mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
CallChain Class Reference
[CallChain class]
Group one or more functions in an instance of a CallChain, then call them in sequence using CallChain::call(). More...
#include <CallChain.h>
Inherits NonCopyable< CallChain >, and NonCopyable< CallChain >.
Public Member Functions | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") CallChain(int size | |
Create an empty chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") virtual ~CallChain() | |
Create an empty chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") pFunctionPointer_t add(Callback< void()> func) | |
Add a function at the end of the chain. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The add function does not support cv-qualifiers. Replaced by ""add(callback(obj, method)).") pFunctionPointer_t add(T *obj | |
Add a function at the end of the chain. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The add_front function does not support cv-qualifiers. Replaced by ""add_front(callback(obj, method)).") pFunctionPointer_t add_front(T *obj | |
Add a function at the beginning of the chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") pFunctionPointer_t get(int i) const | |
Get a function object from the chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") int find(pFunctionPointer_t f) const | |
Look for a function object in the call chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") void clear() | |
Clear the call chain (remove all functions in the chain). | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") bool remove(pFunctionPointer_t f) | |
Remove a function object from the chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") void call() | |
Call all the functions in the chain in sequence. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") void operator()(void) | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") pFunctionPointer_t operator[](int i) const | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") CallChain(int size | |
Create an empty chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") virtual ~CallChain() | |
Create an empty chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") pFunctionPointer_t add(Callback< void()> func) | |
Add a function at the end of the chain. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The add function does not support cv-qualifiers. Replaced by ""add(callback(obj, method)).") pFunctionPointer_t add(T *obj | |
Add a function at the end of the chain. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The add_front function does not support cv-qualifiers. Replaced by ""add_front(callback(obj, method)).") pFunctionPointer_t add_front(T *obj | |
Add a function at the beginning of the chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") pFunctionPointer_t get(int i) const | |
Get a function object from the chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") int find(pFunctionPointer_t f) const | |
Look for a function object in the call chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") void clear() | |
Clear the call chain (remove all functions in the chain). | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") bool remove(pFunctionPointer_t f) | |
Remove a function object from the chain. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") void call() | |
Call all the functions in the chain in sequence. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") void operator()(void) | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","This class is not part of the ""public API of mbed-os and is being removed in the future.") pFunctionPointer_t operator[](int i) const | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
Group one or more functions in an instance of a CallChain, then call them in sequence using CallChain::call().
Used mostly by the interrupt chaining code, but can be used for other purposes.
- Note:
- Synchronization level: Not protected
Example:
#include "mbed.h" CallChain chain; void first(void) { printf("'first' function.\n"); } void second(void) { printf("'second' function.\n"); } class Test { public: void f(void) { printf("A::f (class member).\n"); } }; int main() { Test test; chain.add(second); chain.add_front(first); chain.add(&test, &Test::f); chain.call(); }
Used mostly by the interrupt chaining code, but can be used for other purposes.
- Note:
- Synchronization level: Not protected
Example:
#include "mbed.h" CallChain chain; void first(void) { printf("'first' function.\n"); } void second(void) { printf("'second' function.\n"); } class Test { public: void f(void) { printf("A::f (class member).\n"); } }; int main() { Test test; chain.add(second); chain.add_front(first); chain.add(&test, &Test::f); chain.call(); }
Definition at line 76 of file cmsis/BUILD/mbed/platform/CallChain.h.
Member Function Documentation
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Create an empty chain.
- Parameters:
-
size (optional) Initial size of the chain
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) | const |
Definition at line 247 of file platform/CallChain.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Definition at line 235 of file platform/CallChain.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Call all the functions in the chain in sequence.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Remove a function object from the chain.
- f the function object to remove
- Returns:
- true if the function object was found and removed, false otherwise.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Clear the call chain (remove all functions in the chain).
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) | const |
Look for a function object in the call chain.
- Parameters:
-
f the function object to search
- Returns:
- The index of the function object if found, -1 otherwise.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) | const |
Get a function object from the chain.
- Parameters:
-
i function object index
- Returns:
- The function object at position 'i' in the chain
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The add_front function does not support cv-qualifiers. Replaced by ""add_front(callback(obj, method))." | |||
) |
Add a function at the beginning of the chain.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called
- Returns:
- The function object created for the object and method pointers
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The add function does not support cv-qualifiers. Replaced by ""add(callback(obj, method))." | |||
) |
Add a function at the end of the chain.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called
- Returns:
- The function object created for 'obj' and 'method'
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Add a function at the end of the chain.
- Parameters:
-
func A pointer to a void function
- Returns:
- The function object created for 'func'
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Create an empty chain.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Create an empty chain.
- Parameters:
-
size (optional) Initial size of the chain
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) | const |
Definition at line 247 of file cmsis/BUILD/mbed/platform/CallChain.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Definition at line 235 of file cmsis/BUILD/mbed/platform/CallChain.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Call all the functions in the chain in sequence.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Remove a function object from the chain.
- f the function object to remove
- Returns:
- true if the function object was found and removed, false otherwise.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Clear the call chain (remove all functions in the chain).
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) | const |
Look for a function object in the call chain.
- Parameters:
-
f the function object to search
- Returns:
- The index of the function object if found, -1 otherwise.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) | const |
Get a function object from the chain.
- Parameters:
-
i function object index
- Returns:
- The function object at position 'i' in the chain
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The add_front function does not support cv-qualifiers. Replaced by ""add_front(callback(obj, method))." | |||
) |
Add a function at the beginning of the chain.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called
- Returns:
- The function object created for the object and method pointers
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The add function does not support cv-qualifiers. Replaced by ""add(callback(obj, method))." | |||
) |
Add a function at the end of the chain.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called
- Returns:
- The function object created for 'obj' and 'method'
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Add a function at the end of the chain.
- Parameters:
-
func A pointer to a void function
- Returns:
- The function object created for 'func'
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"This class is not part of the ""public API of mbed-os and is being removed in the future." | |||
) |
Create an empty chain.
Generated on Tue Jul 12 2022 20:41:18 by
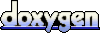