alpha a
Embed:
(wiki syntax)
Show/hide line numbers
motor.cpp
00001 /** A Motor Driver, used for setting the pins for PWM Outpu 00002 * for use with ROCO104 00003 * 00004 * @note Synchronization level: Interrupt safe 00005 * 00006 * Example: 00007 * @code 00008 * // Toggle a LED 00009 * #include "mbed.h" 00010 * 00011 * Motor Wheels(D15,D14,D13,D12); 00012 * 00013 * int main() 00014 * { 00015 * Wheel.Period_in_ms(2);//Set frequency of the PWMs 500Hz 00016 * while(true) 00017 * { 00018 * Wheel.Speed(0.8,0.8);//Forward 80% 00019 * wait(5.0); 00020 * Wheel.stop(); 00021 * wait(1.0); 00022 * Wheel.Speed(-0.8,-0.8);//Reverse 80% 00023 * wait(5.0); 00024 * Wheel.stop(); 00025 * wait(1.0); 00026 * } 00027 * } 00028 * @endcode 00029 */ 00030 00031 #include "motor.h" 00032 Motor::Motor(PinName pinName1, PinName pinName2, PinName pinName3, PinName pinName4) : pin1(pinName1), pin2(pinName2), pin3(pinName3), pin4(pinName4) 00033 { 00034 } 00035 void Motor::Fwd(float duty) 00036 { 00037 this->pin1 = 0.0f; 00038 this->pin2 = duty; 00039 this->pin3 = 0.0f; 00040 this->pin4 = duty; 00041 } 00042 void Motor::Rev(float duty) 00043 { 00044 this->pin1 = duty; 00045 this->pin2 = 0.0f; 00046 this->pin3 = duty; 00047 this->pin4 = 0.0f; 00048 } 00049 void Motor::Stop(void) 00050 { 00051 this->pin1 = 0.0f; 00052 this->pin2 = 0.0f; 00053 this->pin3 = 0.0f; 00054 this->pin4 = 0.0f; 00055 } 00056 int Motor::Speed(float speedA, float speedB) 00057 { 00058 if(speedA>1.0f||speedA<-1.0f){ //CHECK speedA Value is in Range! 00059 return -1; //return ERROR code -1=speedA Value out of range! EXIT Function 00060 } 00061 if(speedB>1.0f||speedA<-1.0f){ //CHECK speedB Value is in Range! 00062 return -2; //return ERROR code -2=speedB Value out of range! EXIT Function 00063 } 00064 00065 //If speed values have passed the checks above then the following code will be executed 00066 00067 if(speedA<0.0f) 00068 { //Reverse A motor 00069 this->pin1 = -speedA; 00070 this->pin2 = 0.0f; 00071 } 00072 00073 else 00074 { //Forward A motor 00075 this->pin1 = 0.0f; 00076 this->pin2 = speedA; 00077 } 00078 00079 if(speedB<0.0f) 00080 { //Reverse B motor 00081 this->pin3 = -speedB; 00082 this->pin4 = 0.0f; 00083 } 00084 else 00085 { //Forward B motor 00086 this->pin3 = 0.0f; 00087 this->pin4 = speedB; 00088 } 00089 return 0; //Return ERROR code Zero i.e. NO ERROR success! 00090 } 00091 00092 void Motor::Period_in_ms(int msPeriod) 00093 { 00094 this->pin1.period_ms(msPeriod); 00095 this->pin2.period_ms(msPeriod); 00096 this->pin3.period_ms(msPeriod); 00097 this->pin4.period_ms(msPeriod); 00098 }
Generated on Sun Jul 17 2022 02:59:28 by
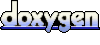