mbed library sources. Supersedes mbed-src. Fixed broken STM32F1xx RTC on rtc_api.c
Dependents: Nucleo_F103RB_RTC_battery_bkup_pwr_off_okay
Fork of mbed-dev by
NonCopyable< T > Class Template Reference
Inheriting from this class autogeneration of copy construction and copy assignement operations. More...
#include <NonCopyable.h>
Protected Member Functions | |
NonCopyable () | |
Disalow construction of NonCopyable objects from outside of its hierarchy. | |
~NonCopyable () | |
Disalow destruction of NonCopyable objects from outside of its hierarchy. |
Detailed Description
template<typename T>
class mbed::NonCopyable< T >
Inheriting from this class autogeneration of copy construction and copy assignement operations.
Classes which are not value type should inherit privately from this class to avoid generation of invalid copy constructor or copy assignement operator which can lead to unoticeable programming errors.
As an example consider the following signature:
class Resource; class Foo { public: Foo() : _resource(new Resource()) { } ~Foo() { delete _resource; } private: Resource* _resource; } Foo get_foo(); Foo foo = get_foo();
There is a bug in this function, it returns a temporary value which will be byte copied into foo then destroyed. Unfortunately, internaly the Foo class manage a pointer to a Resource object. This pointer will be released when the temporary is destroyed and foo will manage a pointer to an already released Resource.
Two issues has to be fixed in the example above:
- Function signature has to be changed to reflect the fact that Foo instances cannot be copied. In that case accessor should return a reference to give access to objects already existing and managed. Generator on the other hand should return a pointer to the created object.
// return a reference to an already managed Foo instance Foo& get_foo(); Foo& foo = get_foo(); // create a new Foo instance Foo* make_foo(); Foo* m = make_foo();
- Copy constructor and copy assignement operator has to be made private in the Foo class. It prevents unwanted copy of Foo objects. This can be done by declaring copy constructor and copy assignement in the private section of the Foo class.
class Foo { public: Foo() : _resource(new Resource()) { } ~Foo() { delete _resource; } private: // disallow copy operations Foo(const Foo&); Foo& operator=(const Foo&); // data members Resource* _resource; }
Another solution is to inherit privately from the NonCopyable class. It reduces the boiler plate needed to avoid copy operations but more importantly it clarifies the programer intent and the object semantic.
class Foo : private NonCopyable<Foo> { public: Foo() : _resource(new Resource()) { } ~Foo() { delete _resource; } private: Resource* _resource; }
- Template Parameters:
-
T The type that should be made non copyable. It prevent cases where the empty base optimization cannot be applied and therefore ensure that the cost of this semantic sugar is null.
As an example, the empty base optimization is prohibited if one of the empty base class is also a base type of the first non static data member:
struct A { }; struct B : A { int foo; }; // thanks to empty base optimization, sizeof(B) == sizeof(int) struct C : A { B b; }; // empty base optimization cannot be applied here because A from C and A from // B shall have a different address. In that case, with the alignement // sizeof(C) == 2* sizeof(int)
The solution to that problem is to templatize the empty class to makes it unique to the type it is applied to:
template<typename T> struct A<T> { }; struct B : A<B> { int foo; }; struct C : A<C> { B b; }; // empty base optimization can be applied B and C does not refer to the same // kind of A. sizeof(C) == sizeof(B) == sizeof(int).
Definition at line 141 of file NonCopyable.h.
Constructor & Destructor Documentation
NonCopyable | ( | ) | [protected] |
Disalow construction of NonCopyable objects from outside of its hierarchy.
Definition at line 146 of file NonCopyable.h.
~NonCopyable | ( | ) | [protected] |
Disalow destruction of NonCopyable objects from outside of its hierarchy.
Definition at line 150 of file NonCopyable.h.
Generated on Thu Jul 14 2022 19:34:41 by
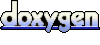