mbed library sources. Supersedes mbed-src. Fixed broken STM32F1xx RTC on rtc_api.c
Dependents: Nucleo_F103RB_RTC_battery_bkup_pwr_off_okay
Fork of mbed-dev by
Platform
Data Structures | |
class | Callback< R()> |
Callback class based on template specialization. More... | |
class | Callback< R(A0)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1, A2)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1, A2, A3)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1, A2, A3, A4)> |
Callback class based on template specialization. More... | |
class | CircularBuffer< T, BufferSize, CounterType > |
Templated Circular buffer class. More... | |
class | CThunk< T > |
Class for created a pointer with data bound to it. More... | |
class | DirHandle |
Represents a directory stream. More... | |
class | FileHandle |
Class FileHandle. More... | |
class | FileSystemLike |
A filesystem-like object is one that can be used to open file-like objects though it by fopen("/name/filename", mode) More... | |
class | LocalFileSystem |
A filesystem for accessing the local mbed Microcontroller USB disk drive. More... | |
class | PlatformMutex |
A stub mutex for when an RTOS is not present. More... | |
struct | SingletonPtr< T > |
Utility class for creating an using a singleton. More... | |
class | Stream |
File stream. More... | |
struct | transaction_t |
Transaction structure. More... | |
class | Transaction< Class > |
Transaction class defines a transaction. More... | |
Typedefs | |
typedef Callback< void()> * | pFunctionPointer_t |
Group one or more functions in an instance of a CallChain, then call them in sequence using CallChain::call(). | |
typedef void(* | mbed_mem_trace_cb_t )(uint8_t op, void *res, void *caller,...) |
Type of the callback used by the memory tracer. | |
Functions | |
void | mbed_start_application (uintptr_t address) |
Start the application at the given address. | |
void | mbed_assert_internal (const char *expr, const char *file, int line) |
Internal mbed assert function which is invoked when MBED_ASSERT macro failes. | |
bool | core_util_are_interrupts_enabled (void) |
Determine the current interrupts enabled state. | |
bool | core_util_is_isr_active (void) |
Determine if this code is executing from an interrupt. | |
void | core_util_critical_section_enter (void) |
Mark the start of a critical section. | |
void | core_util_critical_section_exit (void) |
Mark the end of a critical section. | |
bool | core_util_atomic_cas_u8 (uint8_t *ptr, uint8_t *expectedCurrentValue, uint8_t desiredValue) |
Atomic compare and set. | |
bool | core_util_atomic_cas_u16 (uint16_t *ptr, uint16_t *expectedCurrentValue, uint16_t desiredValue) |
Atomic compare and set. | |
bool | core_util_atomic_cas_u32 (uint32_t *ptr, uint32_t *expectedCurrentValue, uint32_t desiredValue) |
Atomic compare and set. | |
bool | core_util_atomic_cas_ptr (void **ptr, void **expectedCurrentValue, void *desiredValue) |
Atomic compare and set. | |
uint8_t | core_util_atomic_incr_u8 (uint8_t *valuePtr, uint8_t delta) |
Atomic increment. | |
uint16_t | core_util_atomic_incr_u16 (uint16_t *valuePtr, uint16_t delta) |
Atomic increment. | |
uint32_t | core_util_atomic_incr_u32 (uint32_t *valuePtr, uint32_t delta) |
Atomic increment. | |
void * | core_util_atomic_incr_ptr (void **valuePtr, ptrdiff_t delta) |
Atomic increment. | |
uint8_t | core_util_atomic_decr_u8 (uint8_t *valuePtr, uint8_t delta) |
Atomic decrement. | |
uint16_t | core_util_atomic_decr_u16 (uint16_t *valuePtr, uint16_t delta) |
Atomic decrement. | |
uint32_t | core_util_atomic_decr_u32 (uint32_t *valuePtr, uint32_t delta) |
Atomic decrement. | |
void * | core_util_atomic_decr_ptr (void **valuePtr, ptrdiff_t delta) |
Atomic decrement. | |
static void | debug (const char *format,...) |
Output a debug message. | |
static void | debug_if (int condition, const char *format,...) |
Conditionally output a debug message. | |
void | error (const char *format,...) |
To generate a fatal compile-time error, you can use the pre-processor error directive. | |
int | mbed_interface_connected (void) |
Functions to control the mbed interface. | |
int | mbed_interface_reset (void) |
Instruct the mbed interface to reset, as if the reset button had been pressed. | |
int | mbed_interface_disconnect (void) |
This will disconnect the debug aspect of the interface, so semihosting will be disabled. | |
int | mbed_interface_powerdown (void) |
This will disconnect the debug aspect of the interface, and if the USB cable is not connected, also power down the interface. | |
int | mbed_interface_uid (char *uid) |
This returns a string containing the 32-character UID of the mbed interface This is a weak function that can be overwritten if required. | |
void | mbed_mac_address (char *mac) |
This returns a unique 6-byte MAC address, based on the interface UID If the interface is not present, it returns a default fixed MAC address (00:02:F7:F0:00:00) | |
void | mbed_die (void) |
Cause the mbed to flash the BLOD (Blue LEDs Of Death) sequence. | |
void | mbed_error_printf (const char *format,...) |
Print out an error message. | |
void | mbed_error_vfprintf (const char *format, va_list arg) |
Print out an error message. | |
void | mbed_mem_trace_set_callback (mbed_mem_trace_cb_t cb) |
Set the callback used by the memory tracer (use NULL for disable tracing). | |
void * | mbed_mem_trace_malloc (void *res, size_t size, void *caller) |
Trace a call to 'malloc'. | |
void * | mbed_mem_trace_realloc (void *res, void *ptr, size_t size, void *caller) |
Trace a call to 'realloc'. | |
void * | mbed_mem_trace_calloc (void *res, size_t num, size_t size, void *caller) |
Trace a call to 'calloc'. | |
void | mbed_mem_trace_free (void *ptr, void *caller) |
Trace a call to 'free'. | |
void | mbed_mem_trace_default_callback (uint8_t op, void *res, void *caller,...) |
Default memory trace callback. | |
bool | _rtc_is_leap_year (int year) |
Compute if a year is a leap year or not. | |
void | set_time (time_t t) |
Implementation of the C time.h functions. | |
void | attach_rtc (time_t(*read_rtc)(void), void(*write_rtc)(time_t), void(*init_rtc)(void), int(*isenabled_rtc)(void)) |
Attach an external RTC to be used for the C time functions. | |
void | sleep_manager_lock_deep_sleep (void) |
Sleep manager API The sleep manager provides API to automatically select sleep mode. | |
void | sleep_manager_unlock_deep_sleep (void) |
Unlock the deep sleep mode. | |
bool | sleep_manager_can_deep_sleep (void) |
Get the status of deep sleep allowance for a target. | |
void | sleep_manager_sleep_auto (void) |
Enter auto selected sleep mode. | |
static __INLINE void | sleep (void) |
Send the microcontroller to sleep. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.6","One entry point for an application, use sleep()") __INLINE static void deepsleep(void) | |
Send the microcontroller to deep sleep. | |
void | mbed_stats_heap_get (mbed_stats_heap_t *stats) |
Fill the passed in heap stat structure with heap stats. | |
void | mbed_stats_stack_get (mbed_stats_stack_t *stats) |
Fill the passed in structure with stack stats accumulated for all threads. | |
size_t | mbed_stats_stack_get_each (mbed_stats_stack_t *stats, size_t count) |
Fill the passed array of stat structures with the stack stats for each available thread. | |
void | wait (float s) |
Generic wait functions. | |
void | wait_ms (int ms) |
Waits a number of milliseconds. | |
void | wait_us (int us) |
Waits a number of microseconds. | |
static void | singleton_lock (void) |
Lock the singleton mutex. | |
static void | singleton_unlock (void) |
Unlock the singleton mutex. |
Typedef Documentation
typedef void(* mbed_mem_trace_cb_t)(uint8_t op, void *res, void *caller,...) |
Type of the callback used by the memory tracer.
This callback is called when a memory allocation operation (malloc, realloc, calloc, free) is called and tracing is enabled for that memory allocation function.
- Parameters:
-
op the ID of the operation (MBED_MEM_TRACE_MALLOC, MBED_MEM_TRACE_REALLOC, MBED_MEM_TRACE_CALLOC or MBED_MEM_TRACE_FREE). res the result that the memory operation returned (NULL for 'free'). caller the caller of the memory operation. Note that the value of 'caller' might be unreliable.
The rest of the parameters passed 'mbed_mem_trace_cb_t' are the same as the memory operations that triggered its call (see 'man malloc' for details):
- for malloc: cb(MBED_MEM_TRACE_MALLOC, res, caller, size).
- for realloc: cb(MBED_MEM_TRACE_REALLOC, res, caller, ptr, size).
- for calloc: cb(MBED_MEM_TRACE_CALLOC, res, caller, nmemb, size).
- for free: cb(MBED_MEM_TRACE_FREE, NULL, caller, ptr).
Definition at line 60 of file mbed_mem_trace.h.
typedef Callback<void()>* pFunctionPointer_t |
Group one or more functions in an instance of a CallChain, then call them in sequence using CallChain::call().
Used mostly by the interrupt chaining code, but can be used for other purposes.
- Note:
- Synchronization level: Not protected
Example:
#include "mbed.h" CallChain chain; void first(void) { printf("'first' function.\n"); } void second(void) { printf("'second' function.\n"); } class Test { public: void f(void) { printf("A::f (class member).\n"); } }; int main() { Test test; chain.add(second); chain.add_front(first); chain.add(&test, &Test::f); chain.call(); }
Definition at line 66 of file CallChain.h.
Function Documentation
bool _rtc_is_leap_year | ( | int | year ) |
Compute if a year is a leap year or not.
- Parameters:
-
year The year to test it shall be in the range [70:138]. Year 0 is translated into year 1900 CE.
- Returns:
- true if the year in input is a leap year and false otherwise.
- Note:
- - For use by the HAL only
Definition at line 66 of file mbed_mktime.c.
void attach_rtc | ( | time_t(*)(void) | read_rtc, |
void(*)(time_t) | write_rtc, | ||
void(*)(void) | init_rtc, | ||
int(*)(void) | isenabled_rtc | ||
) |
Attach an external RTC to be used for the C time functions.
- Note:
- Synchronization level: Thread safe
- Parameters:
-
read_rtc pointer to function which returns current UNIX timestamp write_rtc pointer to function which sets current UNIX timestamp, can be NULL init_rtc pointer to funtion which initializes RTC, can be NULL isenabled_rtc pointer to function wich returns if the rtc is enabled, can be NULL
Definition at line 77 of file mbed_rtc_time.cpp.
bool core_util_are_interrupts_enabled | ( | void | ) |
Determine the current interrupts enabled state.
This function can be called to determine whether or not interrupts are currently enabled.
- Note:
- NOTE: This function works for both cortex-A and cortex-M, although the underlyng implementation differs.
- Returns:
- true if interrupts are enabled, false otherwise
Definition at line 29 of file mbed_critical.c.
bool core_util_atomic_cas_ptr | ( | void ** | ptr, |
void ** | expectedCurrentValue, | ||
void * | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value.
- Note:
- : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value.
- Parameters:
-
[in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
- Note:
- : In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is still updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
Definition at line 322 of file mbed_critical.c.
bool core_util_atomic_cas_u16 | ( | uint16_t * | ptr, |
uint16_t * | expectedCurrentValue, | ||
uint16_t | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value.
- Note:
- : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value.
- Parameters:
-
[in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
- Note:
- : In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is still updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
Definition at line 123 of file mbed_critical.c.
bool core_util_atomic_cas_u32 | ( | uint32_t * | ptr, |
uint32_t * | expectedCurrentValue, | ||
uint32_t | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value.
- Note:
- : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value.
- Parameters:
-
[in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
- Note:
- : In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is still updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
Definition at line 136 of file mbed_critical.c.
bool core_util_atomic_cas_u8 | ( | uint8_t * | ptr, |
uint8_t * | expectedCurrentValue, | ||
uint8_t | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value.
- Note:
- : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value.
- Parameters:
-
[in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
- Note:
- : In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is still updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
Definition at line 111 of file mbed_critical.c.
void* core_util_atomic_decr_ptr | ( | void ** | valuePtr, |
ptrdiff_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented in bytes.
- Returns:
- The new decremented value.
- Note:
- The type of the pointer argument is not taken into account and the pointer is decremented by bytes
Definition at line 333 of file mbed_critical.c.
uint16_t core_util_atomic_decr_u16 | ( | uint16_t * | valuePtr, |
uint16_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented.
- Returns:
- The new decremented value.
Definition at line 185 of file mbed_critical.c.
uint32_t core_util_atomic_decr_u32 | ( | uint32_t * | valuePtr, |
uint32_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented.
- Returns:
- The new decremented value.
Definition at line 194 of file mbed_critical.c.
uint8_t core_util_atomic_decr_u8 | ( | uint8_t * | valuePtr, |
uint8_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented.
- Returns:
- The new decremented value.
Definition at line 176 of file mbed_critical.c.
void* core_util_atomic_incr_ptr | ( | void ** | valuePtr, |
ptrdiff_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented in bytes.
- Returns:
- The new incremented value.
- Note:
- The type of the pointer argument is not taken into account and the pointer is incremented by bytes.
Definition at line 329 of file mbed_critical.c.
uint16_t core_util_atomic_incr_u16 | ( | uint16_t * | valuePtr, |
uint16_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented.
- Returns:
- The new incremented value.
Definition at line 157 of file mbed_critical.c.
uint32_t core_util_atomic_incr_u32 | ( | uint32_t * | valuePtr, |
uint32_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented.
- Returns:
- The new incremented value.
Definition at line 166 of file mbed_critical.c.
uint8_t core_util_atomic_incr_u8 | ( | uint8_t * | valuePtr, |
uint8_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented.
- Returns:
- The new incremented value.
Definition at line 148 of file mbed_critical.c.
void core_util_critical_section_enter | ( | void | ) |
Mark the start of a critical section.
This function should be called to mark the start of a critical section of code.
- Note:
- NOTES: 1) The use of this style of critical section is targetted at C based implementations. 2) These critical sections can be nested. 3) The interrupt enable state on entry to the first critical section (of a nested set, or single section) will be preserved on exit from the section. 4) This implementation will currently only work on code running in privileged mode.
Definition at line 54 of file mbed_critical.c.
void core_util_critical_section_exit | ( | void | ) |
Mark the end of a critical section.
This function should be called to mark the end of a critical section of code.
- Note:
- NOTES: 1) The use of this style of critical section is targetted at C based implementations. 2) These critical sections can be nested. 3) The interrupt enable state on entry to the first critical section (of a nested set, or single section) will be preserved on exit from the section. 4) This implementation will currently only work on code running in privileged mode.
Definition at line 79 of file mbed_critical.c.
bool core_util_is_isr_active | ( | void | ) |
Determine if this code is executing from an interrupt.
This function can be called to determine if the code is running on interrupt context.
- Note:
- NOTE: This function works for both cortex-A and cortex-M, although the underlyng implementation differs.
- Returns:
- true if in an isr, false otherwise
Definition at line 38 of file mbed_critical.c.
static void debug | ( | const char * | format, |
... | |||
) | [static] |
Output a debug message.
- Parameters:
-
format printf-style format string, followed by variables
Definition at line 35 of file mbed_debug.h.
static void debug_if | ( | int | condition, |
const char * | format, | ||
... | |||
) | [static] |
Conditionally output a debug message.
NOTE: If the condition is constant false (== 0) and the compiler optimization level is greater than 0, then the whole function will be compiled away.
- Parameters:
-
condition output only if condition is true (!= 0) format printf-style format string, followed by variables
Definition at line 53 of file mbed_debug.h.
void error | ( | const char * | format, |
... | |||
) |
To generate a fatal compile-time error, you can use the pre-processor error directive.
- Parameters:
-
format C string that contains data stream to be printed. Code snippets below show valid format.
#error "That shouldn't have happened!"
If the compiler evaluates this line, it will report the error and stop the compile.
For example, you could use this to check some user-defined compile-time variables:
#define NUM_PORTS 7 #if (NUM_PORTS > 4) #error "NUM_PORTS must be less than 4" #endif
Reporting Run-Time Errors: To generate a fatal run-time error, you can use the mbed error() function.
error("That shouldn't have happened!");
If the mbed running the program executes this function, it will print the message via the USB serial port, and then die with the blue lights of death!
The message can use printf-style formatting, so you can report variables in the message too. For example, you could use this to check a run-time condition:
if(x >= 5) { error("expected x to be less than 5, but got %d", x); }
Definition at line 28 of file mbed_error.c.
void mbed_assert_internal | ( | const char * | expr, |
const char * | file, | ||
int | line | ||
) |
Internal mbed assert function which is invoked when MBED_ASSERT macro failes.
This function is active only if NDEBUG is not defined prior to including this assert header file. In case of MBED_ASSERT failing condition, error() is called with the assertation message.
- Parameters:
-
expr Expresion to be checked. file File where assertation failed. line Failing assertation line number.
Definition at line 22 of file mbed_assert.c.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.6" | , |
"One entry point for an | application, | ||
use sleep()" | |||
) |
Send the microcontroller to deep sleep.
- Note:
- This function can be a noop if not implemented by the platform.
- This function will be a noop in debug mode (debug build profile when MBED_DEBUG is defined)
- This function will be a noop while uVisor is in use.
This processor is setup ready for deep sleep, and sent to sleep. This mode has the same sleep features as sleep plus it powers down peripherals and clocks. All state is still maintained.
The processor can only be woken up by an external interrupt on a pin or a watchdog timer.
- Note:
- The mbed interface semihosting is disconnected as part of going to sleep, and can not be restored. Flash re-programming and the USB serial port will remain active, but the mbed program will no longer be able to access the LocalFileSystem
Definition at line 155 of file mbed_sleep.h.
void mbed_die | ( | void | ) |
Cause the mbed to flash the BLOD (Blue LEDs Of Death) sequence.
Definition at line 29 of file mbed_board.c.
void mbed_error_printf | ( | const char * | format, |
... | |||
) |
Print out an error message.
This is typically called when handling a crash.
- Note:
- Synchronization level: Interrupt safe
- Parameters:
-
format C string that contains data stream to be printed. Code snippets below show valid format.
mbed_error_printf("Failed: %s, file: %s, line %d \n", expr, file, line);
Definition at line 52 of file mbed_board.c.
void mbed_error_vfprintf | ( | const char * | format, |
va_list | arg | ||
) |
Print out an error message.
Similar to mbed_error_printf but uses a va_list.
- Note:
- Synchronization level: Interrupt safe
- Parameters:
-
format C string that contains data stream to be printed. arg Variable arguments list
Definition at line 59 of file mbed_board.c.
int mbed_interface_connected | ( | void | ) |
Functions to control the mbed interface.
mbed Microcontrollers have a built-in interface to provide functionality such as drag-n-drop download, reset, serial-over-usb, and access to the mbed local file system. These functions provide means to control the interface suing semihost calls it supports. Determine whether the mbed interface is connected, based on whether debug is enabled
- Returns:
- 1 if interface is connected, 0 otherwise
Definition at line 28 of file mbed_interface.c.
int mbed_interface_disconnect | ( | void | ) |
This will disconnect the debug aspect of the interface, so semihosting will be disabled.
The interface will still support the USB serial aspect
- Returns:
- 0 if successful, -1 otherwise (e.g. interface not present)
Definition at line 50 of file mbed_interface.c.
int mbed_interface_powerdown | ( | void | ) |
This will disconnect the debug aspect of the interface, and if the USB cable is not connected, also power down the interface.
If the USB cable is connected, the interface will remain powered up and visible to the host
- Returns:
- 0 if successful, -1 otherwise (e.g. interface not present)
Definition at line 62 of file mbed_interface.c.
int mbed_interface_reset | ( | void | ) |
Instruct the mbed interface to reset, as if the reset button had been pressed.
- Returns:
- 1 if successful, 0 otherwise (e.g. interface not present)
Definition at line 32 of file mbed_interface.c.
int mbed_interface_uid | ( | char * | uid ) |
This returns a string containing the 32-character UID of the mbed interface This is a weak function that can be overwritten if required.
- Parameters:
-
uid A 33-byte array to write the null terminated 32-byte string
- Returns:
- 0 if successful, -1 otherwise (e.g. interface not present)
Definition at line 41 of file mbed_interface.c.
void mbed_mac_address | ( | char * | mac ) |
This returns a unique 6-byte MAC address, based on the interface UID If the interface is not present, it returns a default fixed MAC address (00:02:F7:F0:00:00)
This is a weak function that can be overwritten if you want to provide your own mechanism to provide a MAC address.
- Parameters:
-
mac A 6-byte array to write the MAC address
Definition at line 84 of file mbed_interface.c.
void* mbed_mem_trace_calloc | ( | void * | res, |
size_t | num, | ||
size_t | size, | ||
void * | caller | ||
) |
Trace a call to 'calloc'.
- Parameters:
-
res the result of running 'calloc'. num the 'nmemb' argument given to 'calloc'. size the 'size' argument given to 'calloc'. caller the caller of the memory operation.
- Returns:
- 'res' (the first argument).
Definition at line 62 of file mbed_mem_trace.c.
void mbed_mem_trace_default_callback | ( | uint8_t | op, |
void * | res, | ||
void * | caller, | ||
... | |||
) |
Default memory trace callback.
DO NOT CALL DIRECTLY. It is meant to be used as the second argument of 'mbed_mem_trace_setup'.
The default callback outputs trace data using 'printf', in a format that's easily parsable by an external tool. For each memory operation, the callback outputs a line that begins with "#<op>:<0xresult>;<0xcaller>-":
- Parameters:
-
op identifies the memory operation ('m' for 'malloc', 'r' for 'realloc', 'c' for 'calloc' and 'f' for 'free'). res (base 16) is the result of the memor operation. This is always NULL for 'free', since 'free' doesn't return anything. caller (base 16) is the caller of the memory operation. Note that the value of 'caller' might be unreliable.
The rest of the output depends on the operation being traced:
- for 'malloc': 'size', where 'size' is the original argument to 'malloc'.
- for 'realloc': '0xptr;size', where 'ptr' (base 16) and 'size' are the original arguments to 'realloc'.
- for 'calloc': 'nmemb;size', where 'nmemb' and 'size' are the original arguments to 'calloc'.
- for 'free': '0xptr', where 'ptr' (base 16) is the original argument to 'free'.
Examples:
- "#m:0x20003240;0x600d-50" encodes a 'malloc' that returned 0x20003240, was called by the instruction at 0x600D with a the 'size' argument equal to 50.
- "#f:0x0;0x602f-0x20003240" encodes a 'free' that was called by the instruction at 0x602f with the 'ptr' argument equal to 0x20003240.
Definition at line 81 of file mbed_mem_trace.c.
void mbed_mem_trace_free | ( | void * | ptr, |
void * | caller | ||
) |
Trace a call to 'free'.
- Parameters:
-
ptr the 'ptr' argument given to 'free'. caller the caller of the memory operation.
Definition at line 72 of file mbed_mem_trace.c.
void* mbed_mem_trace_malloc | ( | void * | res, |
size_t | size, | ||
void * | caller | ||
) |
Trace a call to 'malloc'.
- Parameters:
-
res the result of running 'malloc'. size the 'size' argument given to 'malloc'. caller the caller of the memory operation.
- Returns:
- 'res' (the first argument).
Definition at line 42 of file mbed_mem_trace.c.
void* mbed_mem_trace_realloc | ( | void * | res, |
void * | ptr, | ||
size_t | size, | ||
void * | caller | ||
) |
Trace a call to 'realloc'.
- Parameters:
-
res the result of running 'realloc'. ptr the 'ptr' argument given to 'realloc'. size the 'size' argument given to 'realloc'. caller the caller of the memory operation.
- Returns:
- 'res' (the first argument).
Definition at line 52 of file mbed_mem_trace.c.
void mbed_mem_trace_set_callback | ( | mbed_mem_trace_cb_t | cb ) |
Set the callback used by the memory tracer (use NULL for disable tracing).
- Parameters:
-
cb the callback to call on each memory operation.
Definition at line 38 of file mbed_mem_trace.c.
void mbed_start_application | ( | uintptr_t | address ) |
Start the application at the given address.
This function does not return. It is the applications responsibility for flushing to or powering down external components such as filesystems or socket connections before calling this function. For Cortex-M devices this function powers down generic system components such as the NVIC and set the vector table to that of the new image followed by jumping to the reset handler of the new image.
- Parameters:
-
address Starting address of next application to run
Definition at line 28 of file mbed_application.c.
void mbed_stats_heap_get | ( | mbed_stats_heap_t * | stats ) |
Fill the passed in heap stat structure with heap stats.
- Parameters:
-
stats A pointer to the mbed_stats_heap_t structure to fill
Definition at line 57 of file mbed_alloc_wrappers.cpp.
void mbed_stats_stack_get | ( | mbed_stats_stack_t * | stats ) |
Fill the passed in structure with stack stats accumulated for all threads.
The thread_id will be 0 and stack_cnt will represent number of threads.
- Parameters:
-
stats A pointer to the mbed_stats_stack_t structure to fill
Definition at line 12 of file mbed_stats.c.
size_t mbed_stats_stack_get_each | ( | mbed_stats_stack_t * | stats, |
size_t | count | ||
) |
Fill the passed array of stat structures with the stack stats for each available thread.
- Parameters:
-
stats A pointer to an array of mbed_stats_stack_t structures to fill count The number of mbed_stats_stack_t structures in the provided array
- Returns:
- The number of mbed_stats_stack_t structures that have been filled, this is equal to the number of stacks on the system.
Definition at line 39 of file mbed_stats.c.
void set_time | ( | time_t | t ) |
Implementation of the C time.h functions.
Provides mechanisms to set and read the current time, based on the microcontroller Real-Time Clock (RTC), plus some standard C manipulation and formating functions.
Example:
#include "mbed.h" int main() { set_time(1256729737); // Set RTC time to Wed, 28 Oct 2009 11:35:37 while(1) { time_t seconds = time(NULL); printf("Time as seconds since January 1, 1970 = %d\n", seconds); printf("Time as a basic string = %s", ctime(&seconds)); char buffer[32]; strftime(buffer, 32, "%I:%M %p\n", localtime(&seconds)); printf("Time as a custom formatted string = %s", buffer); wait(1); } }
Set the current time
Initialises and sets the time of the microcontroller Real-Time Clock (RTC) to the time represented by the number of seconds since January 1, 1970 (the UNIX timestamp).
- Parameters:
-
t Number of seconds since January 1, 1970 (the UNIX timestamp)
- Note:
- Synchronization level: Thread safe
Example:
#include "mbed.h" int main() { set_time(1256729737); // Set time to Wed, 28 Oct 2009 11:35:37 }
Definition at line 66 of file mbed_rtc_time.cpp.
static void singleton_lock | ( | void | ) | [static] |
Lock the singleton mutex.
This function is typically used to provide exclusive access when initializing a global object.
Definition at line 39 of file SingletonPtr.h.
static void singleton_unlock | ( | void | ) | [static] |
Unlock the singleton mutex.
This function is typically used to provide exclusive access when initializing a global object.
Definition at line 52 of file SingletonPtr.h.
static __INLINE void sleep | ( | void | ) | [static] |
Send the microcontroller to sleep.
- Note:
- This function can be a noop if not implemented by the platform.
- This function will be a noop in debug mode (debug build profile when MBED_DEBUG is defined).
- This function will be a noop while uVisor is in use.
-
This function will be a noop if the following conditions are met:
- The RTOS is present
- The processor turn off the Systick clock during sleep
- The target does not implement tickless mode
The processor is setup ready for sleep, and sent to sleep using __WFI(). In this mode, the system clock to the core is stopped until a reset or an interrupt occurs. This eliminates dynamic power used by the processor, memory systems and buses. The processor, peripheral and memory state are maintained, and the peripherals continue to work and can generate interrupts.
The processor can be woken up by any internal peripheral interrupt or external pin interrupt.
- Note:
- The mbed interface semihosting is disconnected as part of going to sleep, and can not be restored. Flash re-programming and the USB serial port will remain active, but the mbed program will no longer be able to access the LocalFileSystem
Definition at line 126 of file mbed_sleep.h.
bool sleep_manager_can_deep_sleep | ( | void | ) |
Get the status of deep sleep allowance for a target.
- Returns:
- true if a target can go to deepsleep, false otherwise
Definition at line 50 of file mbed_sleep_manager.c.
void sleep_manager_lock_deep_sleep | ( | void | ) |
Sleep manager API The sleep manager provides API to automatically select sleep mode.
There are two sleep modes:
- sleep
- deepsleep
Use locking/unlocking deepsleep for drivers that depend on features that are not allowed (=disabled) during the deepsleep. For instance, high frequency clocks.
Example:
void driver::handler() { if (_sensor.get_event()) { // any event - we are finished, unlock the deepsleep sleep_manager_unlock_deep_sleep(); _callback(); } } int driver::measure(event_t event, callback_t& callback) { _callback = callback; sleep_manager_lock_deep_sleep(); // start async transaction, we are waiting for an event return _sensor.start(event, callback); }
Lock the deep sleep mode
This locks the automatic deep mode selection. sleep_manager_sleep_auto() will ignore deepsleep mode if this function is invoked at least once (the internal counter is non-zero)
Use this locking mechanism for interrupt driven API that are running in the background and deepsleep could affect their functionality
The lock is a counter, can be locked up to USHRT_MAX This function is IRQ and thread safe
Definition at line 28 of file mbed_sleep_manager.c.
void sleep_manager_sleep_auto | ( | void | ) |
Enter auto selected sleep mode.
It chooses the sleep or deeepsleep modes based on the deepsleep locking counter
This function is IRQ and thread safe
- Note:
- If MBED_DEBUG is defined, only hal_sleep is allowed. This ensures the debugger to be active for debug modes.
Definition at line 55 of file mbed_sleep_manager.c.
void sleep_manager_unlock_deep_sleep | ( | void | ) |
Unlock the deep sleep mode.
Use unlocking in pair with sleep_manager_lock_deep_sleep().
The lock is a counter, should be equally unlocked as locked This function is IRQ and thread safe
Definition at line 39 of file mbed_sleep_manager.c.
void wait | ( | float | s ) |
Generic wait functions.
These provide simple NOP type wait capabilities.
Example:
#include "mbed.h" DigitalOut heartbeat(LED1); int main() { while (1) { heartbeat = 1; wait(0.5); heartbeat = 0; wait(0.5); } }
Waits for a number of seconds, with microsecond resolution (within the accuracy of single precision floating point).
- Parameters:
-
s number of seconds to wait
Definition at line 24 of file mbed_wait_api_no_rtos.c.
void wait_ms | ( | int | ms ) |
Waits a number of milliseconds.
- Parameters:
-
ms the whole number of milliseconds to wait
Definition at line 28 of file mbed_wait_api_no_rtos.c.
void wait_us | ( | int | us ) |
Waits a number of microseconds.
- Parameters:
-
us the whole number of microseconds to wait
Definition at line 32 of file mbed_wait_api_no_rtos.c.
Generated on Thu Jul 14 2022 19:34:40 by
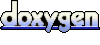