reading data 00104 * 00105 * @param obj The I2C object 00106 * @param address 7-bit address (last bit is 1) 00107 * @param data The buffer for receiving 00108 * @param length Number of bytes to read 00109 * @param stop Stop to be generated after the transfer is done 00110 * @return Number of read bytes 00111 */ 00112 int i2c_read(i2c_t *obj, int address, char *data, int length, int stop); 00113 00114 /** Blocking sending data 00115 * 00116 * @param obj The I2C object 00117 * @param address 7-bit address (last bit is 0) 00118 * @param data The buffer for sending 00119 * @param length Number of bytes to write 00120 * @param stop Stop to be generated after the transfer is done 00121 * @return 00122 * zero or non-zero - Number of written bytes 00123 * negative - I2C_ERROR_XXX status 00124 */ 00125 int i2c_write(i2c_t *obj, int address, const char *data, int length, int stop); 00126 00127 /** Reset I2C peripheral. TODO: The action here. Most of the implementation sends stop() 00128 * 00129 * @param obj The I2C object 00130 */ 00131 void i2c_reset(i2c_t *obj); 00132 00133 /** Read one byte 00134 * 00135 * @param obj The I2C object 00136 * @param last Acknoledge 00137 * @return The read byte 00138 */ 00139 int i2c_byte_read(i2c_t *obj, int last); 00140 00141 /** Write one byte 00142 * 00143 * @param obj The I2C object 00144 * @param data Byte to be written 00145 * @return 0 if NAK was received, 1 if ACK was received, 2 for timeout. 00146 */ 00147 int i2c_byte_write(i2c_t *obj, int data); 00148 00149 /**@}*/ 00150 00151 #if DEVICE_I2CSLAVE 00152 00153 /** 00154 * \defgroup SynchI2C Synchronous I2C Hardware Abstraction Layer for slave 00155 * @{ 00156 */ 00157 00158 /** Configure I2C as slave or master. 00159 * @param obj The I2C object 00160 * @param enable_slave Enable i2c hardware so you can receive events with ::i2c_slave_receive 00161 * @return non-zero if a value is available 00162 */ 00163 void i2c_slave_mode(i2c_t *obj, int enable_slave); 00164 00165 /** Check to see if the I2C slave has been addressed. 00166 * @param obj The I2C object 00167 * @return The status - 1 - read addresses, 2 - write to all slaves, 00168 * 3 write addressed, 0 - the slave has not been addressed 00169 */ 00170 int i2c_slave_receive(i2c_t *obj); 00171 00172 /** Configure I2C as slave or master. 00173 * @param obj The I2C object 00174 * @param data The buffer for receiving 00175 * @param length Number of bytes to read 00176 * @return non-zero if a value is available 00177 */ 00178 int i2c_slave_read(i2c_t *obj, char *data, int length); 00179 00180 /** Configure I2C as slave or master. 00181 * @param obj The I2C object 00182 * @param data The buffer for sending 00183 * @param length Number of bytes to write 00184 * @return non-zero if a value is available 00185 */ 00186 int i2c_slave_write(i2c_t *obj, const char *data, int length); 00187 00188 /** Configure I2C address. 00189 * @param obj The I2C object 00190 * @param idx Currently not used 00191 * @param address The address to be set 00192 * @param mask Currently not used 00193 */ 00194 void i2c_slave_address(i2c_t *obj, int idx, uint32_t address, uint32_t mask); 00195 00196 #endif 00197 00198 /**@}*/ 00199 00200 #if DEVICE_I2C_ASYNCH 00201 00202 /** 00203 * \defgroup hal_AsynchI2C Asynchronous I2C Hardware Abstraction Layer 00204 * @{ 00205 */ 00206 00207 /** Start I2C asynchronous transfer 00208 * 00209 * @param obj The I2C object 00210 * @param tx The transmit buffer 00211 * @param tx_length The number of bytes to transmit 00212 * @param rx The receive buffer 00213 * @param rx_length The number of bytes to receive 00214 * @param address The address to be set - 7bit or 9bit 00215 * @param stop If true, stop will be generated after the transfer is done 00216 * @param handler The I2C IRQ handler to be set 00217 * @param event Event mask for the transfer. See \ref hal_I2CEvents 00218 * @param hint DMA hint usage 00219 */ 00220 void i2c_transfer_asynch(i2c_t *obj, const void *tx, size_t tx_length, void *rx, size_t rx_length, uint32_t address, uint32_t stop, uint32_t handler, uint32_t event, DMAUsage hint); 00221 00222 /** The asynchronous IRQ handler 00223 * 00224 * @param obj The I2C object which holds the transfer information 00225 * @return Event flags if a transfer termination condition was met, otherwise return 0. 00226 */ 00227 uint32_t i2c_irq_handler_asynch(i2c_t *obj); 00228 00229 /** Attempts to determine if the I2C peripheral is already in use 00230 * 00231 * @param obj The I2C object 00232 * @return Non-zero if the I2C module is active or zero if it is not 00233 */ 00234 uint8_t i2c_active(i2c_t *obj); 00235 00236 /** Abort asynchronous transfer 00237 * 00238 * This function does not perform any check - that should happen in upper layers. 00239 * @param obj The I2C object 00240 */ 00241 void i2c_abort_asynch(i2c_t *obj); 00242 00243 #endif 00244 00245 /**@}*/ 00246 00247 #ifdef __cplusplus 00248 } 00249 #endif 00250 00251 #endif 00252 00253 #endif 00254 00255 /** @}*/
Generated on Tue Jul 12 2022 20:41:15 by
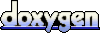