mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
DigitalOut.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef MBED_DIGITALOUT_H 00018 #define MBED_DIGITALOUT_H 00019 00020 #include "platform/platform.h" 00021 #include "hal/gpio_api.h" 00022 #include "platform/mbed_critical.h" 00023 00024 namespace mbed { 00025 /** \addtogroup drivers */ 00026 00027 /** A digital output, used for setting the state of a pin 00028 * 00029 * @note Synchronization level: Interrupt safe 00030 * 00031 * Example: 00032 * @code 00033 * // Toggle a LED 00034 * #include "mbed.h" 00035 * 00036 * DigitalOut led(LED1); 00037 * 00038 * int main() { 00039 * while(1) { 00040 * led = !led; 00041 * wait(0.2); 00042 * } 00043 * } 00044 * @endcode 00045 * @ingroup drivers 00046 */ 00047 class DigitalOut { 00048 00049 public: 00050 /** Create a DigitalOut connected to the specified pin 00051 * 00052 * @param pin DigitalOut pin to connect to 00053 */ 00054 DigitalOut(PinName pin) : gpio() 00055 { 00056 // No lock needed in the constructor 00057 gpio_init_out(&gpio, pin); 00058 } 00059 00060 /** Create a DigitalOut connected to the specified pin 00061 * 00062 * @param pin DigitalOut pin to connect to 00063 * @param value the initial pin value 00064 */ 00065 DigitalOut(PinName pin, int value) : gpio() 00066 { 00067 // No lock needed in the constructor 00068 gpio_init_out_ex(&gpio, pin, value); 00069 } 00070 00071 /** Set the output, specified as 0 or 1 (int) 00072 * 00073 * @param value An integer specifying the pin output value, 00074 * 0 for logical 0, 1 (or any other non-zero value) for logical 1 00075 */ 00076 void write(int value) 00077 { 00078 // Thread safe / atomic HAL call 00079 gpio_write(&gpio, value); 00080 } 00081 00082 /** Return the output setting, represented as 0 or 1 (int) 00083 * 00084 * @returns 00085 * an integer representing the output setting of the pin, 00086 * 0 for logical 0, 1 for logical 1 00087 */ 00088 int read() 00089 { 00090 // Thread safe / atomic HAL call 00091 return gpio_read(&gpio); 00092 } 00093 00094 /** Return the output setting, represented as 0 or 1 (int) 00095 * 00096 * @returns 00097 * Non zero value if pin is connected to uc GPIO 00098 * 0 if gpio object was initialized with NC 00099 */ 00100 int is_connected() 00101 { 00102 // Thread safe / atomic HAL call 00103 return gpio_is_connected(&gpio); 00104 } 00105 00106 /** A shorthand for write() 00107 * \sa DigitalOut::write() 00108 * @code 00109 * DigitalIn button(BUTTON1); 00110 * DigitalOut led(LED1); 00111 * led = button; // Equivalent to led.write(button.read()) 00112 * @endcode 00113 */ 00114 DigitalOut &operator= (int value) 00115 { 00116 // Underlying write is thread safe 00117 write(value); 00118 return *this; 00119 } 00120 00121 /** A shorthand for write() using the assignment operator which copies the 00122 * state from the DigitalOut argument. 00123 * \sa DigitalOut::write() 00124 */ 00125 DigitalOut &operator= (DigitalOut &rhs) 00126 { 00127 core_util_critical_section_enter(); 00128 write(rhs.read()); 00129 core_util_critical_section_exit(); 00130 return *this; 00131 } 00132 00133 /** A shorthand for read() 00134 * \sa DigitalOut::read() 00135 * @code 00136 * DigitalIn button(BUTTON1); 00137 * DigitalOut led(LED1); 00138 * led = button; // Equivalent to led.write(button.read()) 00139 * @endcode 00140 */ 00141 operator int() 00142 { 00143 // Underlying call is thread safe 00144 return read(); 00145 } 00146 00147 protected: 00148 #if !defined(DOXYGEN_ONLY) 00149 gpio_t gpio; 00150 #endif //!defined(DOXYGEN_ONLY) 00151 }; 00152 00153 } // namespace mbed 00154 00155 #endif
Generated on Tue Jul 12 2022 20:41:14 by
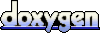