
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
m2minterfaceimpl.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_INTERFACE_IMPL_H 00017 #define M2M_INTERFACE_IMPL_H 00018 00019 #include "mbed-client/m2minterface.h" 00020 #include "mbed-client/m2mserver.h" 00021 #include "mbed-client/m2mconnectionobserver.h" 00022 #include "include/m2mnsdlobserver.h" 00023 #include "mbed-client/m2mtimerobserver.h" 00024 00025 //FORWARD DECLARATION 00026 class M2MNsdlInterface; 00027 class M2MConnectionHandler; 00028 class EventData; 00029 class M2MTimer; 00030 00031 /** 00032 * @brief M2MInterfaceImpl. 00033 * This class implements handling of all mbed Client Interface operations 00034 * defined in OMA LWM2M specifications. 00035 * This includes Bootstrapping, Client Registration, Device Management & 00036 * Service Enablement and Information Reporting. 00037 */ 00038 00039 class M2MInterfaceImpl : public M2MInterface, 00040 public M2MNsdlObserver, 00041 public M2MConnectionObserver, 00042 public M2MTimerObserver 00043 { 00044 private: 00045 // Prevents the use of assignment operator by accident. 00046 M2MInterfaceImpl& operator=( const M2MInterfaceImpl& /*other*/ ); 00047 00048 // Prevents the use of copy constructor by accident 00049 M2MInterfaceImpl( const M2MInterfaceImpl& /*other*/ ); 00050 00051 friend class M2MInterfaceFactory; 00052 00053 private: 00054 00055 /** 00056 * @brief Constructor 00057 * @param observer, Observer to pass the event callbacks for various 00058 * interface operations. 00059 * @param endpoint_name, Endpoint name of the client. 00060 * @param endpoint_type, Endpoint type of the client. 00061 * @param life_time, Life time of the client in seconds 00062 * @param listen_port, Listening port for the endpoint, default is 8000. 00063 * @param domain, Domain of the client. 00064 * @param mode, Binding mode of the client, default is UDP 00065 * @param stack, Network Stack to be used for connection, default is LwIP_IPv4 00066 * @param context_address, Context address default is empty. 00067 */ 00068 M2MInterfaceImpl(M2MInterfaceObserver& observer, 00069 const String &endpoint_name, 00070 const String &endpoint_type, 00071 const int32_t life_time, 00072 const uint16_t listen_port, 00073 const String &domain = "", 00074 BindingMode mode = M2MInterface::NOT_SET, 00075 M2MInterface::NetworkStack stack = M2MInterface::LwIP_IPv4, 00076 const String &context_address = ""); 00077 00078 public: 00079 00080 /** 00081 * @brief Destructor 00082 */ 00083 virtual ~M2MInterfaceImpl(); 00084 00085 /** 00086 * @brief Initiates bootstrapping of the client with the provided Bootstrap 00087 * server information. 00088 * @param security_object, Security object which contains information 00089 * required for successful bootstrapping of the client. 00090 */ 00091 virtual void bootstrap(M2MSecurity *security); 00092 00093 /** 00094 * @brief Cancels on going bootstrapping operation of the client. If the client has 00095 * already successfully bootstrapped then this function deletes existing 00096 * bootstrap information from the client. 00097 */ 00098 virtual void cancel_bootstrap(); 00099 00100 /** 00101 * @brief Initiates registration of the provided Security object to the 00102 * corresponding LWM2M server. 00103 * @param security_object, Security object which contains information 00104 * required for registering to the LWM2M server. 00105 * If client wants to register to multiple LWM2M servers then it has call 00106 * this function once for each of LWM2M server object separately. 00107 * @param object_list, Objects which contains information 00108 * which the client want to register to the LWM2M server. 00109 */ 00110 virtual void register_object(M2MSecurity *security_object, const M2MObjectList &object_list); 00111 00112 /** 00113 * @brief Updates or refreshes the client's registration on the LWM2M 00114 * server. 00115 * @param security_object, Security object from which the device object 00116 * needs to update registration, if there is only one LWM2M server registered 00117 * then this parameter can be NULL. 00118 * @param lifetime, Lifetime for the endpoint client in seconds. 00119 */ 00120 virtual void update_registration(M2MSecurity *security_object, const uint32_t lifetime = 0); 00121 00122 /** 00123 * @brief Unregisters the registered object from the LWM2M server 00124 * @param security_object, Security object from which the device object 00125 * needs to be unregistered, if there is only one LWM2M server registered 00126 * then this parameter can be NULL. 00127 */ 00128 virtual void unregister_object(M2MSecurity* security = NULL); 00129 00130 /** 00131 * @brief Sets the function which will be called indicating client 00132 * is going to sleep when the Binding mode is selected with Queue mode. 00133 * @param callback, Function pointer which will be called when client 00134 * goes to seleep. 00135 */ 00136 virtual void set_queue_sleep_handler(callback_handler handler); 00137 00138 protected: // From M2MNsdlObserver 00139 00140 virtual void coap_message_ready(uint8_t *data_ptr, 00141 uint16_t data_len, 00142 sn_nsdl_addr_s *address_ptr); 00143 00144 virtual void client_registered(M2MServer *server_object); 00145 00146 virtual void registration_updated(const M2MServer &server_object); 00147 00148 virtual void registration_error(uint8_t error_code); 00149 00150 virtual void client_unregistered(); 00151 00152 virtual void bootstrap_done(M2MSecurity *security_object); 00153 00154 virtual void bootstrap_error(); 00155 00156 virtual void coap_data_processed(); 00157 00158 virtual void value_updated(M2MBase *base); 00159 00160 protected: // From M2MConnectionObserver 00161 00162 virtual void data_available(uint8_t* data, 00163 uint16_t data_size, 00164 const M2MConnectionObserver::SocketAddress &address); 00165 00166 virtual void socket_error(uint8_t error_code); 00167 00168 virtual void address_ready(const M2MConnectionObserver::SocketAddress &address, 00169 M2MConnectionObserver::ServerType server_type, 00170 const uint16_t server_port); 00171 00172 virtual void data_sent(); 00173 00174 protected: // from M2MTimerObserver 00175 00176 virtual void timer_expired(M2MTimerObserver::Type type); 00177 00178 00179 private: // state machine state functions 00180 00181 /** 00182 * When the state is Idle. 00183 */ 00184 void state_idle(EventData* data); 00185 00186 /** 00187 * When the client starts bootstrap. 00188 */ 00189 void state_bootstrap( EventData *data); 00190 00191 /** 00192 * When the bootstrap server address is resolved. 00193 */ 00194 void state_bootstrap_address_resolved( EventData *data); 00195 00196 /** 00197 * When the bootstrap resource is created. 00198 */ 00199 void state_bootstrap_resource_created( EventData *data); 00200 00201 /** 00202 * When the server has sent response and bootstrapping is done. 00203 */ 00204 void state_bootstrapped( EventData *data); 00205 00206 /** 00207 * When the client starts register. 00208 */ 00209 void state_register( EventData *data); 00210 00211 /** 00212 * When the server address for register is resolved. 00213 */ 00214 void state_register_address_resolved( EventData *data); 00215 00216 /** 00217 * When the register resource is created. 00218 */ 00219 void state_register_resource_created( EventData *data); 00220 00221 /** 00222 * When the client is registered. 00223 */ 00224 void state_registered( EventData *data); 00225 00226 /** 00227 * When the client is updating registration. 00228 */ 00229 void state_update_registration( EventData *data); 00230 00231 /** 00232 * When the client starts unregister. 00233 */ 00234 void state_unregister( EventData *data); 00235 00236 /** 00237 * When the client has been unregistered. 00238 */ 00239 void state_unregistered( EventData *data); 00240 00241 /** 00242 * When the coap data is been sent through socket. 00243 */ 00244 void state_sending_coap_data( EventData *data); 00245 00246 /** 00247 * When the coap data is sent successfully. 00248 */ 00249 void state_coap_data_sent( EventData *data); 00250 00251 /** 00252 * When the socket is receiving coap data. 00253 */ 00254 void state_receiving_coap_data( EventData *data); 00255 00256 /** 00257 * When the socket has received coap data. 00258 */ 00259 void state_coap_data_received( EventData *data); 00260 00261 /** 00262 * When the coap message is being processed. 00263 */ 00264 void state_processing_coap_data( EventData *data); 00265 00266 /** 00267 * When the coap message has been processed. 00268 */ 00269 void state_coap_data_processed( EventData *data); 00270 00271 /** 00272 * When the client is waiting to receive or send data. 00273 */ 00274 void state_waiting( EventData *data); 00275 00276 /** 00277 * State enumeration order must match the order of state 00278 * method entries in the state map 00279 */ 00280 enum E_States { 00281 STATE_IDLE = 0, 00282 STATE_BOOTSTRAP, 00283 STATE_BOOTSTRAP_ADDRESS_RESOLVED, 00284 STATE_BOOTSTRAP_RESOURCE_CREATED, 00285 STATE_BOOTSTRAPPED, 00286 STATE_REGISTER, //5 00287 STATE_REGISTER_ADDRESS_RESOLVED, 00288 STATE_REGISTER_RESOURCE_CREATED, 00289 STATE_REGISTERED, 00290 STATE_UPDATE_REGISTRATION, 00291 STATE_UNREGISTER, //10 00292 STATE_UNREGISTERED, 00293 STATE_SENDING_COAP_DATA, 00294 STATE_COAP_DATA_SENT, 00295 STATE_COAP_DATA_RECEIVED, 00296 STATE_PROCESSING_COAP_DATA, //15 00297 STATE_COAP_DATA_PROCESSED, 00298 STATE_WAITING, 00299 STATE_MAX_STATES 00300 }; 00301 00302 /** 00303 * @brief Redirects the state machine to right function. 00304 * @param current_state Current state to be set. 00305 * @param data, Data to be passed to the state function. 00306 */ 00307 void state_function( uint8_t current_state, EventData* data ); 00308 00309 /** 00310 * @brief State Engine maintaining state machine logic. 00311 */ 00312 void state_engine(void); 00313 00314 /** 00315 * External event which can trigger the state machine. 00316 * @param New State which the state machine should go to. 00317 * @param data to be passed to the state machine. 00318 */ 00319 void external_event(uint8_t, EventData* = NULL); 00320 00321 /** 00322 * Internal event generated by state machine. 00323 * @param New State which the state machine should go to. 00324 * @param data to be passed to the state machine. 00325 */ 00326 void internal_event(uint8_t, EventData* = NULL); 00327 00328 enum 00329 { 00330 EVENT_IGNORED = 0xFE, 00331 CANNOT_HAPPEN 00332 }; 00333 00334 private: 00335 00336 M2MInterfaceObserver &_observer; 00337 M2MConnectionHandler *_connection_handler; 00338 M2MNsdlInterface *_nsdl_interface; 00339 uint8_t _current_state; 00340 const int _max_states; 00341 bool _event_generated; 00342 EventData *_event_data; 00343 00344 String _endpoint_name; 00345 String _endpoint_type; 00346 String _domain; 00347 int32_t _life_time; 00348 BindingMode _binding_mode; 00349 String _context_address; 00350 uint16_t _listen_port; 00351 M2MSecurity *_register_server; //TODO: to be the list not owned 00352 bool _event_ignored; 00353 bool _register_ongoing; 00354 bool _update_register_ongoing; 00355 M2MTimer *_queue_sleep_timer; 00356 callback_handler _callback_handler; 00357 00358 friend class Test_M2MInterfaceImpl; 00359 00360 }; 00361 00362 #define BEGIN_TRANSITION_MAP \ 00363 static const uint8_t TRANSITIONS[] = {\ 00364 00365 #define TRANSITION_MAP_ENTRY(entry)\ 00366 entry, 00367 00368 #define END_TRANSITION_MAP(data) \ 00369 0 };\ 00370 external_event(TRANSITIONS[_current_state], data); 00371 00372 #endif //M2M_INTERFACE_IMPL_H 00373 00374
Generated on Tue Jul 12 2022 18:27:24 by
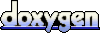