
Embed:
(wiki syntax)
Show/hide line numbers
OS_Target.h
00001 //****************************************************************************** 00002 //* 00003 //* FULLNAME: Single-Chip Microcontroller Real-Time Operating System 00004 //* 00005 //* NICKNAME: scmRTOS 00006 //* 00007 //* PROCESSOR: ARM Cortex-M3 00008 //* 00009 //* TOOLKIT: RVCT (ARM) 00010 //* 00011 //* PURPOSE: Target Dependent Stuff Header. Declarations And Definitions 00012 //* 00013 //* Version: 3.10 00014 //* 00015 //* $Revision: 195 $ 00016 //* $Date:: 2008-06-19 #$ 00017 //* 00018 //* Copyright (c) 2003-2010, Harry E. Zhurov 00019 //* 00020 //* Permission is hereby granted, free of charge, to any person 00021 //* obtaining a copy of this software and associated documentation 00022 //* files (the "Software"), to deal in the Software without restriction, 00023 //* including without limitation the rights to use, copy, modify, merge, 00024 //* publish, distribute, sublicense, and/or sell copies of the Software, 00025 //* and to permit persons to whom the Software is furnished to do so, 00026 //* subject to the following conditions: 00027 //* 00028 //* The above copyright notice and this permission notice shall be included 00029 //* in all copies or substantial portions of the Software. 00030 //* 00031 //* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00032 //* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00033 //* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00034 //* IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY 00035 //* CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, 00036 //* TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH 00037 //* THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00038 //* 00039 //* ================================================================= 00040 //* See http://scmrtos.sourceforge.net for documentation, latest 00041 //* information, license and contact details. 00042 //* ================================================================= 00043 //* 00044 //****************************************************************************** 00045 //* Ported by Andrey Chuikin, Copyright (c) 2008-2010 00046 00047 #ifndef scmRTOS_CORTEXM3_H 00048 #define scmRTOS_CORTEXM3_H 00049 00050 #include <commdefs.h> 00051 00052 //------------------------------------------------------------------------------ 00053 // 00054 // Compiler and Target checks 00055 // 00056 // 00057 #ifndef __ARMCC_VERSION 00058 #error "This file should only be compiled with ARM RVCT Compiler" 00059 #endif // __ARMCC_VERSION 00060 00061 #if __TARGET_ARCH_ARM != 0 || __TARGET_ARCH_THUMB != 4 00062 #error "This file must be compiled for ARMv7-M (Cortex-M3) processor only." 00063 #endif 00064 00065 //------------------------------------------------------------------------------ 00066 // 00067 // Target specific types 00068 // 00069 // 00070 typedef dword TStackItem; 00071 typedef dword TStatusReg; 00072 00073 //----------------------------------------------------------------------------- 00074 // 00075 // Configuration macros 00076 // 00077 // 00078 #define OS_PROCESS __attribute__((__noreturn__)) 00079 #define OS_INTERRUPT 00080 #define DUMMY_INSTR() __NOP() 00081 #define INLINE_PROCESS_CTOR INLINE inline 00082 00083 //----------------------------------------------------------------------------- 00084 // 00085 // Uncomment macro value below for SystemTimer() run in critical section 00086 // 00087 // This is useful (and necessary) when target processor has hardware 00088 // enabled nested interrups. Cortex-M3 have such interrupts. 00089 // 00090 #define SYS_TIMER_CRIT_SECT() TCritSect cs 00091 00092 //----------------------------------------------------------------------------- 00093 // Separate return stack not required 00094 #define SEPARATE_RETURN_STACK 0 00095 00096 //----------------------------------------------------------------------------- 00097 // Software interrupt stack switching not supported in Cortex-M3 port 00098 // because processor implements hardware stack switching. 00099 // So, system timer isr wrapper can't be choosen at project level 00100 // 00101 #define scmRTOS_ISRW_TYPE TISRW 00102 00103 //----------------------------------------------------------------------------- 00104 // 00105 // scmRTOS Context Switch Scheme 00106 // 00107 // The macro defines a context switch manner. Value 0 sets direct context 00108 // switch in the scheduler and in the OS ISRs. This is the primary method. 00109 // Value 1 sets the second way to switch context - by using of software 00110 // interrupt. See documentation fo details. 00111 // Cortex-M3 port supports software interrupt switch method only. 00112 // 00113 #define scmRTOS_CONTEXT_SWITCH_SCHEME 1 00114 00115 //----------------------------------------------------------------------------- 00116 // 00117 // Include project-level configurations 00118 // !!! The order of includes is important !!! 00119 // 00120 #include "../../scmRTOS_config.h" 00121 #include "../scmRTOS_TARGET_CFG.h" 00122 #include <scmRTOS_defs.h> 00123 #include <LPC17xx.h> 00124 00125 //----------------------------------------------------------------------------- 00126 // 00127 // The Critital Section Wrapper 00128 // 00129 // 00130 #define __enable_interrupt() __enable_irq() 00131 #define __disable_interrupt() __disable_irq() 00132 00133 #define __set_interrupt_state(status) __set_PRIMASK(status) 00134 #define __get_interrupt_state() __get_PRIMASK() 00135 00136 class TCritSect 00137 { 00138 public: 00139 TCritSect () : StatusReg(__get_interrupt_state()) { __disable_interrupt(); } 00140 ~TCritSect() { __set_interrupt_state(StatusReg); } 00141 00142 private: 00143 TStatusReg StatusReg; 00144 }; 00145 //----------------------------------------------------------------------------- 00146 00147 //----------------------------------------------------------------------------- 00148 // 00149 // Priority stuff 00150 // 00151 // 00152 namespace OS 00153 { 00154 INLINE inline OS::TProcessMap GetPrioTag(const byte pr) { return static_cast<OS::TProcessMap> (1 << pr); } 00155 00156 #if scmRTOS_PRIORITY_ORDER == 0 00157 INLINE inline byte GetHighPriority(TProcessMap pm) 00158 { 00159 byte pr = 0; 00160 00161 while( !(pm & 0x0001) ) 00162 { 00163 pr++; 00164 pm >>= 1; 00165 } 00166 return pr; 00167 } 00168 #else 00169 INLINE inline byte GetHighPriority(TProcessMap pm) { return (31 - __clz(pm)); } 00170 #endif // scmRTOS_PRIORITY_ORDER 00171 } 00172 00173 //----------------------------------------------------------------------------- 00174 // 00175 // Interrupt and Interrupt Service Routines support 00176 // 00177 INLINE inline TStatusReg GetInterruptState( ) { return __get_interrupt_state(); } 00178 INLINE inline void SetInterruptState(TStatusReg sr) { __set_interrupt_state(sr); } 00179 00180 INLINE inline void EnableInterrupts() { __enable_interrupt(); } 00181 INLINE inline void DisableInterrupts() { __disable_interrupt(); } 00182 00183 00184 namespace OS 00185 { 00186 INLINE inline void EnableContextSwitch() { EnableInterrupts(); } 00187 INLINE inline void DisableContextSwitch() { DisableInterrupts(); } 00188 } 00189 00190 #include <OS_Kernel.h> 00191 00192 namespace OS 00193 { 00194 //-------------------------------------------------------------------------- 00195 // 00196 // NAME : OS ISR support 00197 // 00198 // PURPOSE : Implements common actions on interrupt enter and exit 00199 // under the OS 00200 // 00201 // DESCRIPTION: 00202 // 00203 // 00204 class TISRW 00205 { 00206 public: 00207 INLINE TISRW() { ISR_Enter(); } 00208 INLINE ~TISRW() { ISR_Exit(); } 00209 00210 private: 00211 //----------------------------------------------------- 00212 INLINE void ISR_Enter() 00213 { 00214 TCritSect cs; 00215 Kernel.ISR_NestCount++; 00216 } 00217 //----------------------------------------------------- 00218 INLINE void ISR_Exit() 00219 { 00220 TCritSect cs; 00221 if(--Kernel.ISR_NestCount) return; 00222 Kernel.SchedISR(); 00223 } 00224 //----------------------------------------------------- 00225 }; 00226 00227 // No software interrupt stack switching provided, 00228 // TISRW_SS declared to be the same as TISRW for porting compability 00229 #define TISRW_SS TISRW 00230 00231 } // ns OS 00232 //----------------------------------------------------------------------------- 00233 00234 #endif // scmRTOS_CORTEXM3_H 00235 //----------------------------------------------------------------------------- 00236
Generated on Tue Jul 12 2022 21:14:46 by
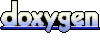