
Example multicasting of packets
Dependencies: EthernetInterface mbed-rtos mbed
Fork of MulticastSend by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 00004 const char* MCAST_GRP = "224.1.1.1"; 00005 const int MCAST_PORT = 5007; 00006 00007 int main() { 00008 EthernetInterface eth; 00009 eth.init(); //Use DHCP 00010 eth.connect(); 00011 00012 UDPSocket sock; 00013 sock.init(); 00014 00015 Endpoint multicast_group; 00016 multicast_group.set_address(MCAST_GRP, MCAST_PORT); 00017 00018 char out_buffer[] = "very important data"; 00019 while (true) { 00020 printf("Multicast to group: %s\n", MCAST_GRP); 00021 sock.sendTo(multicast_group, out_buffer, sizeof(out_buffer)); 00022 Thread::wait(1000); 00023 } 00024 }
Generated on Tue Jul 12 2022 21:27:54 by
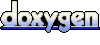