
Basic example showing the CMSIS-RTOS mail API
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "cmsis_os.h" 00003 00004 typedef struct { 00005 float voltage; /* AD result of measured voltage */ 00006 float current; /* AD result of measured current */ 00007 uint32_t counter; /* A counter value */ 00008 } mail_t; 00009 00010 osMailQDef(mail_box, 16, mail_t); 00011 osMailQId mail_box; 00012 00013 void send_thread (void const *args) { 00014 uint32_t i = 0; 00015 while (true) { 00016 i++; // fake data update 00017 mail_t *mail = (mail_t*)osMailAlloc(mail_box, osWaitForever); 00018 mail->voltage = (i * 0.1) * 33; 00019 mail->current = (i * 0.1) * 11; 00020 mail->counter = i; 00021 osMailPut(mail_box, mail); 00022 osDelay(1000); 00023 } 00024 } 00025 00026 osThreadDef(send_thread, osPriorityNormal, DEFAULT_STACK_SIZE); 00027 00028 int main (void) { 00029 mail_box = osMailCreate(osMailQ(mail_box), NULL); 00030 osThreadCreate(osThread(send_thread), NULL); 00031 00032 while (true) { 00033 osEvent evt = osMailGet(mail_box, osWaitForever); 00034 if (evt.status == osEventMail) { 00035 mail_t *mail = (mail_t*)evt.value.p; 00036 printf("\nVoltage: %.2f V\n\r" , mail->voltage); 00037 printf("Current: %.2f A\n\r" , mail->current); 00038 printf("Number of cycles: %u\n\r", mail->counter); 00039 00040 osMailFree(mail_box, mail); 00041 } 00042 } 00043 }
Generated on Tue Jul 12 2022 11:40:16 by
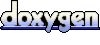