mbed socket API
Dependents: EthernetInterface EthernetInterface_RSF EthernetInterface EthernetInterface ... more
Socket.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #ifndef SOCKET_H_ 00019 #define SOCKET_H_ 00020 00021 #include "lwip/sockets.h" 00022 #include "lwip/netdb.h" 00023 00024 //DNS 00025 inline struct hostent *gethostbyname(const char *name) { 00026 return lwip_gethostbyname(name); 00027 } 00028 00029 inline int gethostbyname_r(const char *name, struct hostent *ret, char *buf, size_t buflen, struct hostent **result, int *h_errnop) { 00030 return lwip_gethostbyname_r(name, ret, buf, buflen, result, h_errnop); 00031 } 00032 00033 class TimeInterval; 00034 00035 /** Socket file descriptor and select wrapper 00036 */ 00037 class Socket { 00038 public: 00039 /** Socket 00040 */ 00041 Socket(); 00042 00043 /** Set blocking or non-blocking mode of the socket and a timeout on 00044 blocking socket operations 00045 \param blocking true for blocking mode, false for non-blocking mode. 00046 \param timeout timeout in ms [Default: (1500)ms]. 00047 */ 00048 void set_blocking(bool blocking, unsigned int timeout=1500); 00049 00050 /** Set socket options 00051 \param level stack level (see: lwip/sockets.h) 00052 \param optname option ID 00053 \param optval option value 00054 \param socklen_t length of the option value 00055 \return 0 on success, -1 on failure 00056 */ 00057 int set_option(int level, int optname, const void *optval, socklen_t optlen); 00058 00059 /** Get socket options 00060 \param level stack level (see: lwip/sockets.h) 00061 \param optname option ID 00062 \param optval buffer pointer where to write the option value 00063 \param socklen_t length of the option value 00064 \return 0 on success, -1 on failure 00065 */ 00066 int get_option(int level, int optname, void *optval, socklen_t *optlen); 00067 00068 /** Close the socket 00069 \param shutdown free the left-over data in message queues 00070 */ 00071 int close(bool shutdown=true); 00072 00073 ~Socket(); 00074 00075 protected: 00076 int _sock_fd; 00077 int init_socket(int type); 00078 00079 int wait_readable(TimeInterval& timeout); 00080 int wait_writable(TimeInterval& timeout); 00081 00082 bool _blocking; 00083 unsigned int _timeout; 00084 00085 private: 00086 int select(struct timeval *timeout, bool read, bool write); 00087 }; 00088 00089 /** Time interval class used to specify timeouts 00090 */ 00091 class TimeInterval { 00092 friend class Socket; 00093 00094 public: 00095 /** Time Interval 00096 \param ms time interval expressed in milliseconds 00097 */ 00098 TimeInterval(unsigned int ms); 00099 00100 private: 00101 struct timeval _time; 00102 }; 00103 00104 #endif /* SOCKET_H_ */
Generated on Tue Jul 12 2022 11:05:10 by
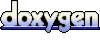