
IIR Sample Code
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "main.h" 00003 00004 #include "BP_4KHz_Fc_35KHz_Fs_5N.h" 00005 00006 //MBED Class Instances follows 00007 DigitalOut SampLED(LED1); //Digital Output (GREEN LED is PB_3, D13 You can use an Oscilloscope on This pin to confirm sample rate) 00008 00009 AnalogIn Ain(PA_1); //Analog Input (Signal Input 0 to +3 Volts) 00010 AnalogOut Aout(PA_4); //Analog Output (Signal Input 0 to +3 Volts) 00011 00012 Ticker sample_timer; 00013 00014 //Ticker routine PROTOTYPE to service samples from Analogue IP and port to Analogue Output 00015 void sampler(void); 00016 00017 int main() { 00018 00019 float sample_rate=(1.0/Fs)*1000000.0; //Calculate the number of uS required for a Frequency Sampling Rate 00020 //Fs held in init.h 00021 sample_timer.attach_us(&sampler,(int)sample_rate); 00022 //Ticker Instance serviced by routine at a repeat rate in microseconds 00023 00024 while(1) { 00025 sleep(); 00026 } 00027 } 00028 00029 //Ticker routine to service samples from Analogue IP and port to Analogue Output 00030 void sampler(void) 00031 { 00032 SampLED = ON; //LED Indicates start of sampling 00033 00034 xn=Ain; //Input ADC. N.B. ADC in MBED is 0.0 to 1.0 float!!!!!! 00035 00036 centreTap = xn*b0 + xnm1*b1 + xnm2*b2 + xnm3*b3 + xnm4*b4; //IIR Filter 00037 yn = centreTap*a0 - a1*ynm1 - a2*ynm2 - a3*ynm3 - a4*ynm4; //Result in yn 00038 00039 Aout=yn+0.5f; //Output resultant to DAC. Again MBED uses 0.0 to 1.0 float!!!!!! and Offset to give 0 to 3V3 range 00040 00041 //THESE NEED TO BE LOADED IN THIS ORDER OTHERWISE ALL xnm VALUES WILL BECOME THE SAME AS xn 00042 xnm4 = xnm3; 00043 xnm3 = xnm2; 00044 xnm2 = xnm1; 00045 xnm1 = xn; 00046 00047 //THESE NEED TO BE LOADED IN THIS ORDER OTHERWISE ALL ynm VALUES WILL BECOME THE SAME AS yn 00048 ynm4 = ynm3; 00049 ynm3 = ynm2; 00050 ynm2 = ynm1; 00051 ynm1 = yn; 00052 00053 SampLED = OFF; //LED Indicates end of sampling 00054 } 00055
Generated on Wed Jul 20 2022 13:57:55 by
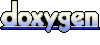