
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
nsdlaccesshelper.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "include/nsdlaccesshelper.h" 00017 #include "include/m2mnsdlinterface.h" 00018 00019 // callback function for NSDL library to call into 00020 M2MNsdlInterface *__nsdl_interface = NULL; 00021 00022 uint8_t __nsdl_c_callback(struct nsdl_s *nsdl_handle, 00023 sn_coap_hdr_s *received_coap_ptr, 00024 sn_nsdl_addr_s *address, 00025 sn_nsdl_capab_e nsdl_capab) 00026 { 00027 uint8_t status = 0; 00028 if(__nsdl_interface) { 00029 status = __nsdl_interface->resource_callback(nsdl_handle,received_coap_ptr, 00030 address, nsdl_capab); 00031 } 00032 return status; 00033 } 00034 00035 void* __nsdl_c_memory_alloc(uint16_t size) 00036 { 00037 void * val = NULL; 00038 if(__nsdl_interface) { 00039 val = __nsdl_interface->memory_alloc(size); 00040 } 00041 return val; 00042 } 00043 00044 void __nsdl_c_memory_free(void *ptr) 00045 { 00046 if(__nsdl_interface) { 00047 __nsdl_interface->memory_free(ptr); 00048 } 00049 } 00050 00051 uint8_t __nsdl_c_send_to_server(struct nsdl_s * nsdl_handle, 00052 sn_nsdl_capab_e protocol, 00053 uint8_t *data_ptr, 00054 uint16_t data_len, 00055 sn_nsdl_addr_s *address_ptr) 00056 { 00057 uint8_t status = 0; 00058 if(__nsdl_interface) { 00059 status = __nsdl_interface->send_to_server_callback(nsdl_handle, 00060 protocol, data_ptr, 00061 data_len, address_ptr); 00062 } 00063 return status; 00064 } 00065 00066 uint8_t __nsdl_c_received_from_server(struct nsdl_s * nsdl_handle, 00067 sn_coap_hdr_s *coap_header, 00068 sn_nsdl_addr_s *address_ptr) 00069 { 00070 uint8_t status = 0; 00071 if(__nsdl_interface) { 00072 status = __nsdl_interface->received_from_server_callback(nsdl_handle, 00073 coap_header, 00074 address_ptr); 00075 } 00076 return status; 00077 } 00078 00079 void __nsdl_c_bootstrap_done(sn_nsdl_oma_server_info_t *server_info_ptr) 00080 { 00081 if(__nsdl_interface) { 00082 __nsdl_interface->bootstrap_done_callback(server_info_ptr); 00083 } 00084 } 00085 00086 void* __socket_malloc( void * context, size_t size) 00087 { 00088 (void) context; 00089 return malloc(size); 00090 } 00091 00092 void __socket_free(void * context, void * ptr) 00093 { 00094 (void) context; 00095 free(ptr); 00096 }
Generated on Tue Jul 12 2022 18:27:24 by
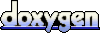