Pseudo real-time clock using Ticker interruption, also implements time() and set_time() for platforms that don't (such as mBuino)
Fork of PseudoRTC by
PseudoRTC Class Reference
Example: More...
#include <PseudoRTC.h>
Public Member Functions | |
PseudoRTC (void) | |
Create a pseudo real-time clock. | |
void | setTime (int y, int mo, int d, int h, int mi, int s) |
Set time in the pseudo real-time clock. | |
void | set_time (time_t thetime) |
Set the time using a unix timestamp value (the number of seconds since January 1, 1970) | |
time_t | time (time_t *timer) |
Get the unix timestamp value (the number of seconds since January 1, 1970) | |
time_t | addSeconds (int nSec) |
add (or subtract) some seconds to the rtc to adjust time as needed | |
int | getYear (void) |
Get the year value. | |
int | getMonth (void) |
Get the month value. | |
int | getDay (void) |
Get the day value. | |
int | getHour (void) |
Get the hour value. | |
int | getMinute (void) |
Get the minute value. | |
int | getSecond (void) |
Get the second value. |
Detailed Description
Example:
#include "mbed.h" #include "PseudoRTC.h" PseudoRTC c; main() { time_t seconds; char buffer[32]; c.set_time(1256729737); // Set RTC time to Wed, 28 Oct 2009 11:35:37 (NOW WORKING ON mBuino) seconds=c.time(NULL); printf("Time as seconds since January 1, 1970 = %d\r\n", seconds); printf("Time as a basic string = %s\r", ctime(&seconds)); // ctime includes \n strftime(buffer, 32, "%I:%M %p", localtime(&seconds)); printf("Time as a custom formatted string = %s\r\n", buffer); c.setTime(2014, 09, 20, 21, 05, 30); // Second example: September 20, 2014, 21:05:30 while(true) { printf("%04d/%02d/%02d %02d:%02d:%02d\r\m", c.getYear(), c.getMonth(), c.getDay(), c.getHour(), c.getMinute(), c.getSecond()); wait(1); } }
Definition at line 52 of file PseudoRTC.h.
Constructor & Destructor Documentation
PseudoRTC | ( | void | ) |
Create a pseudo real-time clock.
Definition at line 21 of file PseudoRTC.cpp.
Member Function Documentation
time_t addSeconds | ( | int | nSec ) |
add (or subtract) some seconds to the rtc to adjust time as needed
- Parameters:
-
nSec number of seconds to add or subtract
- Returns:
- time_t current timestamp
Definition at line 73 of file PseudoRTC.cpp.
int getDay | ( | void | ) |
Get the day value.
Definition at line 89 of file PseudoRTC.cpp.
int getHour | ( | void | ) |
Get the hour value.
Definition at line 94 of file PseudoRTC.cpp.
int getMinute | ( | void | ) |
Get the minute value.
Definition at line 99 of file PseudoRTC.cpp.
int getMonth | ( | void | ) |
Get the month value.
Definition at line 84 of file PseudoRTC.cpp.
int getSecond | ( | void | ) |
Get the second value.
Definition at line 104 of file PseudoRTC.cpp.
int getYear | ( | void | ) |
Get the year value.
Definition at line 79 of file PseudoRTC.cpp.
void set_time | ( | time_t | thetime ) |
Set the time using a unix timestamp value (the number of seconds since January 1, 1970)
- Parameters:
-
thetime unix time as time_t
Definition at line 51 of file PseudoRTC.cpp.
void setTime | ( | int | y, |
int | mo, | ||
int | d, | ||
int | h, | ||
int | mi, | ||
int | s | ||
) |
Set time in the pseudo real-time clock.
- Parameters:
-
y Year mo Month (1-12) d Day (1-31) h Hour (0-23) m Minute (0-59) s Second (0-59)
Definition at line 36 of file PseudoRTC.cpp.
time_t time | ( | time_t * | timer = NULL ) |
Get the unix timestamp value (the number of seconds since January 1, 1970)
- Parameters:
-
timer Pointer to the time_t variable
- Returns:
- time_t current timestamp
Definition at line 66 of file PseudoRTC.cpp.
Generated on Tue Jul 12 2022 20:45:19 by
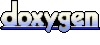