
u-blox modem HTTP client test
Dependencies: UbloxUSBModem mbed
HTTPText.cpp
00001 /* HTTPText.cpp */ 00002 /* Copyright (C) 2012 mbed.org, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #include "HTTPText.h" 00021 00022 #include <cstring> 00023 00024 #define OK 0 00025 00026 using std::memcpy; 00027 using std::strncpy; 00028 using std::strlen; 00029 00030 #define MIN(x,y) (((x)<(y))?(x):(y)) 00031 00032 HTTPText::HTTPText(char* str) : m_str(str), m_pos(0) 00033 { 00034 m_size = strlen(str) + 1; 00035 } 00036 00037 HTTPText::HTTPText(char* str, size_t size) : m_str(str), m_size(size), m_pos(0) 00038 { 00039 00040 } 00041 00042 //IHTTPDataIn 00043 /*virtual*/ void HTTPText::readReset() 00044 { 00045 m_pos = 0; 00046 } 00047 00048 /*virtual*/ int HTTPText::read(char* buf, size_t len, size_t* pReadLen) 00049 { 00050 *pReadLen = MIN(len, m_size - 1 - m_pos); 00051 memcpy(buf, m_str + m_pos, *pReadLen); 00052 m_pos += *pReadLen; 00053 return OK; 00054 } 00055 00056 /*virtual*/ int HTTPText::getDataType(char* type, size_t maxTypeLen) //Internet media type for Content-Type header 00057 { 00058 strncpy(type, "text/plain", maxTypeLen-1); 00059 type[maxTypeLen-1] = '\0'; 00060 return OK; 00061 } 00062 00063 /*virtual*/ bool HTTPText::getIsChunked() //For Transfer-Encoding header 00064 { 00065 return false; 00066 } 00067 00068 /*virtual*/ size_t HTTPText::getDataLen() //For Content-Length header 00069 { 00070 return m_size - 1; 00071 } 00072 00073 //IHTTPDataOut 00074 /*virtual*/ void HTTPText::writeReset() 00075 { 00076 m_pos = 0; 00077 } 00078 00079 /*virtual*/ int HTTPText::write(const char* buf, size_t len) 00080 { 00081 size_t writeLen = MIN(len, m_size - 1 - m_pos); 00082 memcpy(m_str + m_pos, buf, writeLen); 00083 m_pos += writeLen; 00084 m_str[m_pos] = '\0'; 00085 return OK; 00086 } 00087 00088 /*virtual*/ void HTTPText::setDataType(const char* type) //Internet media type from Content-Type header 00089 { 00090 00091 } 00092 00093 /*virtual*/ void HTTPText::setIsChunked(bool chunked) //From Transfer-Encoding header 00094 { 00095 00096 } 00097 00098 /*virtual*/ void HTTPText::setDataLen(size_t len) //From Content-Length header, or if the transfer is chunked, next chunk length 00099 { 00100 00101 } 00102 00103 00104
Generated on Tue Jul 12 2022 14:33:52 by
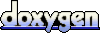