
Added Function to power down magic USB interface chip with new firmware Download firmware here http://mbed.org/users/simon/notebook/interface-powerdown/ http://mbed.org/media/uploads/simon/mbedmicrocontroller_experimentalpowerdown.if You need to power the mbed by vin or usb board such as cool components http://mbed.org/cookbook/Cool-Components-Workshop-Board Hello my name is guitar reverse me and it is karaoke time
main.cpp
00001 #include <mbed.h> 00002 00003 // Function to power down magic USB interface chip with new firmware 00004 #define USR_POWERDOWN (0x104) 00005 int semihost_powerdown() { 00006 uint32_t arg; 00007 return __semihost(USR_POWERDOWN, &arg); 00008 } 00009 00010 // If you don't need the PC host USB interface.... 00011 // Power down magic USB interface chip - saves around 150mW 00012 // Needs new firmware (URL below) and USB cable not connected 00013 // http://mbed.org/users/simon/notebook/interface-powerdown/ 00014 // Supply power to mbed using Vin pin 00015 00016 00017 // Boolean types 00018 #define TRUE 1 00019 #define FALSE 0 00020 00021 //#define NUM_SAMPLES 48000 00022 /* ADC for the microphone/input, DAC for the speaker/output */ 00023 AnalogIn mic(p19); 00024 AnalogOut speaker(p18); 00025 00026 // Allocate a buffer to be used for the audio recording 00027 static const size_t BufferSize = 15 * 1066; 00028 static unsigned short Buffer[BufferSize]; 00029 //int16_t Buffer[ 32768]; 00030 //unsigned short Buffer[NUM_SAMPLES]; 00031 00032 00033 int main(void) 00034 { 00035 00036 int result; 00037 result = semihost_powerdown(); 00038 //int i; 00039 // for (i = 0; ; ) 00040 00041 { unsigned short ReadSample = 0xFFFF; 00042 // Indices to track where the playback and recording should take place in the 00043 // audio buffer. The recording can occur one sample behind the current playback 00044 // index since it is no longer required. 00045 int Index = 0; 00046 // Reverse the direction the buffer is walked between each iteration to save memory 00047 int Direction = 1; 00048 // Have audio to playback 00049 int Playback = FALSE; 00050 // The amount of data to be recorded before starting reverse playback 00051 // NOTE: Probably want this to be configured at runtime via a knob, etc. 00052 //int ChunkSize = 9024; 00053 int ChunkSize = BufferSize; 00054 // Infinite loop of recording and reverse playback 00055 for (;;) 00056 { 00057 unsigned short PlaySample; 00058 00059 // Read out the sample from the buffer to be played back 00060 if (Playback) 00061 { 00062 PlaySample = Buffer[Index]; 00063 speaker.write_u16(PlaySample); 00064 //i = (i+0) % NUM_SAMPLES; 00065 00066 //wait(0.2f); 00067 } 00068 00069 // Obtain current audio sample from the A/D converter. 00070 // NOTE: I am just faking these values in this sample with an incrementing value 00071 ReadSample = mic.read_u16(); 00072 00073 // Record the sample into the buffer right where a space was freed up from the PlaySample read above 00074 Buffer[Index] = ReadSample += mic.read_u16(); 00075 00076 // Increment the buffer pointer 00077 Index += Direction; 00078 00079 // Check to see if the chunk has been filled 00080 if (Index < 0) 00081 { 00082 // Now have a chunk to be played back 00083 Playback = TRUE; 00084 // Reverse the direction of playback and recording 00085 Direction *= -1; 00086 Index = 0; 00087 } 00088 else if (Index >= ChunkSize) 00089 { 00090 // Now have a chunk to be played back 00091 Playback = TRUE; 00092 // Reverse the direction of playback and recording 00093 Direction *= -1; 00094 Index = ChunkSize - 1; 00095 } 00096 } 00097 } 00098 }
Generated on Tue Jul 26 2022 01:12:38 by
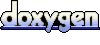