
u-blox modem HTTP client test
Dependencies: UbloxUSBModem mbed
HTTPText.h
00001 /* HTTPText.h */ 00002 /* Copyright (C) 2012 mbed.org, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 00021 #ifndef HTTPTEXT_H_ 00022 #define HTTPTEXT_H_ 00023 00024 #include "../IHTTPData.h" 00025 00026 /** A data endpoint to store text 00027 */ 00028 class HTTPText : public IHTTPDataIn, public IHTTPDataOut 00029 { 00030 public: 00031 /** Create an HTTPText instance for output 00032 * @param str String to be transmitted 00033 */ 00034 HTTPText(char* str); 00035 00036 /** Create an HTTPText instance for input 00037 * @param str Buffer to store the incoming string 00038 * @param size Size of the buffer 00039 */ 00040 HTTPText(char* str, size_t size); 00041 00042 protected: 00043 //IHTTPDataIn 00044 virtual void readReset(); 00045 00046 virtual int read(char* buf, size_t len, size_t* pReadLen); 00047 00048 virtual int getDataType(char* type, size_t maxTypeLen); //Internet media type for Content-Type header 00049 00050 virtual bool getIsChunked(); //For Transfer-Encoding header 00051 00052 virtual size_t getDataLen(); //For Content-Length header 00053 00054 //IHTTPDataOut 00055 virtual void writeReset(); 00056 00057 virtual int write(const char* buf, size_t len); 00058 00059 virtual void setDataType(const char* type); //Internet media type from Content-Type header 00060 00061 virtual void setIsChunked(bool chunked); //From Transfer-Encoding header 00062 00063 virtual void setDataLen(size_t len); //From Content-Length header, or if the transfer is chunked, next chunk length 00064 00065 private: 00066 char* m_str; 00067 size_t m_size; 00068 00069 size_t m_pos; 00070 }; 00071 00072 #endif /* HTTPTEXT_H_ */
Generated on Tue Jul 12 2022 14:33:52 by
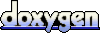