mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
FileSystemHandle Class Reference
[FileSystemHandle functions]
A filesystem-like object is one that can be used to open file-like objects though it by fopen("/name/filename", mode) More...
#include <FileSystemHandle.h>
Inherits NonCopyable< FileSystemHandle >, and NonCopyable< FileSystemHandle >.
Inherited by FileSystemLike, and FileSystemLike.
Public Member Functions | |
virtual | ~FileSystemHandle () |
FileSystemHandle lifetime. | |
virtual int | open (FileHandle **file, const char *filename, int flags)=0 |
Open a file on the filesystem. | |
virtual int | open (DirHandle **dir, const char *path) |
Open a directory on the filesystem. | |
virtual int | remove (const char *path) |
Remove a file from the filesystem. | |
virtual int | rename (const char *path, const char *newpath) |
Rename a file in the filesystem. | |
virtual int | stat (const char *path, struct stat *st) |
Store information about the file in a stat structure. | |
virtual int | mkdir (const char *path, mode_t mode) |
Create a directory in the filesystem. | |
virtual int | statvfs (const char *path, struct statvfs *buf) |
Store information about the mounted filesystem in a statvfs structure. | |
virtual | ~FileSystemHandle () |
FileSystemHandle lifetime. | |
virtual int | open (FileHandle **file, const char *filename, int flags)=0 |
Open a file on the filesystem. | |
virtual int | open (DirHandle **dir, const char *path) |
Open a directory on the filesystem. | |
virtual int | remove (const char *path) |
Remove a file from the filesystem. | |
virtual int | rename (const char *path, const char *newpath) |
Rename a file in the filesystem. | |
virtual int | stat (const char *path, struct stat *st) |
Store information about the file in a stat structure. | |
virtual int | mkdir (const char *path, mode_t mode) |
Create a directory in the filesystem. | |
virtual int | statvfs (const char *path, struct statvfs *buf) |
Store information about the mounted filesystem in a statvfs structure. | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
A filesystem-like object is one that can be used to open file-like objects though it by fopen("/name/filename", mode)
Implementations must define at least open (the default definitions of the rest of the functions just return error values).
- Note:
- Synchronization level: Set by subclass
Definition at line 44 of file cmsis/BUILD/mbed/platform/FileSystemHandle.h.
Constructor & Destructor Documentation
virtual ~FileSystemHandle | ( | ) | [virtual] |
FileSystemHandle lifetime.
Definition at line 48 of file cmsis/BUILD/mbed/platform/FileSystemHandle.h.
virtual ~FileSystemHandle | ( | ) | [virtual] |
FileSystemHandle lifetime.
Definition at line 48 of file platform/FileSystemHandle.h.
Member Function Documentation
int mkdir | ( | const char * | path, |
mode_t | mode | ||
) | [virtual] |
Create a directory in the filesystem.
- Parameters:
-
path The name of the directory to create. mode The permissions with which to create the directory
- Returns:
- 0 on success, negative error code on failure
Definition at line 43 of file FileSystemHandle.cpp.
virtual int mkdir | ( | const char * | path, |
mode_t | mode | ||
) | [virtual] |
Create a directory in the filesystem.
- Parameters:
-
path The name of the directory to create. mode The permissions with which to create the directory
- Returns:
- 0 on success, negative error code on failure
int open | ( | mbed::DirHandle ** | dir, |
const char * | path | ||
) | [virtual] |
Open a directory on the filesystem.
- Parameters:
-
dir Destination for the handle to the directory path Name of the directory to open
- Returns:
- 0 on success, negative error code on failure
Reimplemented in LocalFileSystem, and LocalFileSystem.
Definition at line 23 of file FileSystemHandle.cpp.
virtual int open | ( | FileHandle ** | file, |
const char * | filename, | ||
int | flags | ||
) | [pure virtual] |
Open a file on the filesystem.
- Parameters:
-
file Destination for the handle to a newly created file filename The name of the file to open flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND
- Returns:
- 0 on success, negative error code on failure
Implemented in LocalFileSystem, and LocalFileSystem.
virtual int open | ( | DirHandle ** | dir, |
const char * | path | ||
) | [virtual] |
Open a directory on the filesystem.
- Parameters:
-
dir Destination for the handle to the directory path Name of the directory to open
- Returns:
- 0 on success, negative error code on failure
Reimplemented in LocalFileSystem, and LocalFileSystem.
virtual int open | ( | FileHandle ** | file, |
const char * | filename, | ||
int | flags | ||
) | [pure virtual] |
Open a file on the filesystem.
- Parameters:
-
file Destination for the handle to a newly created file filename The name of the file to open flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND
- Returns:
- 0 on success, negative error code on failure
Implemented in LocalFileSystem, and LocalFileSystem.
virtual int remove | ( | const char * | path ) | [virtual] |
Remove a file from the filesystem.
- Parameters:
-
path The name of the file to remove.
- Returns:
- 0 on success, negative error code on failure
Reimplemented in LocalFileSystem, and LocalFileSystem.
int remove | ( | const char * | path ) | [virtual] |
Remove a file from the filesystem.
- Parameters:
-
path The name of the file to remove.
- Returns:
- 0 on success, negative error code on failure
Reimplemented in LocalFileSystem, and LocalFileSystem.
Definition at line 28 of file FileSystemHandle.cpp.
int rename | ( | const char * | path, |
const char * | newpath | ||
) | [virtual] |
Rename a file in the filesystem.
- Parameters:
-
path The name of the file to rename. newpath The name to rename it to
- Returns:
- 0 on success, negative error code on failure
Definition at line 33 of file FileSystemHandle.cpp.
virtual int rename | ( | const char * | path, |
const char * | newpath | ||
) | [virtual] |
Rename a file in the filesystem.
- Parameters:
-
path The name of the file to rename. newpath The name to rename it to
- Returns:
- 0 on success, negative error code on failure
virtual int stat | ( | const char * | path, |
struct stat * | st | ||
) | [virtual] |
Store information about the file in a stat structure.
- Parameters:
-
path The name of the file to find information about st The stat buffer to write to
- Returns:
- 0 on success, negative error code on failure
int stat | ( | const char * | path, |
struct stat * | st | ||
) | [virtual] |
Store information about the file in a stat structure.
- Parameters:
-
path The name of the file to find information about st The stat buffer to write to
- Returns:
- 0 on success, negative error code on failure
Definition at line 38 of file FileSystemHandle.cpp.
virtual int statvfs | ( | const char * | path, |
struct statvfs * | buf | ||
) | [virtual] |
Store information about the mounted filesystem in a statvfs structure.
- Parameters:
-
path The name of the file to find information about buf The stat buffer to write to
- Returns:
- 0 on success, negative error code on failure
int statvfs | ( | const char * | path, |
struct statvfs * | buf | ||
) | [virtual] |
Store information about the mounted filesystem in a statvfs structure.
- Parameters:
-
path The name of the file to find information about buf The stat buffer to write to
- Returns:
- 0 on success, negative error code on failure
Definition at line 48 of file FileSystemHandle.cpp.
Generated on Tue Jul 12 2022 20:41:18 by
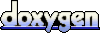