USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
USBDeviceConnected.cpp
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "USBDeviceConnected.h" 00018 #include "dbg.h" 00019 00020 USBDeviceConnected::USBDeviceConnected() { 00021 init(); 00022 } 00023 00024 void USBDeviceConnected::init() { 00025 hub_nb = 0; 00026 port = 0; 00027 vid = 0; 00028 pid = 0; 00029 nb_interf = 0; 00030 enumerated = false; 00031 activeAddr = false; 00032 sizeControlEndpoint = 8; 00033 device_class = 0; 00034 device_subclass = 0; 00035 proto = 0; 00036 speed = false; 00037 for (int i = 0; i < MAX_INTF; i++) { 00038 memset((void *)&intf[i], 0, sizeof(INTERFACE)); 00039 intf[i].in_use = false; 00040 for (int j = 0; j < MAX_ENDPOINT_PER_INTERFACE; j++) { 00041 intf[i].ep[j] = NULL; 00042 strcpy(intf[i].name, "Unknown"); 00043 } 00044 } 00045 hub_parent = NULL; 00046 hub = NULL; 00047 nb_interf = 0; 00048 } 00049 00050 INTERFACE * USBDeviceConnected::getInterface(uint8_t index) { 00051 if (index >= MAX_INTF) 00052 return NULL; 00053 00054 if (intf[index].in_use) 00055 return &intf[index]; 00056 00057 return NULL; 00058 } 00059 00060 bool USBDeviceConnected::addInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol) { 00061 if ((intf_nb >= MAX_INTF) || (intf[intf_nb].in_use)) { 00062 return false; 00063 } 00064 intf[intf_nb].in_use = true; 00065 intf[intf_nb].intf_class = intf_class; 00066 intf[intf_nb].intf_subclass = intf_subclass; 00067 intf[intf_nb].intf_protocol = intf_protocol; 00068 intf[intf_nb].nb_endpoint = 0; 00069 return true; 00070 } 00071 00072 bool USBDeviceConnected::addEndpoint(uint8_t intf_nb, USBEndpoint * ept) { 00073 if ((intf_nb >= MAX_INTF) || (intf[intf_nb].in_use == false) || (intf[intf_nb].nb_endpoint >= MAX_ENDPOINT_PER_INTERFACE)) { 00074 return false; 00075 } 00076 intf[intf_nb].nb_endpoint++; 00077 00078 for (int i = 0; i < MAX_ENDPOINT_PER_INTERFACE; i++) { 00079 if (intf[intf_nb].ep[i] == NULL) { 00080 intf[intf_nb].ep[i] = ept; 00081 return true; 00082 } 00083 } 00084 return false; 00085 } 00086 00087 void USBDeviceConnected::init(uint8_t hub_, uint8_t port_, bool lowSpeed_) { 00088 USB_DBG("init dev: %p", this); 00089 init(); 00090 hub_nb = hub_; 00091 port = port_; 00092 speed = lowSpeed_; 00093 } 00094 00095 void USBDeviceConnected::disconnect() { 00096 for(int i = 0; i < MAX_INTF; i++) { 00097 if (intf[i].detach) intf[i].detach.call(); 00098 } 00099 init(); 00100 } 00101 00102 00103 USBEndpoint * USBDeviceConnected::getEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir, uint8_t index) { 00104 if (intf_nb >= MAX_INTF) { 00105 return NULL; 00106 } 00107 for (int i = 0; i < MAX_ENDPOINT_PER_INTERFACE; i++) { 00108 if ((intf[intf_nb].ep[i]->getType() == type) && (intf[intf_nb].ep[i]->getDir() == dir)) { 00109 if(index) { 00110 index--; 00111 } else { 00112 return intf[intf_nb].ep[i]; 00113 } 00114 } 00115 } 00116 return NULL; 00117 } 00118 00119 USBEndpoint * USBDeviceConnected::getEndpoint(uint8_t intf_nb, uint8_t index) { 00120 if ((intf_nb >= MAX_INTF) || (index >= MAX_ENDPOINT_PER_INTERFACE)) { 00121 return NULL; 00122 } 00123 return intf[intf_nb].ep[index]; 00124 }
Generated on Tue Jul 12 2022 13:32:26 by
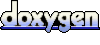