mbed socket API
Dependents: EthernetInterface EthernetInterface_RSF EthernetInterface EthernetInterface ... more
UDPSocket.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef UDPSOCKET_H 00020 #define UDPSOCKET_H 00021 00022 #include "Socket/Socket.h" 00023 #include "Socket/Endpoint.h" 00024 00025 /** 00026 UDP Socket 00027 */ 00028 class UDPSocket : public Socket { 00029 00030 public: 00031 /** Instantiate an UDP Socket. 00032 */ 00033 UDPSocket(); 00034 00035 /** Init the UDP Client Socket without binding it to any specific port 00036 \return 0 on success, -1 on failure. 00037 */ 00038 int init(void); 00039 00040 /** Bind a UDP Server Socket to a specific port 00041 \param port The port to listen for incoming connections on 00042 \return 0 on success, -1 on failure. 00043 */ 00044 int bind(int port); 00045 00046 /** Join the multicast group at the given address 00047 \param address The address of the multicast group 00048 \return 0 on success, -1 on failure. 00049 */ 00050 int join_multicast_group(const char* address); 00051 00052 /** Set the socket in broadcasting mode 00053 \return 0 on success, -1 on failure. 00054 */ 00055 int set_broadcasting(bool broadcast=true); 00056 00057 /** Send a packet to a remote endpoint 00058 \param remote The remote endpoint 00059 \param packet The packet to be sent 00060 \param length The length of the packet to be sent 00061 \return the number of written bytes on success (>=0) or -1 on failure 00062 */ 00063 int sendTo(Endpoint &remote, char *packet, int length); 00064 00065 /** Receive a packet from a remote endpoint 00066 \param remote The remote endpoint 00067 \param buffer The buffer for storing the incoming packet data. If a packet 00068 is too long to fit in the supplied buffer, excess bytes are discarded 00069 \param length The length of the buffer 00070 \return the number of received bytes on success (>=0) or -1 on failure 00071 */ 00072 int receiveFrom(Endpoint &remote, char *buffer, int length); 00073 }; 00074 00075 #endif
Generated on Tue Jul 12 2022 11:05:10 by
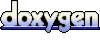