mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
RawSerial.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include "drivers/RawSerial.h" 00018 #include "platform/mbed_wait_api.h" 00019 #include <stdio.h> 00020 #include <cstdarg> 00021 00022 00023 #if DEVICE_SERIAL 00024 00025 #define STRING_STACK_LIMIT 120 00026 00027 namespace mbed { 00028 00029 RawSerial::RawSerial(PinName tx, PinName rx, int baud) : SerialBase(tx, rx, baud) 00030 { 00031 // No lock needed in the constructor 00032 } 00033 00034 int RawSerial::getc() 00035 { 00036 lock(); 00037 int ret = _base_getc(); 00038 unlock(); 00039 return ret; 00040 } 00041 00042 int RawSerial::putc(int c) 00043 { 00044 lock(); 00045 int ret = _base_putc(c); 00046 unlock(); 00047 return ret; 00048 } 00049 00050 int RawSerial::puts(const char *str) 00051 { 00052 lock(); 00053 while (*str) { 00054 putc(*str ++); 00055 } 00056 unlock(); 00057 return 0; 00058 } 00059 00060 // Experimental support for printf in RawSerial. No Stream inheritance 00061 // means we can't call printf() directly, so we use sprintf() instead. 00062 // We only call malloc() for the sprintf() buffer if the buffer 00063 // length is above a certain threshold, otherwise we use just the stack. 00064 int RawSerial::printf(const char *format, ...) 00065 { 00066 lock(); 00067 std::va_list arg; 00068 va_start(arg, format); 00069 // ARMCC microlib does not properly handle a size of 0. 00070 // As a workaround supply a dummy buffer with a size of 1. 00071 char dummy_buf[1]; 00072 int len = vsnprintf(dummy_buf, sizeof(dummy_buf), format, arg); 00073 if (len < STRING_STACK_LIMIT) { 00074 char temp[STRING_STACK_LIMIT]; 00075 vsprintf(temp, format, arg); 00076 puts(temp); 00077 } else { 00078 char *temp = new char[len + 1]; 00079 vsprintf(temp, format, arg); 00080 puts(temp); 00081 delete[] temp; 00082 } 00083 va_end(arg); 00084 unlock(); 00085 return len; 00086 } 00087 00088 /** Acquire exclusive access to this serial port 00089 */ 00090 void RawSerial::lock() 00091 { 00092 // No lock used - external synchronization required 00093 } 00094 00095 /** Release exclusive access to this serial port 00096 */ 00097 void RawSerial::unlock() 00098 { 00099 // No lock used - external synchronization required 00100 } 00101 00102 } // namespace mbed 00103 00104 #endif
Generated on Tue Jul 12 2022 20:41:15 by
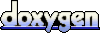