mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
Functions | |
void | set_time (time_t t) |
Implementation of the C time.h functions. | |
void | attach_rtc (time_t(*read_rtc)(void), void(*write_rtc)(time_t), void(*init_rtc)(void), int(*isenabled_rtc)(void)) |
Attach an external RTC to be used for the C time functions. |
Function Documentation
void attach_rtc | ( | time_t(*)(void) | read_rtc, |
void(*)(time_t) | write_rtc, | ||
void(*)(void) | init_rtc, | ||
int(*)(void) | isenabled_rtc | ||
) |
Attach an external RTC to be used for the C time functions.
- Note:
- Synchronization level: Thread safe
- Parameters:
-
read_rtc pointer to function which returns current UNIX timestamp write_rtc pointer to function which sets current UNIX timestamp, can be NULL init_rtc pointer to function which initializes RTC, can be NULL isenabled_rtc pointer to function which returns if the RTC is enabled, can be NULL
Definition at line 119 of file mbed_rtc_time.cpp.
void set_time | ( | time_t | t ) |
Implementation of the C time.h functions.
Provides mechanisms to set and read the current time, based on the microcontroller Real-Time Clock (RTC), plus some standard C manipulation and formatting functions.
Example:
#include "mbed.h" int main() { set_time(1256729737); // Set RTC time to Wed, 28 Oct 2009 11:35:37 while (true) { time_t seconds = time(NULL); printf("Time as seconds since January 1, 1970 = %u\n", (unsigned int)seconds); printf("Time as a basic string = %s", ctime(&seconds)); char buffer[32]; strftime(buffer, 32, "%I:%M %p\n", localtime(&seconds)); printf("Time as a custom formatted string = %s", buffer); wait(1); } }
Set the current time
Initializes and sets the time of the microcontroller Real-Time Clock (RTC) to the time represented by the number of seconds since January 1, 1970 (the UNIX timestamp).
- Parameters:
-
t Number of seconds since January 1, 1970 (the UNIX timestamp)
- Note:
- Synchronization level: Thread safe
Example:
#include "mbed.h" int main() { set_time(1256729737); // Set time to Wed, 28 Oct 2009 11:35:37 }
Definition at line 107 of file mbed_rtc_time.cpp.
Generated on Tue Jul 12 2022 20:41:16 by
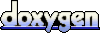