mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
Low level interface to the microsecond ticker of a target. More...
Modules | |
Microsecond Ticker tests | |
Tests to validate the proper implementation of the microsecond ticker. | |
Ticker Hal | |
Low level interface to the ticker of a target. | |
Ticker Tests | |
Tests to validate the proper implementation of a ticker. | |
Functions | |
ticker_irq_handler_type | set_us_ticker_irq_handler (ticker_irq_handler_type ticker_irq_handler) |
Set ticker IRQ handler. | |
const ticker_data_t * | get_us_ticker_data (void) |
Get ticker's data. | |
void | us_ticker_irq_handler (void) |
The wrapper for ticker_irq_handler, to pass us ticker's data. | |
void | us_ticker_init (void) |
Initialize the ticker. | |
void | us_ticker_free (void) |
Deinitialize the us ticker. | |
uint32_t | us_ticker_read (void) |
Read the current counter. | |
void | us_ticker_set_interrupt (timestamp_t timestamp) |
Set interrupt for specified timestamp. | |
void | us_ticker_disable_interrupt (void) |
Disable us ticker interrupt. | |
void | us_ticker_clear_interrupt (void) |
Clear us ticker interrupt. | |
void | us_ticker_fire_interrupt (void) |
Set pending interrupt that should be fired right away. | |
const ticker_info_t * | us_ticker_get_info (void) |
Get frequency and counter bits of this ticker. |
Detailed Description
Low level interface to the microsecond ticker of a target.
# Defined behavior * Has a reported frequency between 250KHz and 8MHz - Verified by test us_ticker_info_test * Has a counter that is at least 16 bits wide - Verified by test us_ticker_info_test * All behavior defined by the ticker specification
# Undefined behavior * See the ticker specification
- See also:
- Microsecond Ticker tests
Function Documentation
const ticker_data_t * get_us_ticker_data | ( | void | ) |
Get ticker's data.
- Returns:
- The microsecond ticker data
Definition at line 39 of file mbed_us_ticker_api.c.
ticker_irq_handler_type set_us_ticker_irq_handler | ( | ticker_irq_handler_type | ticker_irq_handler ) |
Set ticker IRQ handler.
- Parameters:
-
ticker_irq_handler IRQ handler to be connected
- Returns:
- previous ticker IRQ handler
- Note:
- by default IRQ handler is set to ticker_irq_handler
- this function is primarily for testing purposes and it's not required part of HAL implementation
Definition at line 44 of file mbed_us_ticker_api.c.
void us_ticker_clear_interrupt | ( | void | ) |
Clear us ticker interrupt.
Pseudo Code:
void us_ticker_clear_interrupt(void) { // Write to the ICR (interrupt clear register) of the TIMER TIMER_ICR = TIMER_ICR_COMPARE_Msk; }
void us_ticker_disable_interrupt | ( | void | ) |
Disable us ticker interrupt.
Pseudo Code:
void us_ticker_disable_interrupt(void) { // Disable the compare interrupt TIMER_CTRL &= ~TIMER_CTRL_COMPARE_ENABLE_Msk; }
void us_ticker_fire_interrupt | ( | void | ) |
Set pending interrupt that should be fired right away.
The ticker should be initialized prior calling this function.
Pseudo Code:
void us_ticker_fire_interrupt(void) { NVIC_SetPendingIRQ(TIMER_IRQn); }
void us_ticker_free | ( | void | ) |
Deinitialize the us ticker.
Powerdown the us ticker in preparation for sleep, powerdown, or reset.
After this function is called, no other ticker functions should be called except us_ticker_init(), calling any function other than init is undefined.
- Note:
- This function stops the ticker from counting.
Pseudo Code:
uint32_t us_ticker_free() { // Disable timer TIMER_CTRL &= ~TIMER_CTRL_ENABLE_Msk; // Disable the compare interrupt TIMER_CTRL &= ~TIMER_CTRL_COMPARE_ENABLE_Msk; // Disable timer interrupt NVIC_DisableIRQ(TIMER_IRQn); // Disable clock gate so processor cannot read TIMER registers POWER_CTRL &= ~POWER_CTRL_TIMER_Msk; }
const ticker_info_t * us_ticker_get_info | ( | void | ) |
Get frequency and counter bits of this ticker.
Pseudo Code:
const ticker_info_t* us_ticker_get_info() { static const ticker_info_t info = { 1000000, // 1 MHz 32 // 32 bit counter }; return &info; }
void us_ticker_init | ( | void | ) |
Initialize the ticker.
Initialize or re-initialize the ticker. This resets all the clocking and prescaler registers, along with disabling the compare interrupt.
- Note:
- Initialization properties tested by ticker_init_test
Pseudo Code:
void us_ticker_init() { // Enable clock gate so processor can read TIMER registers POWER_CTRL |= POWER_CTRL_TIMER_Msk; // Disable the timer and ensure it is powered down TIMER_CTRL &= ~(TIMER_CTRL_ENABLE_Msk | TIMER_CTRL_COMPARE_ENABLE_Msk); // Configure divisors uint32_t prescale = SystemCoreClock / 1000000; TIMER_PRESCALE = prescale - 1; TIMER_CTRL |= TIMER_CTRL_ENABLE_Msk; // Install the interrupt handler NVIC_SetVector(TIMER_IRQn, (uint32_t)us_ticker_irq_handler); NVIC_EnableIRQ(TIMER_IRQn); }
void us_ticker_irq_handler | ( | void | ) |
The wrapper for ticker_irq_handler, to pass us ticker's data.
Definition at line 53 of file mbed_us_ticker_api.c.
uint32_t us_ticker_read | ( | void | ) |
Read the current counter.
Read the current counter value without performing frequency conversions. If no rollover has occurred, the seconds passed since us_ticker_init() was called can be found by dividing the ticks returned by this function by the frequency returned by us_ticker_get_info.
- Returns:
- The current timer's counter value in ticks
Pseudo Code:
uint32_t us_ticker_read() { return TIMER_COUNT; }
void us_ticker_set_interrupt | ( | timestamp_t | timestamp ) |
Set interrupt for specified timestamp.
- Parameters:
-
timestamp The time in ticks to be set
- Note:
- no special handling needs to be done for times in the past as the common timer code will detect this and call us_ticker_fire_interrupt() if this is the case
- calling this function with timestamp of more than the supported number of bits returned by us_ticker_get_info results in undefined behavior.
Pseudo Code:
void us_ticker_set_interrupt(timestamp_t timestamp) { TIMER_COMPARE = timestamp; TIMER_CTRL |= TIMER_CTRL_COMPARE_ENABLE_Msk; }
Generated on Tue Jul 12 2022 20:41:16 by
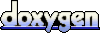