mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
critical section function
[Platform]
Data Structures | |
struct | core_util_atomic_flag |
A lock-free, primitive atomic flag. More... | |
Typedefs | |
typedef struct core_util_atomic_flag | core_util_atomic_flag |
A lock-free, primitive atomic flag. | |
typedef struct core_util_atomic_flag | core_util_atomic_flag |
A lock-free, primitive atomic flag. | |
Functions | |
bool | core_util_are_interrupts_enabled (void) |
Determine the current interrupts enabled state. | |
bool | core_util_is_isr_active (void) |
Determine if this code is executing from an interrupt. | |
void | core_util_critical_section_enter (void) |
Mark the start of a critical section. | |
void | core_util_critical_section_exit (void) |
Mark the end of a critical section. | |
bool | core_util_in_critical_section (void) |
Determine if we are currently in a critical section. | |
bool | core_util_atomic_flag_test_and_set (volatile core_util_atomic_flag *flagPtr) |
Atomic test and set. | |
void | core_util_atomic_flag_clear (volatile core_util_atomic_flag *flagPtr) |
Atomic clear. | |
bool | core_util_atomic_cas_u8 (volatile uint8_t *ptr, uint8_t *expectedCurrentValue, uint8_t desiredValue) |
Atomic compare and set. | |
bool | core_util_atomic_cas_u16 (volatile uint16_t *ptr, uint16_t *expectedCurrentValue, uint16_t desiredValue) |
Atomic compare and set. | |
bool | core_util_atomic_cas_u32 (volatile uint32_t *ptr, uint32_t *expectedCurrentValue, uint32_t desiredValue) |
Atomic compare and set. | |
bool | core_util_atomic_cas_ptr (void *volatile *ptr, void **expectedCurrentValue, void *desiredValue) |
Atomic compare and set. | |
uint8_t | core_util_atomic_incr_u8 (volatile uint8_t *valuePtr, uint8_t delta) |
Atomic increment. | |
uint16_t | core_util_atomic_incr_u16 (volatile uint16_t *valuePtr, uint16_t delta) |
Atomic increment. | |
uint32_t | core_util_atomic_incr_u32 (volatile uint32_t *valuePtr, uint32_t delta) |
Atomic increment. | |
void * | core_util_atomic_incr_ptr (void *volatile *valuePtr, ptrdiff_t delta) |
Atomic increment. | |
uint8_t | core_util_atomic_decr_u8 (volatile uint8_t *valuePtr, uint8_t delta) |
Atomic decrement. | |
uint16_t | core_util_atomic_decr_u16 (volatile uint16_t *valuePtr, uint16_t delta) |
Atomic decrement. | |
uint32_t | core_util_atomic_decr_u32 (volatile uint32_t *valuePtr, uint32_t delta) |
Atomic decrement. | |
void * | core_util_atomic_decr_ptr (void *volatile *valuePtr, ptrdiff_t delta) |
Atomic decrement. |
Typedef Documentation
typedef struct core_util_atomic_flag core_util_atomic_flag |
A lock-free, primitive atomic flag.
Emulate C11's atomic_flag. The flag is initially in an indeterminate state unless explicitly initialized with CORE_UTIL_ATOMIC_FLAG_INIT.
typedef struct core_util_atomic_flag core_util_atomic_flag |
A lock-free, primitive atomic flag.
Emulate C11's atomic_flag. The flag is initially in an indeterminate state unless explicitly initialized with CORE_UTIL_ATOMIC_FLAG_INIT.
Function Documentation
bool core_util_are_interrupts_enabled | ( | void | ) |
Determine the current interrupts enabled state.
This function can be called to determine whether or not interrupts are currently enabled.
- Note:
- NOTE: This function works for both cortex-A and cortex-M, although the underlying implementation differs.
- Returns:
- true if interrupts are enabled, false otherwise
Definition at line 48 of file mbed_critical.c.
bool core_util_atomic_cas_ptr | ( | void *volatile * | ptr, |
void ** | expectedCurrentValue, | ||
void * | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value.
- Note:
- : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value.
- Parameters:
-
[in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
- Note:
- : In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is instead updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
- Note:
- : This corresponds to the C11 "atomic_compare_exchange_strong" - it always succeeds if the current value is expected, as per the pseudocode above; it will not spuriously fail as "atomic_compare_exchange_weak" may.
Definition at line 347 of file mbed_critical.c.
bool core_util_atomic_cas_u16 | ( | volatile uint16_t * | ptr, |
uint16_t * | expectedCurrentValue, | ||
uint16_t | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value.
- Note:
- : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value.
- Parameters:
-
[in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
- Note:
- : In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is instead updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
- Note:
- : This corresponds to the C11 "atomic_compare_exchange_strong" - it always succeeds if the current value is expected, as per the pseudocode above; it will not spuriously fail as "atomic_compare_exchange_weak" may.
Definition at line 247 of file mbed_critical.c.
bool core_util_atomic_cas_u32 | ( | volatile uint32_t * | ptr, |
uint32_t * | expectedCurrentValue, | ||
uint32_t | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value.
- Note:
- : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value.
- Parameters:
-
[in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
- Note:
- : In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is instead updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a
- Note:
- : This corresponds to the C11 "atomic_compare_exchange_strong" - it always succeeds if the current value is expected, as per the pseudocode above; it will not spuriously fail as "atomic_compare_exchange_weak" may. }
Definition at line 265 of file mbed_critical.c.
bool core_util_atomic_cas_u8 | ( | volatile uint8_t * | ptr, |
uint8_t * | expectedCurrentValue, | ||
uint8_t | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value.
- Note:
- : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value.
- Parameters:
-
[in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
- Note:
- : In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is instead updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
- Note:
- : This corresponds to the C11 "atomic_compare_exchange_strong" - it always succeeds if the current value is expected, as per the pseudocode above; it will not spuriously fail as "atomic_compare_exchange_weak" may.
Definition at line 230 of file mbed_critical.c.
void * core_util_atomic_decr_ptr | ( | void *volatile * | valuePtr, |
ptrdiff_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented in bytes.
- Returns:
- The new decremented value.
- Note:
- The type of the pointer argument is not taken into account and the pointer is decremented by bytes
Definition at line 360 of file mbed_critical.c.
uint16_t core_util_atomic_decr_u16 | ( | volatile uint16_t * | valuePtr, |
uint16_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented.
- Returns:
- The new decremented value.
Definition at line 324 of file mbed_critical.c.
uint32_t core_util_atomic_decr_u32 | ( | volatile uint32_t * | valuePtr, |
uint32_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented.
- Returns:
- The new decremented value.
Definition at line 334 of file mbed_critical.c.
uint8_t core_util_atomic_decr_u8 | ( | volatile uint8_t * | valuePtr, |
uint8_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented.
- Returns:
- The new decremented value.
Definition at line 314 of file mbed_critical.c.
void core_util_atomic_flag_clear | ( | volatile core_util_atomic_flag * | flagPtr ) |
Atomic clear.
- Parameters:
-
flagPtr Target flag being cleared.
Definition at line 103 of file mbed_critical.c.
bool core_util_atomic_flag_test_and_set | ( | volatile core_util_atomic_flag * | flagPtr ) |
Atomic test and set.
Atomically tests then sets the flag to true, returning the previous value.
- Parameters:
-
flagPtr Target flag being tested and set.
- Returns:
- The previous value.
Definition at line 221 of file mbed_critical.c.
void * core_util_atomic_incr_ptr | ( | void *volatile * | valuePtr, |
ptrdiff_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented in bytes.
- Returns:
- The new incremented value.
- Note:
- The type of the pointer argument is not taken into account and the pointer is incremented by bytes.
Definition at line 355 of file mbed_critical.c.
uint16_t core_util_atomic_incr_u16 | ( | volatile uint16_t * | valuePtr, |
uint16_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented.
- Returns:
- The new incremented value.
Definition at line 293 of file mbed_critical.c.
uint32_t core_util_atomic_incr_u32 | ( | volatile uint32_t * | valuePtr, |
uint32_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented.
- Returns:
- The new incremented value.
Definition at line 303 of file mbed_critical.c.
uint8_t core_util_atomic_incr_u8 | ( | volatile uint8_t * | valuePtr, |
uint8_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented.
- Returns:
- The new incremented value.
Definition at line 283 of file mbed_critical.c.
void core_util_critical_section_enter | ( | void | ) |
Mark the start of a critical section.
This function should be called to mark the start of a critical section of code.
- Note:
- NOTES: 1) The use of this style of critical section is targetted at C based implementations. 2) These critical sections can be nested. 3) The interrupt enable state on entry to the first critical section (of a nested set, or single section) will be preserved on exit from the section. 4) This implementation will currently only work on code running in privileged mode.
Definition at line 78 of file mbed_critical.c.
void core_util_critical_section_exit | ( | void | ) |
Mark the end of a critical section.
This function should be called to mark the end of a critical section of code.
- Note:
- NOTES: 1) The use of this style of critical section is targetted at C based implementations. 2) These critical sections can be nested. 3) The interrupt enable state on entry to the first critical section (of a nested set, or single section) will be preserved on exit from the section. 4) This implementation will currently only work on code running in privileged mode.
Definition at line 88 of file mbed_critical.c.
bool core_util_in_critical_section | ( | void | ) |
Determine if we are currently in a critical section.
- Returns:
- true if in a critical section, false otherwise.
Definition at line 73 of file mbed_critical.c.
bool core_util_is_isr_active | ( | void | ) |
Determine if this code is executing from an interrupt.
This function can be called to determine if the code is running on interrupt context.
- Note:
- NOTE: This function works for both cortex-A and cortex-M, although the underlying implementation differs.
- Returns:
- true if in an isr, false otherwise
Definition at line 57 of file mbed_critical.c.
Generated on Tue Jul 12 2022 20:41:16 by
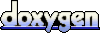