
Basic example showing the CMSIS-RTOS Semaphore API
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "cmsis_os.h" 00003 00004 osSemaphoreId two_slots; 00005 osSemaphoreDef(two_slots); 00006 00007 void test_thread(void const *name) { 00008 while (true) { 00009 osSemaphoreWait(two_slots, osWaitForever); 00010 printf("%s\n\r", (const char*)name); 00011 osDelay(1000); 00012 osSemaphoreRelease(two_slots); 00013 } 00014 } 00015 00016 void t2(void const *argument) {test_thread("Th 2");} 00017 osThreadDef(t2, osPriorityNormal, DEFAULT_STACK_SIZE); 00018 00019 void t3(void const *argument) {test_thread("Th 3");} 00020 osThreadDef(t3, osPriorityNormal, DEFAULT_STACK_SIZE); 00021 00022 int main (void) { 00023 two_slots = osSemaphoreCreate(osSemaphore(two_slots), 2); 00024 00025 osThreadCreate(osThread(t2), NULL); 00026 osThreadCreate(osThread(t3), NULL); 00027 00028 test_thread((void *)"Th 1"); 00029 }
Generated on Tue Jul 12 2022 11:40:13 by
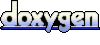