
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
sn_coap_protocol.c File Reference
CoAP Protocol implementation. More...
Go to the source code of this file.
Functions | |
static int8_t | sn_coap_protocol_linked_list_duplication_info_search (sn_nsdl_addr_s *scr_addr_ptr, uint16_t msg_id) |
Searches stored message from Linked list (Address and Message ID as key) | |
static void | sn_coap_protocol_linked_list_duplication_info_remove (struct coap_s *handle, uint8_t *scr_addr_ptr, uint16_t port, uint16_t msg_id) |
Removes stored Duplication info from Linked list. | |
static void | sn_coap_protocol_linked_list_duplication_info_remove_old_ones (struct coap_s *handle) |
Removes old stored Duplication detection infos from Linked list. | |
static void | sn_coap_protocol_linked_list_blockwise_msg_remove (struct coap_s *handle, coap_blockwise_msg_s *removed_msg_ptr) |
Removes stored blockwise message from Linked list. | |
static uint8_t * | sn_coap_protocol_linked_list_blockwise_payload_search (sn_nsdl_addr_s *src_addr_ptr, uint16_t *payload_length) |
Searches stored blockwise payload from Linked list (Address as key) | |
static void | sn_coap_protocol_linked_list_blockwise_payload_remove_oldest (struct coap_s *handle) |
Removes current stored blockwise paylod from Linked list. | |
static uint16_t | sn_coap_protocol_linked_list_blockwise_payloads_get_len (sn_nsdl_addr_s *src_addr_ptr) |
Counts length of Payloads in Linked list (Address as key) | |
static void | sn_coap_protocol_linked_list_blockwise_remove_old_data (struct coap_s *handle) |
Removes old stored Blockwise messages and payloads from Linked list. | |
static sn_nsdl_transmit_s * | sn_coap_protocol_linked_list_send_msg_search (sn_nsdl_addr_s *src_addr_ptr, uint16_t msg_id) |
Searches stored resending message from Linked list. | |
static void | sn_coap_protocol_release_allocated_send_msg_mem (struct coap_s *handle, coap_send_msg_s *freed_send_msg_ptr) |
Releases memory of given Sending message (coap_send_msg_s) | |
static uint16_t | sn_coap_count_linked_list_size (const coap_send_msg_list_t *linked_list_ptr) |
Counts total message size of all messages in linked list. | |
int8_t | sn_coap_protocol_set_block_size (uint16_t block_size) |
If block transfer is enabled, this function changes the block size. | |
int8_t | sn_coap_protocol_set_duplicate_buffer_size (uint8_t message_count) |
If dublicate message detection is enabled, this function changes buffer size. | |
int8_t | sn_coap_protocol_set_retransmission_parameters (uint8_t resending_count, uint8_t resending_intervall) |
If re-transmissions are enabled, this function changes resending count and interval. | |
int8_t | sn_coap_protocol_set_retransmission_buffer (uint8_t buffer_size_messages, uint16_t buffer_size_bytes) |
If re-transmissions are enabled, this function changes message retransmission queue size. | |
void | sn_coap_protocol_clear_retransmission_buffer (struct coap_s *handle) |
If re-transmissions are enabled, this function removes all messages from the retransmission queue. | |
int8_t | sn_coap_protocol_exec (struct coap_s *handle, uint32_t current_time) |
Sends CoAP messages from re-sending queue, if there is any. |
Detailed Description
CoAP Protocol implementation.
Functionality: CoAP Protocol
Definition in file sn_coap_protocol.c.
Function Documentation
static uint16_t sn_coap_count_linked_list_size | ( | const coap_send_msg_list_t * | linked_list_ptr ) | [static] |
Counts total message size of all messages in linked list.
- Parameters:
-
const coap_send_msg_list_t *linked_list_ptr pointer to linked list
Definition at line 1419 of file sn_coap_protocol.c.
void sn_coap_protocol_clear_retransmission_buffer | ( | struct coap_s * | handle ) |
If re-transmissions are enabled, this function removes all messages from the retransmission queue.
- Parameters:
-
*handle Pointer to CoAP library handle
Definition at line 296 of file sn_coap_protocol.c.
int8_t sn_coap_protocol_exec | ( | struct coap_s * | handle, |
uint32_t | current_time | ||
) |
Sends CoAP messages from re-sending queue, if there is any.
Cleans also old messages from the duplication list and from block receiving list
This function can be called e.g. once in a second but also more frequently.
- Parameters:
-
*handle Pointer to CoAP library handle current_time is System time in seconds. This time is used for message re-sending timing and to identify old saved data.
- Returns:
- 0 if success -1 if failed
Definition at line 689 of file sn_coap_protocol.c.
static void sn_coap_protocol_linked_list_blockwise_msg_remove | ( | struct coap_s * | handle, |
coap_blockwise_msg_s * | removed_msg_ptr | ||
) | [static] |
Removes stored blockwise message from Linked list.
- Parameters:
-
removed_msg_ptr is message to be removed
Definition at line 1085 of file sn_coap_protocol.c.
static void sn_coap_protocol_linked_list_blockwise_payload_remove_oldest | ( | struct coap_s * | handle ) | [static] |
Removes current stored blockwise paylod from Linked list.
Definition at line 1207 of file sn_coap_protocol.c.
static uint8_t * sn_coap_protocol_linked_list_blockwise_payload_search | ( | sn_nsdl_addr_s * | src_addr_ptr, |
uint16_t * | payload_length | ||
) | [static] |
Searches stored blockwise payload from Linked list (Address as key)
- Parameters:
-
*addr_ptr is pointer to Address key to be searched *payload_length is pointer to returned Payload length
- Returns:
- Return value is pointer to found stored blockwise payload in Linked list or NULL if payload not found
Definition at line 1182 of file sn_coap_protocol.c.
static uint16_t sn_coap_protocol_linked_list_blockwise_payloads_get_len | ( | sn_nsdl_addr_s * | src_addr_ptr ) | [static] |
Counts length of Payloads in Linked list (Address as key)
- Parameters:
-
*addr_ptr is pointer to Address key
- Returns:
- Return value is length of Payloads as bytes
Definition at line 1258 of file sn_coap_protocol.c.
static void sn_coap_protocol_linked_list_blockwise_remove_old_data | ( | struct coap_s * | handle ) | [static] |
Removes old stored Blockwise messages and payloads from Linked list.
Definition at line 1283 of file sn_coap_protocol.c.
static void sn_coap_protocol_linked_list_duplication_info_remove | ( | struct coap_s * | handle, |
uint8_t * | addr_ptr, | ||
uint16_t | port, | ||
uint16_t | msg_id | ||
) | [static] |
Removes stored Duplication info from Linked list.
- Parameters:
-
*addr_ptr is pointer to Address key to be removed port is Port key to be removed msg_id is Message ID key to be removed
Definition at line 1023 of file sn_coap_protocol.c.
static void sn_coap_protocol_linked_list_duplication_info_remove_old_ones | ( | struct coap_s * | handle ) | [static] |
Removes old stored Duplication detection infos from Linked list.
Definition at line 1056 of file sn_coap_protocol.c.
static int8_t sn_coap_protocol_linked_list_duplication_info_search | ( | sn_nsdl_addr_s * | addr_ptr, |
uint16_t | msg_id | ||
) | [static] |
Searches stored message from Linked list (Address and Message ID as key)
- Parameters:
-
*addr_ptr is pointer to Address key to be searched msg_id is Message ID key to be searched
- Returns:
- Return value is 0 when message found and -1 if not found
Definition at line 990 of file sn_coap_protocol.c.
static sn_nsdl_transmit_s * sn_coap_protocol_linked_list_send_msg_search | ( | sn_nsdl_addr_s * | src_addr_ptr, |
uint16_t | msg_id | ||
) | [static] |
Searches stored resending message from Linked list.
- Parameters:
-
*src_addr_ptr is searching key for searched message msg_id is searching key for searched message
- Returns:
- Return value is pointer to found stored resending message in Linked list or NULL if message not found
Definition at line 845 of file sn_coap_protocol.c.
static void sn_coap_protocol_release_allocated_send_msg_mem | ( | struct coap_s * | handle, |
coap_send_msg_s * | freed_send_msg_ptr | ||
) | [static] |
Releases memory of given Sending message (coap_send_msg_s)
- Parameters:
-
*freed_send_msg_ptr is pointer to released Sending message
Definition at line 1384 of file sn_coap_protocol.c.
int8_t sn_coap_protocol_set_block_size | ( | uint16_t | block_size ) |
If block transfer is enabled, this function changes the block size.
- Parameters:
-
uint16_t block_size maximum size of CoAP payload. Valid sizes are 16, 32, 64, 128, 256, 512 and 1024 bytes
- Returns:
- 0 = success -1 = failure
Definition at line 230 of file sn_coap_protocol.c.
int8_t sn_coap_protocol_set_duplicate_buffer_size | ( | uint8_t | message_count ) |
If dublicate message detection is enabled, this function changes buffer size.
- Parameters:
-
uint8_t message_count max number of messages saved for duplicate control
- Returns:
- 0 = success -1 = failure
Definition at line 252 of file sn_coap_protocol.c.
int8_t sn_coap_protocol_set_retransmission_buffer | ( | uint8_t | buffer_size_messages, |
uint16_t | buffer_size_bytes | ||
) |
If re-transmissions are enabled, this function changes message retransmission queue size.
Set size to '0' to disable feature. If both are set to '0', then re-sendings are disabled.
- Parameters:
-
uint8_t buffer_size_messages queue size - maximum number of messages to be saved to queue uint8_t buffer_size_bytes queue size - maximum size of messages saved to queue
- Returns:
- 0 = success -1 = failure
Definition at line 281 of file sn_coap_protocol.c.
int8_t sn_coap_protocol_set_retransmission_parameters | ( | uint8_t | resending_count, |
uint8_t | resending_intervall | ||
) |
If re-transmissions are enabled, this function changes resending count and interval.
- Parameters:
-
uint8_t resending_count max number of resendings for message uint8_t resending_intervall message resending intervall in seconds
- Returns:
- 0 = success -1 = failure
Definition at line 263 of file sn_coap_protocol.c.
Generated on Tue Jul 12 2022 18:27:27 by
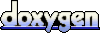