
Hello World for I2C
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 // Read temperature from LM75BD 00004 00005 I2C i2c(p28, p27); 00006 00007 const int addr = 0x90; 00008 00009 int main() { 00010 char cmd[2]; 00011 while (1) { 00012 cmd[0] = 0x01; 00013 cmd[1] = 0x00; 00014 i2c.write(addr, cmd, 2); 00015 00016 wait(0.5); 00017 00018 cmd[0] = 0x00; 00019 i2c.write(addr, cmd, 1); 00020 i2c.read(addr, cmd, 2); 00021 00022 float tmp = (float((cmd[0]<<8)|cmd[1]) / 256.0); 00023 printf("Temp = %.2f\n", tmp); 00024 } 00025 }
Generated on Tue Jul 12 2022 13:20:49 by
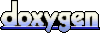