
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
M2MNsdlInterface Class Reference
M2MNsdlInterface Class which interacts between mbed Client C++ Library and mbed-client-c library. More...
#include <m2mnsdlinterface.h>
Inherits M2MTimerObserver, and M2MObservationHandler.
Public Member Functions | |
M2MNsdlInterface (M2MNsdlObserver &observer) | |
Constructor. | |
virtual | ~M2MNsdlInterface () |
Destructor. | |
void | create_endpoint (const String &endpoint_name, const String &endpoint_type, const int32_t life_time, const String &domain, const uint8_t mode, const String &context_address) |
Creates endpoint object for the nsdl stack. | |
void | delete_endpoint () |
Deletes the endpoint. | |
bool | create_nsdl_list_structure (const M2MObjectList &object_list) |
Creates the NSDL structure for the registered objectlist. | |
bool | delete_nsdl_resource (const String &resource_name) |
Removed the NSDL resource for the given resource. | |
bool | create_bootstrap_resource (sn_nsdl_addr_s *address) |
Creates the bootstrap object. | |
bool | send_register_message (uint8_t *address, const uint16_t port, sn_nsdl_addr_type_e address_type) |
Sends the register message to the server. | |
bool | send_update_registration (const uint32_t lifetime=0) |
Sends the update registration message to the server. | |
bool | send_unregister_message () |
Sends unregister message to the server. | |
void * | memory_alloc (uint16_t size) |
Memory Allocation required for libCoap. | |
void | memory_free (void *ptr) |
Memory free functions required for libCoap. | |
uint8_t | send_to_server_callback (struct nsdl_s *nsdl_handle, sn_nsdl_capab_e protocol, uint8_t *data, uint16_t data_len, sn_nsdl_addr_s *address) |
Callback from nsdl library to inform the data is ready to be sent to server. | |
uint8_t | received_from_server_callback (struct nsdl_s *nsdl_handle, sn_coap_hdr_s *coap_header, sn_nsdl_addr_s *address) |
Callback from nsdl library to inform the data which is received from server for the client has been converted to coap message. | |
uint8_t | resource_callback (struct nsdl_s *nsdl_handle, sn_coap_hdr_s *coap, sn_nsdl_addr_s *address, sn_nsdl_capab_e nsdl_capab) |
Callback from nsdl library to inform the data which is received from server for the resources has been converted to coap message. | |
void | bootstrap_done_callback (sn_nsdl_oma_server_info_t *server_info) |
Callback when the bootstrap information is received from bootstrap server. | |
bool | process_received_data (uint8_t *data, uint16_t data_size, sn_nsdl_addr_s *address) |
Callback when there is data received from server and needs to be processed. | |
void | stop_timers () |
Stops all the timers in case there is any errors. | |
Protected Member Functions | |
virtual void | timer_expired (M2MTimerObserver::Type type) |
Indicates that the time has expired. | |
virtual void | observation_to_be_sent (M2MBase *object) |
Observation callback to be sent to the server due to a change in a parameter under observation. | |
virtual void | resource_to_be_deleted (const String &resource_name) |
Callback for deleting an NSDL resource. | |
virtual void | value_updated (M2MBase *base, const String &object_name) |
Callback indicating that the value of the resource object is updated by server. | |
virtual void | remove_object (M2MBase *object) |
Callback for removing an object from the list. |
Detailed Description
M2MNsdlInterface Class which interacts between mbed Client C++ Library and mbed-client-c library.
Definition at line 43 of file m2mnsdlinterface.h.
Constructor & Destructor Documentation
M2MNsdlInterface | ( | M2MNsdlObserver & | observer ) |
Constructor.
- Parameters:
-
observer,Observer to pass the event callbacks from nsdl library.
Definition at line 29 of file m2mnsdlinterface.cpp.
~M2MNsdlInterface | ( | ) | [virtual] |
Destructor.
Definition at line 66 of file m2mnsdlinterface.cpp.
Member Function Documentation
void bootstrap_done_callback | ( | sn_nsdl_oma_server_info_t * | server_info ) |
Callback when the bootstrap information is received from bootstrap server.
- Parameters:
-
server_info,Server information received from bootstrap server.
Definition at line 583 of file m2mnsdlinterface.cpp.
bool create_bootstrap_resource | ( | sn_nsdl_addr_s * | address ) |
Creates the bootstrap object.
- Parameters:
-
address Bootstrap address.
- Returns:
- true if created and sent successfully else false.
Definition at line 215 of file m2mnsdlinterface.cpp.
void create_endpoint | ( | const String & | endpoint_name, |
const String & | endpoint_type, | ||
const int32_t | life_time, | ||
const String & | domain, | ||
const uint8_t | mode, | ||
const String & | context_address | ||
) |
Creates endpoint object for the nsdl stack.
- Parameters:
-
endpoint_name,Endpoint name of the client. endpoint_type,Endpoint type of the client. life_time,Life time of the client in seconds domain,Domain of the client. mode,Binding mode of the client, default is UDP context_address,Context address default is empty.
Definition at line 135 of file m2mnsdlinterface.cpp.
bool create_nsdl_list_structure | ( | const M2MObjectList & | object_list ) |
Creates the NSDL structure for the registered objectlist.
- Parameters:
-
object_list,List of objects to be registered.
- Returns:
- true if structure created successfully else false.
Definition at line 190 of file m2mnsdlinterface.cpp.
void delete_endpoint | ( | ) |
Deletes the endpoint.
Definition at line 177 of file m2mnsdlinterface.cpp.
bool delete_nsdl_resource | ( | const String & | resource_name ) |
Removed the NSDL resource for the given resource.
- Parameters:
-
resource_name,Resource name to be removed.
- Returns:
- true if removed successfully else false.
Definition at line 207 of file m2mnsdlinterface.cpp.
void * memory_alloc | ( | uint16_t | size ) |
Memory Allocation required for libCoap.
- Parameters:
-
size,Size of memory to be reserved.
Definition at line 314 of file m2mnsdlinterface.cpp.
void memory_free | ( | void * | ptr ) |
Memory free functions required for libCoap.
- Parameters:
-
ptr,Object whose memory needs to be freed.
Definition at line 322 of file m2mnsdlinterface.cpp.
void observation_to_be_sent | ( | M2MBase * | object ) | [protected, virtual] |
Observation callback to be sent to the server due to a change in a parameter under observation.
- Parameters:
-
object,Observed object whose information needs to be sent.
Implements M2MObservationHandler.
Definition at line 720 of file m2mnsdlinterface.cpp.
bool process_received_data | ( | uint8_t * | data, |
uint16_t | data_size, | ||
sn_nsdl_addr_s * | address | ||
) |
Callback when there is data received from server and needs to be processed.
- Parameters:
-
data,data received from server. data_size,data size received from server. addres,address structure of the server.
- Returns:
- true if successfully processed else false.
Definition at line 690 of file m2mnsdlinterface.cpp.
uint8_t received_from_server_callback | ( | struct nsdl_s * | nsdl_handle, |
sn_coap_hdr_s * | coap_header, | ||
sn_nsdl_addr_s * | address | ||
) |
Callback from nsdl library to inform the data which is received from server for the client has been converted to coap message.
- Parameters:
-
nsdl_handle,Handler for the nsdl structure for this endpoint coap_header,Coap message formed from data. address,Server address from where the data is received.
- Returns:
- 1 if successful else 0.
Definition at line 339 of file m2mnsdlinterface.cpp.
void remove_object | ( | M2MBase * | object ) | [protected, virtual] |
Callback for removing an object from the list.
- Parameters:
-
object,M2MObject to be removed.
Implements M2MObservationHandler.
Definition at line 769 of file m2mnsdlinterface.cpp.
uint8_t resource_callback | ( | struct nsdl_s * | nsdl_handle, |
sn_coap_hdr_s * | coap, | ||
sn_nsdl_addr_s * | address, | ||
sn_nsdl_capab_e | nsdl_capab | ||
) |
Callback from nsdl library to inform the data which is received from server for the resources has been converted to coap message.
- Parameters:
-
nsdl_handle,Handler for the nsdl resource structure for this endpoint.. coap_header,Coap message formed from data. address,Server address from where the data is received. nsdl_capab,Protocol for the message, currently only coap is supported.
- Returns:
- 1 if successful else 0.
Definition at line 513 of file m2mnsdlinterface.cpp.
void resource_to_be_deleted | ( | const String & | resource_name ) | [protected, virtual] |
Callback for deleting an NSDL resource.
- Parameters:
-
resource_name,Name of the observed object whose information needs to be deleted.
Implements M2MObservationHandler.
Definition at line 735 of file m2mnsdlinterface.cpp.
bool send_register_message | ( | uint8_t * | address, |
const uint16_t | port, | ||
sn_nsdl_addr_type_e | address_type | ||
) |
Sends the register message to the server.
- Returns:
- true if register sent successfully else false.
Definition at line 237 of file m2mnsdlinterface.cpp.
uint8_t send_to_server_callback | ( | struct nsdl_s * | nsdl_handle, |
sn_nsdl_capab_e | protocol, | ||
uint8_t * | data, | ||
uint16_t | data_len, | ||
sn_nsdl_addr_s * | address | ||
) |
Callback from nsdl library to inform the data is ready to be sent to server.
- Parameters:
-
nsdl_handle,Handler for the nsdl structure for this endpoint protocol,Protocol format of the data data,Data to be sent. data_len,Size of the data to be sent address,server address where data has to be sent.
- Returns:
- 1 if successful else 0.
Definition at line 328 of file m2mnsdlinterface.cpp.
bool send_unregister_message | ( | ) |
Sends unregister message to the server.
- Returns:
- true if unregister sent successfully else false.
Definition at line 301 of file m2mnsdlinterface.cpp.
bool send_update_registration | ( | const uint32_t | lifetime = 0 ) |
Sends the update registration message to the server.
- Parameters:
-
lifetime,Updated lifetime value in seconds.
- Returns:
- true if sent successfully else false.
Definition at line 253 of file m2mnsdlinterface.cpp.
void stop_timers | ( | ) |
Stops all the timers in case there is any errors.
Definition at line 701 of file m2mnsdlinterface.cpp.
void timer_expired | ( | M2MTimerObserver::Type | type ) | [protected, virtual] |
Indicates that the time has expired.
- Parameters:
-
type,Type of timer that has expired.
Implements M2MTimerObserver.
Definition at line 709 of file m2mnsdlinterface.cpp.
void value_updated | ( | M2MBase * | base, |
const String & | object_name | ||
) | [protected, virtual] |
Callback indicating that the value of the resource object is updated by server.
- Parameters:
-
base,Object whose value is updated. object_name,Name of the resource which is updated, default is empty.
Implements M2MObservationHandler.
Definition at line 741 of file m2mnsdlinterface.cpp.
Generated on Tue Jul 12 2022 18:27:28 by
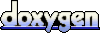