mbed library sources. Supersedes mbed-src. Fixed broken STM32F1xx RTC on rtc_api.c
Dependents: Nucleo_F103RB_RTC_battery_bkup_pwr_off_okay
Fork of mbed-dev by
DirHandle.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_DIRHANDLE_H 00017 #define MBED_DIRHANDLE_H 00018 00019 #include <stdint.h> 00020 #include "platform/platform.h" 00021 #include "platform/FileHandle.h" 00022 #include "platform/NonCopyable.h" 00023 00024 namespace mbed { 00025 /** \addtogroup platform */ 00026 00027 00028 /** Represents a directory stream. Objects of this type are returned 00029 * by an opendir function. The core functions are read and seek, 00030 * but only a subset needs to be provided. 00031 * 00032 * If a FileSystemLike class defines the opendir method, then the 00033 * directories of an object of that type can be accessed by 00034 * DIR *d = opendir("/example/directory") (or opendir("/example") 00035 * to open the root of the filesystem), and then using readdir(d) etc. 00036 * 00037 * The root directory is considered to contain all FileHandle and 00038 * FileSystem objects, so the DIR* returned by opendir("/") will 00039 * reflect this. 00040 * 00041 * @note to create a directory, @see Dir 00042 * @note Synchronization level: Set by subclass 00043 * @ingroup platform 00044 */ 00045 class DirHandle : private NonCopyable<DirHandle> { 00046 public: 00047 virtual ~DirHandle() {} 00048 00049 /** Read the next directory entry 00050 * 00051 * @param ent The directory entry to fill out 00052 * @return 1 on reading a filename, 0 at end of directory, negative error on failure 00053 */ 00054 virtual ssize_t read(struct dirent *ent) = 0; 00055 00056 /** Close a directory 00057 * 00058 * @return 0 on success, negative error code on failure 00059 */ 00060 virtual int close() = 0; 00061 00062 /** Set the current position of the directory 00063 * 00064 * @param offset Offset of the location to seek to, 00065 * must be a value returned from tell 00066 */ 00067 virtual void seek(off_t offset) = 0; 00068 00069 /** Get the current position of the directory 00070 * 00071 * @return Position of the directory that can be passed to rewind 00072 */ 00073 virtual off_t tell() = 0; 00074 00075 /** Rewind the current position to the beginning of the directory 00076 */ 00077 virtual void rewind() = 0; 00078 00079 /** Get the sizeof the directory 00080 * 00081 * @return Number of files in the directory 00082 */ 00083 virtual size_t size() 00084 { 00085 off_t off = tell(); 00086 size_t size = 0; 00087 struct dirent *ent = new struct dirent; 00088 00089 rewind(); 00090 while (read(ent) > 0) { 00091 size += 1; 00092 } 00093 seek(off); 00094 00095 delete ent; 00096 return size; 00097 } 00098 00099 /** Closes the directory. 00100 * 00101 * @returns 00102 * 0 on success, 00103 * -1 on error. 00104 */ 00105 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::close") 00106 virtual int closedir() { return close(); }; 00107 00108 /** Return the directory entry at the current position, and 00109 * advances the position to the next entry. 00110 * 00111 * @returns 00112 * A pointer to a dirent structure representing the 00113 * directory entry at the current position, or NULL on reaching 00114 * end of directory or error. 00115 */ 00116 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::read") 00117 virtual struct dirent *readdir() 00118 { 00119 static struct dirent ent; 00120 return (read(&ent) > 0) ? &ent : NULL; 00121 } 00122 00123 /** Resets the position to the beginning of the directory. 00124 */ 00125 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::rewind") 00126 virtual void rewinddir() { rewind(); } 00127 00128 /** Returns the current position of the DirHandle. 00129 * 00130 * @returns 00131 * the current position, 00132 * -1 on error. 00133 */ 00134 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::tell") 00135 virtual off_t telldir() { return tell(); } 00136 00137 /** Sets the position of the DirHandle. 00138 * 00139 * @param location The location to seek to. Must be a value returned by telldir. 00140 */ 00141 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::seek") 00142 virtual void seekdir(off_t location) { seek(location); } 00143 }; 00144 00145 00146 } // namespace mbed 00147 00148 #endif /* MBED_DIRHANDLE_H */
Generated on Thu Jul 14 2022 19:34:39 by
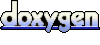