
u-blox modem HTTP client test
Dependencies: UbloxUSBModem mbed
HTTPClient.h
00001 /* HTTPClient.h */ 00002 /* Copyright (C) 2012 mbed.org, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 /** \file 00021 HTTP Client header file 00022 */ 00023 00024 #ifndef HTTP_CLIENT_H 00025 #define HTTP_CLIENT_H 00026 00027 #include "TCPSocketConnection.h" 00028 00029 #define HTTP_CLIENT_DEFAULT_TIMEOUT 15000 00030 00031 class HTTPData; 00032 00033 #include "IHTTPData.h" 00034 #include "mbed.h" 00035 00036 ///HTTP client results 00037 enum HTTPResult 00038 { 00039 HTTP_PROCESSING, ///<Processing 00040 HTTP_PARSE, ///<url Parse error 00041 HTTP_DNS, ///<Could not resolve name 00042 HTTP_PRTCL, ///<Protocol error 00043 HTTP_NOTFOUND, ///<HTTP 404 Error 00044 HTTP_REFUSED, ///<HTTP 403 Error 00045 HTTP_ERROR, ///<HTTP xxx error 00046 HTTP_TIMEOUT, ///<Connection timeout 00047 HTTP_CONN, ///<Connection error 00048 HTTP_CLOSED, ///<Connection was closed by remote host 00049 HTTP_OK = 0, ///<Success 00050 }; 00051 00052 /**A simple HTTP Client 00053 The HTTPClient is composed of: 00054 - The actual client (HTTPClient) 00055 - Classes that act as a data repository, each of which deriving from the HTTPData class (HTTPText for short text content, HTTPFile for file I/O, HTTPMap for key/value pairs, and HTTPStream for streaming purposes) 00056 */ 00057 class HTTPClient 00058 { 00059 public: 00060 ///Instantiate the HTTP client 00061 HTTPClient(); 00062 ~HTTPClient(); 00063 00064 #if 0 //TODO add header handlers 00065 /** 00066 Provides a basic authentification feature (Base64 encoded username and password) 00067 Pass two NULL pointers to switch back to no authentication 00068 @param user username to use for authentication, must remain valid durlng the whole HTTP session 00069 @param user password to use for authentication, must remain valid durlng the whole HTTP session 00070 */ 00071 void basicAuth(const char* user, const char* password); //Basic Authentification 00072 #endif 00073 00074 //High Level setup functions 00075 /** Execute a GET request on the URL 00076 Blocks until completion 00077 @param url : url on which to execute the request 00078 @param pDataIn : pointer to an IHTTPDataIn instance that will collect the data returned by the request, can be NULL 00079 @param timeout waiting timeout in ms (osWaitForever for blocking function, not recommended) 00080 @return 0 on success, HTTP error (<0) on failure 00081 */ 00082 HTTPResult get(const char* url, IHTTPDataIn* pDataIn, int timeout = HTTP_CLIENT_DEFAULT_TIMEOUT); //Blocking 00083 00084 /** Execute a GET request on the URL 00085 Blocks until completion 00086 This is a helper to directly get a piece of text from a HTTP result 00087 @param url : url on which to execute the request 00088 @param result : pointer to a char array in which the result will be stored 00089 @param maxResultLen : length of the char array (including space for the NULL-terminating char) 00090 @param timeout waiting timeout in ms (osWaitForever for blocking function, not recommended) 00091 @return 0 on success, HTTP error (<0) on failure 00092 */ 00093 HTTPResult get(const char* url, char* result, size_t maxResultLen, int timeout = HTTP_CLIENT_DEFAULT_TIMEOUT); //Blocking 00094 00095 /** Execute a POST request on the URL 00096 Blocks until completion 00097 @param url : url on which to execute the request 00098 @param dataOut : a IHTTPDataOut instance that contains the data that will be posted 00099 @param pDataIn : pointer to an IHTTPDataIn instance that will collect the data returned by the request, can be NULL 00100 @param timeout waiting timeout in ms (osWaitForever for blocking function, not recommended) 00101 @return 0 on success, HTTP error (<0) on failure 00102 */ 00103 HTTPResult post(const char* url, const IHTTPDataOut& dataOut, IHTTPDataIn* pDataIn, int timeout = HTTP_CLIENT_DEFAULT_TIMEOUT); //Blocking 00104 00105 /** Execute a PUT request on the URL 00106 Blocks until completion 00107 @param url : url on which to execute the request 00108 @param dataOut : a IHTTPDataOut instance that contains the data that will be put 00109 @param pDataIn : pointer to an IHTTPDataIn instance that will collect the data returned by the request, can be NULL 00110 @param timeout waiting timeout in ms (osWaitForever for blocking function, not recommended) 00111 @return 0 on success, HTTP error (<0) on failure 00112 */ 00113 HTTPResult put(const char* url, const IHTTPDataOut& dataOut, IHTTPDataIn* pDataIn, int timeout = HTTP_CLIENT_DEFAULT_TIMEOUT); //Blocking 00114 00115 /** Execute a DELETE request on the URL 00116 Blocks until completion 00117 @param url : url on which to execute the request 00118 @param pDataIn : pointer to an IHTTPDataIn instance that will collect the data returned by the request, can be NULL 00119 @param timeout waiting timeout in ms (osWaitForever for blocking function, not recommended) 00120 @return 0 on success, HTTP error (<0) on failure 00121 */ 00122 HTTPResult del(const char* url, IHTTPDataIn* pDataIn, int timeout = HTTP_CLIENT_DEFAULT_TIMEOUT); //Blocking 00123 00124 /** Get last request's HTTP response code 00125 @return The HTTP response code of the last request 00126 */ 00127 int getHTTPResponseCode(); 00128 00129 private: 00130 enum HTTP_METH 00131 { 00132 HTTP_GET, 00133 HTTP_POST, 00134 HTTP_PUT, 00135 HTTP_DELETE, 00136 HTTP_HEAD 00137 }; 00138 00139 HTTPResult connect(const char* url, HTTP_METH method, IHTTPDataOut* pDataOut, IHTTPDataIn* pDataIn, int timeout); //Execute request 00140 HTTPResult recv(char* buf, size_t minLen, size_t maxLen, size_t* pReadLen); //0 on success, err code on failure 00141 HTTPResult send(char* buf, size_t len = 0); //0 on success, err code on failure 00142 HTTPResult parseURL(const char* url, char* scheme, size_t maxSchemeLen, char* host, size_t maxHostLen, uint16_t* port, char* path, size_t maxPathLen); //Parse URL 00143 00144 //Parameters 00145 TCPSocketConnection m_sock; 00146 00147 int m_timeout; 00148 00149 const char* m_basicAuthUser; 00150 const char* m_basicAuthPassword; 00151 int m_httpResponseCode; 00152 00153 }; 00154 00155 //Including data containers here for more convenience 00156 #include "data/HTTPText.h" 00157 #include "data/HTTPMap.h" 00158 00159 #endif
Generated on Tue Jul 12 2022 14:33:52 by
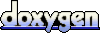