Base library for cellular modem implementations
Dependencies: Socket lwip-sys lwip
Dependents: CellularUSBModem CellularUSBModem
LinkMonitor.h
00001 /* LinkMonitor.h */ 00002 /* Copyright (C) 2012 mbed.org, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #ifndef LINKMONITOR_H_ 00021 #define LINKMONITOR_H_ 00022 00023 #include "core/fwk.h" 00024 00025 #include "rtos.h" 00026 00027 #include "at/ATCommandsInterface.h" 00028 00029 /** Component to monitor link quality 00030 * 00031 */ 00032 class LinkMonitor : protected IATCommandsProcessor 00033 { 00034 public: 00035 /** Create LinkMonitor instance 00036 @param pIf Pointer to the ATCommandsInterface instance to use 00037 */ 00038 LinkMonitor(ATCommandsInterface* pIf); 00039 00040 /** Initialize monitor 00041 */ 00042 int init(bool gsm = true); 00043 00044 /** Registration State 00045 */ 00046 enum REGISTRATION_STATE 00047 { 00048 REGISTRATION_STATE_UNKNOWN, //!< Unknown 00049 REGISTRATION_STATE_REGISTERING, //!< Registering 00050 REGISTRATION_STATE_DENIED, //!< Denied 00051 REGISTRATION_STATE_NO_SIGNAL, //!< No Signal 00052 REGISTRATION_STATE_HOME_NETWORK, //!< Registered on home network 00053 REGISTRATION_STATE_ROAMING //!< Registered on roaming network 00054 }; 00055 00056 /** Bearer type 00057 */ 00058 enum BEARER 00059 { 00060 BEARER_UNKNOWN, //!< Unknown 00061 BEARER_GSM, //!< GSM (2G) 00062 BEARER_EDGE, //!< EDGE (2.5G) 00063 BEARER_UMTS, //!< UMTS (3G) 00064 BEARER_HSPA, //!< HSPA (3G+) 00065 BEARER_LTE //!< LTE (4G) 00066 }; 00067 00068 /** Get link state 00069 @param pRssi pointer to store the current RSSI in dBm, between -51 dBm and -113 dBm if known; -51 dBm means -51 dBm or more; -113 dBm means -113 dBm or less; 0 if unknown 00070 @param pRegistrationState pointer to store the current registration state 00071 @param pBearer pointer to store the current bearer 00072 @return 0 on success, error code on failure 00073 */ 00074 int getState(int* pRssi, REGISTRATION_STATE* pRegistrationState, BEARER* pBearer); 00075 00076 /** Get my phone number 00077 @param phoneNumber pointer to store the current phoneNumber 00078 @return 0 on success, error code on failure 00079 */ 00080 int getPhoneNumber(char* phoneNumber); 00081 protected: 00082 //IATCommandsProcessor 00083 virtual int onNewATResponseLine(ATCommandsInterface* pInst, const char* line); 00084 virtual int onNewEntryPrompt(ATCommandsInterface* pInst); 00085 00086 private: 00087 ATCommandsInterface* m_pIf; 00088 00089 int m_rssi; 00090 bool m_gsm; 00091 REGISTRATION_STATE m_registrationState; 00092 BEARER m_bearer; 00093 char m_phoneNumber[16]; 00094 }; 00095 00096 #endif /* LINKMONITOR_H_ */
Generated on Tue Jul 26 2022 09:42:59 by
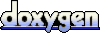