u-blox USB modems (GSM and CDMA)
Dependencies: CellularUSBModem
Dependents: C027_CANInterfaceComm C027_ModemTransparentUSBCDC_revb UbloxModemHTTPClientTest C027_HTTPClientTest ... more
UbloxGSMModemInitializer.cpp
00001 /* Copyright (c) 2010-2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "UbloxGSMModemInitializer.h" 00020 #include "core/dbg.h" 00021 00022 #define __DEBUG__ 0 00023 #ifndef __MODULE__ 00024 #define __MODULE__ "UbloxGSMModemInitializer.cpp" 00025 #endif 00026 00027 //----------------------------------------------------------------------- 00028 // mamm, u-blox Modem 00029 //----------------------------------------------------------------------- 00030 00031 UbloxGSMModemInitializer::UbloxGSMModemInitializer(USBHost* pHost) : WANDongleInitializer(pHost) 00032 { 00033 00034 } 00035 00036 uint16_t UbloxGSMModemInitializer::getMSDVid() { return 0x1546; } 00037 uint16_t UbloxGSMModemInitializer::getMSDPid() { return 0x0000; } 00038 00039 uint16_t UbloxGSMModemInitializer::getSerialVid() { return 0x1546; } 00040 uint16_t UbloxGSMModemInitializer::getSerialPid() { return 0x1102; } 00041 00042 bool UbloxGSMModemInitializer::switchMode(USBDeviceConnected* pDev) 00043 { 00044 for (int i = 0; i < pDev->getNbIntf(); i++) 00045 { 00046 if (pDev->getInterface(i)->intf_class == MSD_CLASS) 00047 { 00048 USBEndpoint* pEp = pDev->getEndpoint(i, BULK_ENDPOINT, OUT); 00049 if ( pEp != NULL ) 00050 { 00051 ERR("MSD descriptor found on device %p, intf %d", (void *)pDev, i); 00052 } 00053 } 00054 } 00055 return false; 00056 } 00057 00058 #define UBX_SERIALCOUNT 7 00059 00060 int UbloxGSMModemInitializer::getSerialPortCount() 00061 { 00062 return UBX_SERIALCOUNT; 00063 } 00064 00065 /*virtual*/ void UbloxGSMModemInitializer::setVidPid(uint16_t vid, uint16_t pid) 00066 { 00067 if( (vid == getSerialVid() ) && ( pid == getSerialPid() ) ) 00068 { 00069 m_hasSwitched = true; 00070 m_currentSerialIntf = 0; 00071 m_endpointsToFetch = UBX_SERIALCOUNT*2; 00072 } 00073 else 00074 { 00075 m_hasSwitched = false; 00076 m_endpointsToFetch = 1; 00077 } 00078 } 00079 00080 /*virtual*/ bool UbloxGSMModemInitializer::parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol) //Must return true if the interface should be parsed 00081 { 00082 if( m_hasSwitched ) 00083 { 00084 DBG("Interface #%d; Class:%02x; SubClass:%02x; Protocol:%02x", intf_nb, intf_class, intf_subclass, intf_protocol); 00085 if( intf_class == 0x0A ) 00086 { 00087 if( (m_currentSerialIntf == 0) || (m_currentSerialIntf == 1) ) 00088 { 00089 m_serialIntfMap[m_currentSerialIntf++] = intf_nb; 00090 return true; 00091 } 00092 m_currentSerialIntf++; 00093 } 00094 } 00095 else 00096 { 00097 if( (intf_nb == 0) && (intf_class == MSD_CLASS) ) 00098 { 00099 return true; 00100 } 00101 } 00102 return false; 00103 } 00104 00105 /*virtual*/ bool UbloxGSMModemInitializer::useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir) //Must return true if the endpoint will be used 00106 { 00107 if( m_hasSwitched ) 00108 { 00109 DBG("USBEndpoint on Interface #%d; Type:%d; Direction:%d", intf_nb, type, dir); 00110 if( (type == BULK_ENDPOINT) && m_endpointsToFetch ) 00111 { 00112 m_endpointsToFetch--; 00113 return true; 00114 } 00115 } 00116 else 00117 { 00118 if( (type == BULK_ENDPOINT) && (dir == OUT) && m_endpointsToFetch ) 00119 { 00120 m_endpointsToFetch--; 00121 return true; 00122 } 00123 } 00124 return false; 00125 } 00126 00127 /*virtual*/ int UbloxGSMModemInitializer::getType() 00128 { 00129 return WAN_DONGLE_TYPE_UBLOX_LISAU200; 00130 } 00131
Generated on Tue Jul 12 2022 19:17:01 by
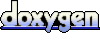