
Embed:
(wiki syntax)
Show/hide line numbers
usrlib.cpp
00001 //****************************************************************************** 00002 //* 00003 //* FULLNAME: Single-Chip Microcontroller Real-Time Operating System 00004 //* 00005 //* NICKNAME: scmRTOS 00006 //* 00007 //* PURPOSE: User Suport Library Source 00008 //* 00009 //* Version: 3.10 00010 //* 00011 //* $Revision: 256 $ 00012 //* $Date:: 2010-01-22 #$ 00013 //* 00014 //* Copyright (c) 2003-2010, Harry E. Zhurov 00015 //* 00016 //* Permission is hereby granted, free of charge, to any person 00017 //* obtaining a copy of this software and associated documentation 00018 //* files (the "Software"), to deal in the Software without restriction, 00019 //* including without limitation the rights to use, copy, modify, merge, 00020 //* publish, distribute, sublicense, and/or sell copies of the Software, 00021 //* and to permit persons to whom the Software is furnished to do so, 00022 //* subject to the following conditions: 00023 //* 00024 //* The above copyright notice and this permission notice shall be included 00025 //* in all copies or substantial portions of the Software. 00026 //* 00027 //* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00028 //* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00029 //* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00030 //* IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY 00031 //* CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, 00032 //* TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH 00033 //* THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00034 //* 00035 //* ================================================================= 00036 //* See http://scmrtos.sourceforge.net for documentation, latest 00037 //* information, license and contact details. 00038 //* ================================================================= 00039 //* 00040 //****************************************************************************** 00041 00042 #include <usrlib.h> 00043 #include <commdefs.h> 00044 00045 using namespace usr; 00046 00047 //------------------------------------------------------------------------------ 00048 // 00049 /// Circular buffer function-member description 00050 // 00051 // 00052 // 00053 TCbuf::TCbuf(byte* const Address, const byte Size) : 00054 buf(Address), 00055 size(Size), 00056 count(0), 00057 first(0), 00058 last(0) 00059 { 00060 } 00061 //------------------------------------------------------------------------------ 00062 bool TCbuf::write(const byte* data, const byte Count) 00063 { 00064 if( Count > (size - count) ) 00065 return false; 00066 00067 for(byte i = 0; i < Count; i++) 00068 push(*(data++)); 00069 00070 return true; 00071 } 00072 //------------------------------------------------------------------------------ 00073 void TCbuf::read(byte* data, const byte Count) 00074 { 00075 byte N = Count <= count ? Count : count; 00076 00077 for(byte i = 0; i < N; i++) 00078 data[i] = pop(); 00079 } 00080 //------------------------------------------------------------------------------ 00081 byte TCbuf::get_byte(const byte index) const 00082 { 00083 byte x = first + index; 00084 00085 if(x < size) 00086 return buf[x]; 00087 else 00088 return buf[x - size]; 00089 } 00090 00091 //------------------------------------------------------------------------------ 00092 bool TCbuf::put(const byte item) 00093 { 00094 if(count == size) 00095 return false; 00096 00097 push(item); 00098 return true; 00099 } 00100 //------------------------------------------------------------------------------ 00101 byte TCbuf::get() 00102 { 00103 if(count) 00104 return pop(); 00105 else 00106 return 0; 00107 } 00108 //------------------------------------------------------------------------------ 00109 // 00110 /// \note 00111 /// For internal purposes. 00112 /// Use this function with care - it doesn't perform free size check. 00113 // 00114 void TCbuf::push(const byte item) 00115 { 00116 buf[last] = item; 00117 last++; 00118 count++; 00119 00120 if(last == size) 00121 last = 0; 00122 } 00123 //------------------------------------------------------------------------------ 00124 // 00125 /// \note 00126 /// For internal purposes. 00127 /// Use this function with care - it doesn't perform free size check. 00128 // 00129 byte TCbuf::pop() 00130 { 00131 byte item = buf[first]; 00132 00133 count--; 00134 first++; 00135 if(first == size) 00136 first = 0; 00137 00138 return item; 00139 } 00140 //------------------------------------------------------------------------------
Generated on Tue Jul 12 2022 21:14:46 by
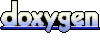